PHP Integration: Reusable HTML
PHP Integration: Reusable HTML
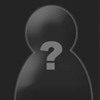
Everyone knows of the usefulness of PHP as a server-side language. However, one often overlooked aspect of PHP is that it can make rendering HTML a breeze. The major concepts at play here revolve around PHP included information, PHP variable / database information for HTML, and conditional / loop constructs assisting HTML.
PHP Includes
PHP includes generally have a two-fold purpose: un-cluttering the source in a web application page, and reusing useful source for multiple pages. When rendering HTML, the same is no different. Have a navigation bar that you use on all of your pages? Put the whole navbar in an included file, then just include it where the navbar would be. Example:
<div id=”main”>
<div style=”float:left;width:30%”>
<ul>
<li><a href=”first.php”>First Link</a></li>
<li><a href=”first.php”>Second Link</a></li>
<li><a href=”first.php”>Third Link</a></li>
</ul>
</div>
<div id=”content” style=”float:right;width:70%”>
My content is here.
</div>
</div>
Now, if we put the unordered list (starting at <ul> and ending at </ul>) in an include file by itself, we could include that file in every one of our pages. Thus, our code would become something like:
<div id=”main”>
<div style=”float:left;width:30%”>
<?php include ‘list.php’; ?>
</div>
<div id=”content” style=”float:right;width:70%”>
My content is here.
</div>
</div>
That example was a small space-saving and clarity example because the navbar was so simple and small. However, involved web applications will have more navigation items, as well as having more repeated / reused HTML throughout them. With common site design, it is likely that a page will have a header (logo and some information), navigation, and footer (copyright info and redundant nav, usually) in common between pages. However, we don’t have to limit it just to the logo and copyright info and such… since PHP interprets the pages before the HTML is rendered, we can effectively make pages that repeat the necessary HTML with includes. By “the necessary HTML”, I mean ALL of the HTML surrounding the content. Take this more complex example:
<?php $title = “Our Title”; include ‘header.php’; include ‘nav.php’; ?> <div id=“content” style=“float:right;width:70%”> My content is here. </div> <?php include ‘footer.php’; ?>
In ‘header.php’, we’ll have this opening HTML:
<html> <head> <title><?php echo $title; ?></title> </head> <body> <div id=“main”>
In ‘nav.php’, we’ll have the nav items’ HTML:
<div style=“float:left;width:30%”> <ul> <li><a href=“first.php”>First Link</a></li> <li><a href=“first.php”>Second Link</a></li> <li><a href=“first.php”>Third Link</a></li> </ul> </div>
For our ‘footer.php’, we’d just have some redundant or self-promotional information, so I will omit it for space’s sake. While the approach of using includes saves you time with repeating redundant information, there is an even more beneficial reason to use this approach: updates. If you wanted to change the site logo, add a few nav items, or even just update the copyright info at the bottom to reflect the current year... doing it in includes would only take *one* change in *one* place, while repeated HTML would leave you changing it on multiple pages.
PHP Conditionals / Loops Encasing HTML
Everyone is aware that PHP and HTML can co-exist in the same file, just as everyone is aware that PHP can output HTML using echo, print, or any variant. However, were you aware that it’s not necessary to “directly” output HTML using PHP when you want to take advantage of PHP’s loop and conditional constructs? This is a simple one, so I’m going to rush through it. Here are examples of the concept:
<?php if (isset($_GET[‘id’])) { ?> <div>Here’s a bunch of output!</div> <?php } else { ?> <div>No output for you!</div> <?php } ?>
When the PHP page is interpreted, the conditionals are read as normal by the interpreter… and whichever one is true will become the outputted HTML. Generally, you don’t want to bother with this when you have one or two lines of HTML to output… just echo’ing it to the page will be easier to read in that case. If there’s significantly more, just take that route. Same applies for loops as well:
<?php for ($x=0;$x<10;$x++) { ?> <p>I get printed 10 times!</p> <?php } ?>
PHP Shorthand
That last example (with the for loop) was the perfect time to launch into this topic. Generally, from PHP, you could use the “echo” or “print” statements to output info to the screen (especially PHP variables). However, there’s a shorter way if you don’t mind changing one setting in php.ini (short_open_tag to on | [url] http://mattsblog.ca/2007/07/26/tip-of-the-day-php-shorthand/[/url]):
<?=$myVariable?> That’s it. You have the opening <? for the shorthand method of opening PHP tags, = for the shorthand method of using “echo”, and the normal trailing ?>. What is so great about this? Oh… you can use it where you might not think of using PHP variables. Examples:
- <form action=“mypage.php?action=<?=$_POST[‘action’]?>&id=3”>
- <select><option value=“first”<?=$selected?>></select>
- <script>alert(’<?=ucwords($alertMsg)?>’);</script>
Single-Quoted Strings for HTML / Non-variables
To put the reasoning in a nutshell, single-quoted strings are interpreted faster than double-quoted strings. Why? Double-quoted strings could contain PHP variables; single-quoted ones will not. That’s good reasoning for not using double-quoted strings unless you really need variables in there but, mainly, I follow these two rules:
-
If I have to echo HTML (which uses lots of double quotes) or text without variables, I use single-quoted strings.
