PHP Integration: Reusable HTML
PHP Integration: Reusable HTML
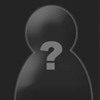
Everyone knows of the usefulness of PHP as a server-side language. However, one often overlooked aspect of PHP is that it can make rendering HTML a breeze. The major concepts at play here revolve around PHP included information, PHP variable / database information for HTML, and conditional / loop constructs assisting HTML.
PHP Includes
PHP includes generally have a two-fold purpose: un-cluttering the source in a web application page, and reusing useful source for multiple pages. When rendering HTML, the same is no different. Have a navigation bar that you use on all of your pages? Put the whole navbar in an included file, then just include it where the navbar would be. Example:
<div id=”main”>
<div style=”float:left;width:30%”>
<ul>
<li><a href=”first.php”>First Link</a></li>
<li><a href=”first.php”>Second Link</a></li>
<li><a href=”first.php”>Third Link</a></li>
</ul>
</div>
<div id=”content” style=”float:right;width:70%”>
My content is here.
</div>
</div>
Now, if we put the unordered list (starting at <ul> and ending at </ul>) in an include file by itself, we could include that file in every one of our pages. Thus, our code would become something like:
<div id=”main”>
<div style=”float:left;width:30%”>
<?php include ‘list.php’; ?>
</div>
<div id=”content” style=”float:right;width:70%”>
My content is here.
</div>
</div>
That example was a small space-saving and clarity example because the navbar was so simple and small. However, involved web applications will have more navigation items, as well as having more repeated / reused HTML throughout them. With common site design, it is likely that a page will have a header (logo and some information), navigation, and footer (copyright info and redundant nav, usually) in common between pages. However, we don’t have to limit it just to the logo and copyright info and such… since PHP interprets the pages before the HTML is rendered, we can effectively make pages that repeat the necessary HTML with includes. By “the necessary HTML”, I mean ALL of the HTML surrounding the content. Take this more complex example:
<?php $title = “Our Title”; include ‘header.php’; include ‘nav.php’; ?> <div id=“content” style=“float:right;width:70%”> My content is here. </div> <?php include ‘footer.php’; ?>
In ‘header.php’, we’ll have this opening HTML:
<html> <head> <title><?php echo $title; ?></title> </head> <body> <div id=“main”>
In ‘nav.php’, we’ll have the nav items’ HTML:
<div style=“float:left;width:30%”> <ul> <li><a href=“first.php”>First Link</a></li> <li><a href=“first.php”>Second Link</a></li> <li><a href=“first.php”>Third Link</a></li> </ul> </div>
For our ‘footer.php’, we’d just have some redundant or self-promotional information, so I will omit it for space’s sake. While the approach of using includes saves you time with repeating redundant information, there is an even more beneficial reason to use this approach: updates. If you wanted to change the site logo, add a few nav items, or even just update the copyright info at the bottom to reflect the current year... doing it in includes would only take *one* change in *one* place, while repeated HTML would leave you changing it on multiple pages.
PHP Conditionals / Loops Encasing HTML
Everyone is aware that PHP and HTML can co-exist in the same file, just as everyone is aware that PHP can output HTML using echo, print, or any variant. However, were you aware that it’s not necessary to “directly” output HTML using PHP when you want to take advantage of PHP’s loop and conditional constructs? This is a simple one, so I’m going to rush through it. Here are examples of the concept:
<?php if (isset($_GET[‘id’])) { ?> <div>Here’s a bunch of output!</div> <?php } else { ?> <div>No output for you!</div> <?php } ?>
When the PHP page is interpreted, the conditionals are read as normal by the interpreter… and whichever one is true will become the outputted HTML. Generally, you don’t want to bother with this when you have one or two lines of HTML to output… just echo’ing it to the page will be easier to read in that case. If there’s significantly more, just take that route. Same applies for loops as well:
<?php for ($x=0;$x<10;$x++) { ?> <p>I get printed 10 times!</p> <?php } ?>
PHP Shorthand
That last example (with the for loop) was the perfect time to launch into this topic. Generally, from PHP, you could use the “echo” or “print” statements to output info to the screen (especially PHP variables). However, there’s a shorter way if you don’t mind changing one setting in php.ini (short_open_tag to on | [url] http://mattsblog.ca/2007/07/26/tip-of-the-day-php-shorthand/[/url]):
<?=$myVariable?> That’s it. You have the opening <? for the shorthand method of opening PHP tags, = for the shorthand method of using “echo”, and the normal trailing ?>. What is so great about this? Oh… you can use it where you might not think of using PHP variables. Examples:
- <form action=“mypage.php?action=<?=$_POST[‘action’]?>&id=3”>
- <select><option value=“first”<?=$selected?>></select>
- <script>alert(’<?=ucwords($alertMsg)?>’);</script>
Single-Quoted Strings for HTML / Non-variables
To put the reasoning in a nutshell, single-quoted strings are interpreted faster than double-quoted strings. Why? Double-quoted strings could contain PHP variables; single-quoted ones will not. That’s good reasoning for not using double-quoted strings unless you really need variables in there but, mainly, I follow these two rules:
-
If I have to echo HTML (which uses lots of double quotes) or text without variables, I use single-quoted strings.
-
If I have to echo variables inside the text or echo a bunch of single quotes (Javascript, for instance), I use double quotes.
Most of it is the convenience of not having to escape every quote similar to what you started the string with. Example:
echo “<input id=\“myname\” type=\“text\” value=\“Zephyr\” />”; echo ‘<input id=“myname” type=“text” value=“Zephyr” />’;
DB Page Meat
… Huh? Alright, it may not be clear… but, “DB Page Meat” is referring to the wonderful concept of making the “meat” of your page (the main content) an entry in a database. How is this any easier than statically coding it with includes? It’s as simple as this:
<?php $sql = “SELECT title, content FROM myTable WHERE id=”.$_GET[‘id’]; $result = mysql_query($sql);
if ($result)
{
$row = mysql_fetch_array($result);
$title = $row[‘title’];
include ‘header.php’;
include ‘nav.php’;
echo $row[‘content’];
include ‘footer.php’;
}
?>
If you can make your site navigation use links that reference content in the database (by using GETs or POSTs with id numbers), you could realistically make that above page… your only page. Simplicity at its best.
Populate HTML w/ GET or POST as Action
So, we’ve already seen plenty of time-saving techniques using PHP to create usable and repeated HTML. Now, we’ll get down to the single-best use of these concepts: forms. Forms are generally composed of the same types of inputs when either adding new data or editing data of a particular type. For instance, we could have a form that asks you for your name, location, sex, and level of education. When editing your information, you’d be using a similar form. Why not use… the same form? To illustrate, we’ll capture the GET variable that we’d pass (called “action”), then act appropriately:
if (isset($_GET[‘action’])) { $sql = “SELECT name,location,sex,education FROM table WHERE id=”.$_GET[‘id’]; $result = mysql_query($sql);
if ($result)
{
$row = mysql_fetch_array($result);
}
}
<form action=“<?=$_SERVER[‘PHP_SELF’]?>“ method=“POST”> Name:<input type=“text” name=“myname” value=“<?=$row[‘name’]?>“ /> Location:<input type=“text” name=“mylocation” value=“<?=$row[‘location’]?>“ /> Sex:<input type=“radio” name=“sex” value=“male” <?php if (isset($row[‘sex’]) && $row[‘sex’] == ‘male’){ echo ’ checked=“checked”’; } ?> />Male<input type=“radio” name=“sex” value=“female” <?php if (isset($row[‘sex’]) && $row[‘sex’] == ‘female’){ echo ’ checked=“checked”’; } ?> /> <select name=“education”><option value=“none”>None</option> <?php $sql2 = “SELECT level FROM edutable ORDER BY id”; $result2 = mysql_query($sql2);
if ($result2)
{
while ($row2 = mysql_fetch_array($result2))
{
echo ‘<option value=”’.$row2[‘level’];
if ($row2[‘level’] == $row[‘education’])
{
echo ‘ selected’;
}
echo ‘>’.ucwords($row2[‘level’]).’</option>’;
}
}
?> </select> <input type=“submit” name=“mysubmit” value=“<?=ucwords($_GET[‘action’].’ Info’)?>“ /> </form>
Caught you off-guard with the select repetition up there, didn’t I? Well, that was a last-minute addition that I thought I’d just throw in. PHP and MySQL are great for creating large groups of options in a form… less manual work, easily-repeatable. That concept can be applied to select areas, groups of checkboxes… even whole groups of input areas if you have a number of them in succession. Use PHP and MySQL to the fullest when making your site efficient and easy to maintain.
I know everyone promises that there will be a follow-up article to many of the articles… but, I would like to assure you all that I have already started my second article on PHP. So, enjoy this one, leave some quality feedback, and we’ll see how the next one goes!
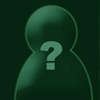
fashizzlepop 15 years ago
At first look it looks very good. I can't take the time to look in depth right now but great job. 2 good articles in a row! Woo hoo!
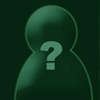
spyware 15 years ago
Poor Chinchilla3k 09 22 2008 - 05:46:08am. Anyway, good article. Voting awesome for content!
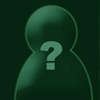
ghost 15 years ago
I might be wrong, but if you want to use the names with the $row['x'] stuff, you need to add the MYSQL_ASSOC constant to the statement, like this:
$row = mysql_fetch_array($result, MYSQL_ASSOC);
Very thorough article though, good job :happy:
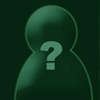
ghost 15 years ago
I initially thought that as well, jj… however, it seems that it's not necessary to specify that you're retrieving an associative array anymore. Both $row[0] and $row['x'] will work with mysql_fetch_array() without MYSQL_ASSOC. Thank you for the feedback, though. :)
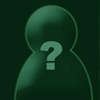
ghost 15 years ago
The concept of the hole article is wrong. In real production you will never see anything like this. Template is what you need to learn about. I would even go further and not recommend anyone to use this if you really want to learn something. There is some great technology out there that are 10 times better. Google about XSLT or Template in PHP.
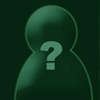
ghost 15 years ago
I beg to differ. The concept of master page templating in PHP uses concepts that are synonymous to the ones demonstrated in this article. In fact, the example that is composed almost entirely of includes and that uses DB access for filling content is at least as efficient as master pages; they simply use different methods to achieve the same objective. Also, I would love to hear about the statistical data that you are basing your claim of "you will never see anything like this in real production" off of; I work for a web design firm that also does work on sites that we did not originate and, in those cases, the concepts I illustrated are already present in various forms. Even PEAR templating does the same, though the templates are separated to a point of almost being too convoluted. Finally, mentioning XSLT as an alternative to doing what's illustrated in PHP is like recommending people to stop using .Net 2.0 with DB access and just use LINQ; advocate what you will, but there is nothing fundamentally wrong with the concepts I illustrated. Personal preference should not affect your review.
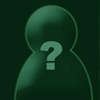
ghost 15 years ago
One last bit directed at Arto: If you wish to advocate XSLT for use in templating, then you should write your own article about it to demonstrate… as I have done advocating my methods of choice.
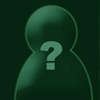
ghost 15 years ago
Your article had content people can learn from. The content can be summarized as (not replace the actual article mind you) as:
-Separation of static data into separate files for easy/centered manageability and producing the final product via php includes.
-Storage of static data into database for easy/centered manageability and producing the final product via SQL queries
-Information of arbitrary PHP internals and PHP tidbits.
Several things that bothered me is that the information is not so much trivial as it is common sense / very very un-esoteric. It's not that far of a stretch to come to this conclusion when one tries to solve the problem of redundant code. The code snippets you provide execute un-sanitized queries (on a hacking site.) The article is about as complex and intuitive as using vectors to have dynamically sized arrays in C++. It's the sort of thing the average developer should have no problem coming up with on their own.
That's not to say that posts you make on the forum have no influence on the way I judged the article. You usually are very critical about people's ideas and code (you are supportive though)… which is a good thing. You may have fulfilled the criteria you use to criticize other people but I am being critical of you in a similar way but using a different criteria. I'm not treating you as a newbie.. if yours31f came up with this article I probably would've rated it as 'very good'. I am treating you as another developer.. and I am telling you that the code is amateurish and the ideas you proposed is common-sense in practice.
I admit that I was a bit biased by your recent posts (not the SPDF thread.. what bothered me more was your post in the 2 cpu thread where you just replied "No.").. but I still probably wouldn't have rated it higher than Average.
Your article is better than most other HBH articles.. but that's not saying much considering the community. I'm also sure that your article will be able to help a few individuals in this community.
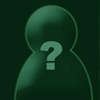
spyware 15 years ago
Chin, this isn't aimed at "other developers". This article is aimed at regular HBH folk. The common people. The stupid people. The people without common sense like Zephyr, or you. Those people can do with long, "common-sense" code. They GAIN something from this article. THEY, not YOU. Not ZEPHYR. Consider the flow of information; high ranks provide, low ranks consume. This article is useful, consumable and Awesome rateable. Enjoy your clouds of sophistication all you want, some people like Zephyr can actually float down to help some people.
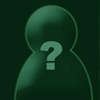
ghost 15 years ago
I do help.. I try not to flaunt the ways I help people. You can read most of my more recent posts.. they were to help people. You read in the spdf thread that I directly contacted the OP to help him. I had directly contacted yours31f and was patient enough to give him advice and help him with his code which lead to his marginally better forum etiquette recently. You lecturing me about helping people is baseless.
I finished my justification for the rating saying that it will probably help in this community. I acknowledged it. Why is it expected that I base the rating I give an article on how it could benefit the worst individual?
I understand that I usually appear to have a 'high and mighty' aura in my thread posts. They are usually in replies to people who act the same way to complete newbies. Zephyr helps.. I am not trying to be high and mighty here.. I am being critical.
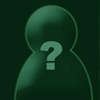
spyware 15 years ago
You are critical of this article's content. The content is (clearly) aimed at beginning/new php programmers. You're saying this article is too "common-sense" for developers.
Dent. I think there's a dent in this logic. Dentdentdent.
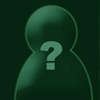
ghost 15 years ago
Chin, your feedback is very complete and focused, for which I am most grateful. My goal in encouraging feedback is to receive that, whether good or bad. There are a few specific points I'd like to address, however: (1) The target audience of this article can be seen in the article's summary; this should be noted by every reviewer of this article from here on out. My following articles will progress more and more to polished code but, in order to reach that, common convention must become… common. A number of PHP newbies here are in need of the transitional steps. (2) Sanitizing the queries is something that would be largely done from the header include, since the variables should be handled before they are "touched by code". This is also a concept that I plan to write an article on but, in the meantime, I am omitting it both for space considerations and for content focus. (3) Your opinion of me and my forum posts are good to know, but ultimately irrelevant. I'm glad that they did not factor into your review of this article. Your reasoning for voting and reviewing as you did has its merits and carries more weight than some reviews. Thank you.
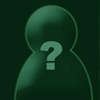
reaper4334 15 years ago
Great article. In depth and clear, yet has good complexity, enough to describe things properly rather than just briefly mentioning everything. Good job :)
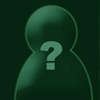
ghost 15 years ago
Writing an article … it's like 4 lines of code to use XSLT in PHP and for XSL there is plenty of article on the web about that. And why it's not being used in real production. It's simple, if you want to put some php code in that, after 1 month of production it's gonna get so messy that's almost impossible to continue to code or debug a problem. I've seen website coded in 2 page like that and it's an horror. Also, you can't apply the principle of "coding in layer" with that. Output and php code is all mixed up together and if you want to do a mess with your code that's how you do it. Also just a quick example to demonstrate up to what point it can be a bad way of coding : Imagine if you builded up a site like Amazon and you wanted to change the design of the site. How are you gonna do that ? The design is mixed up with the code and it's gonna take you hour and day to do something it would have took you few minutes to do if you had used template and you had separate PHP code from HTML code. Also, it's not because you work in a firm that you necesseraly code the better way. It's a way of coding what you propose, but I totally disagree that's it's a good one. If you want to do a clean job, you have to learn to separate PHP code from HTML code.
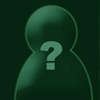
ghost 15 years ago
So, in other words… you suggest XSLT, but you're not willing to write an article to counter what I've written? Okay. It's not being used in real production, but you're basing that on… what? If you re-read my article, you'll find that the PHP code is not "mixed in" with the HTML code. It augments the HTML, hence the title of my article. If you want to change the design of a site, the backbone of your site will be in includes, as shown above. Templates would be handled the same way… PHP output is HTML and, if you change the layout, it's likely that you have to change the output from PHP functions and procedural-style code. PHP templating doesn't change this. I never at any point said that I "code the better way"; you're turning this into a pissing contest instead of a trade of ideas. You're complaining that my way is not the most efficient, and yet you have neglected to notice the focus audience that I specified in the article summary. Basically, you're just crapping up my comment area with your unjustified, opinionated, and reference-lacking shit… Is that about it? If you want an argument, go somewhere else. I wrote an article for people that are not expert PHP coders to enjoy and learn from and, if you don't like it, shut the fuck up.
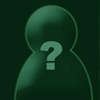
ghost 15 years ago
I wrote this article based on a perceived need. A number of people at this site seek to learn PHP, only to find it difficult to grasp form-based and session-based sites that use PHP. This article was intended to provide that information in an easy-to-digest manner. The code in the article is intentionally sub-par because I made it easier to read; I literally had to write the code the way I would normally, then go back and "dumb it down". I didn't write this article to display expert coding techniques (obviously) and the summary reflects EXACTLY THAT. I took a simple concept and embellished upon it with some more advanced techniques to make it a full-bodied article. If you're going to criticize it, criticize with justification based upon the original topic AND the implied level of proficiency from the summary. Otherwise, you're just trying to blow smoke up your own ass… which doesn't need to happen in my comment area. If that's your goal, then just PM me and I'll listen to your shit all day long. The reason why we don't have more quality articles is because there's no incentive to write any with people on their high horses bashing thorough and detailed articles that are useful to a certain group of people. None of you started as "elite" (in fact, very few of you actually are), and it was articles like this, the articles that esteemed members have written, and the forum posts that members who actually give a shit enough to help made… that got you there.
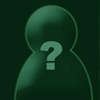
ghost 15 years ago
First of all it is mixed. You have "PHP Code, HTML, PHP Code, HTML, PHP Code, etc." Not mixed content is "Beginning of file, PHP Code, Ending of file". Second I'm gonna stop arguing with you because you first of seems to miss some concept in programming that are the center of my point of view. I would strongly suggest you to read about this http://en.wikipedia.org/wiki/Multilayered_architecture and that http://en.wikipedia.org/wiki/Model-view-controller
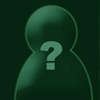
ghost 15 years ago
I have sent you a PM… Apparently, you don't have enough respect for my words to note that I requested at least that much. This argument won't continue here. If you're that desperate to win… You just did. Congrats.
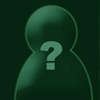
ghost 15 years ago
Inbox was full didn't received the message, resend it. Respect ? I'm arguing about your idea not you, it's perfectly normal to don't have same opinion on an idea … I'm not saying your an idiot.
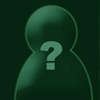
ghost 15 years ago
zephyr!!! Nice content. Proper syntax, closing the php tags where you should. Using ul / ol for lists. Nice. Just, style code in your main documents is ugly. But, i guess you coulnd't shot that :P The one sad thing is, that this basic n00b shit has to be tought on a site that supposed to consist of hackers! If you don't even know this, i think you have no buisness here, but at w3c , or php.net. Or school!
Nice article though, i wish more people knew how to teach in a proper manner. :happy: peace.