-
If I have to echo variables inside the text or echo a bunch of single quotes (Javascript, for instance), I use double quotes.
Most of it is the convenience of not having to escape every quote similar to what you started the string with. Example:
echo “<input id=\“myname\” type=\“text\” value=\“Zephyr\” />”; echo ‘<input id=“myname” type=“text” value=“Zephyr” />’;
DB Page Meat
… Huh? Alright, it may not be clear… but, “DB Page Meat” is referring to the wonderful concept of making the “meat” of your page (the main content) an entry in a database. How is this any easier than statically coding it with includes? It’s as simple as this:
<?php $sql = “SELECT title, content FROM myTable WHERE id=”.$_GET[‘id’]; $result = mysql_query($sql);
if ($result)
{
$row = mysql_fetch_array($result);
$title = $row[‘title’];
include ‘header.php’;
include ‘nav.php’;
echo $row[‘content’];
include ‘footer.php’;
}
?>
If you can make your site navigation use links that reference content in the database (by using GETs or POSTs with id numbers), you could realistically make that above page… your only page. Simplicity at its best.
Populate HTML w/ GET or POST as Action
So, we’ve already seen plenty of time-saving techniques using PHP to create usable and repeated HTML. Now, we’ll get down to the single-best use of these concepts: forms. Forms are generally composed of the same types of inputs when either adding new data or editing data of a particular type. For instance, we could have a form that asks you for your name, location, sex, and level of education. When editing your information, you’d be using a similar form. Why not use… the same form? To illustrate, we’ll capture the GET variable that we’d pass (called “action”), then act appropriately:
if (isset($_GET[‘action’])) { $sql = “SELECT name,location,sex,education FROM table WHERE id=”.$_GET[‘id’]; $result = mysql_query($sql);
if ($result)
{
$row = mysql_fetch_array($result);
}
}
<form action=“<?=$_SERVER[‘PHP_SELF’]?>“ method=“POST”> Name:<input type=“text” name=“myname” value=“<?=$row[‘name’]?>“ /> Location:<input type=“text” name=“mylocation” value=“<?=$row[‘location’]?>“ /> Sex:<input type=“radio” name=“sex” value=“male” <?php if (isset($row[‘sex’]) && $row[‘sex’] == ‘male’){ echo ’ checked=“checked”’; } ?> />Male<input type=“radio” name=“sex” value=“female” <?php if (isset($row[‘sex’]) && $row[‘sex’] == ‘female’){ echo ’ checked=“checked”’; } ?> /> <select name=“education”><option value=“none”>None</option> <?php $sql2 = “SELECT level FROM edutable ORDER BY id”; $result2 = mysql_query($sql2);
if ($result2)
{
while ($row2 = mysql_fetch_array($result2))
{
echo ‘<option value=”’.$row2[‘level’];
if ($row2[‘level’] == $row[‘education’])
{
echo ‘ selected’;
}
echo ‘>’.ucwords($row2[‘level’]).’</option>’;
}
}
?> </select> <input type=“submit” name=“mysubmit” value=“<?=ucwords($_GET[‘action’].’ Info’)?>“ /> </form>
Caught you off-guard with the select repetition up there, didn’t I? Well, that was a last-minute addition that I thought I’d just throw in. PHP and MySQL are great for creating large groups of options in a form… less manual work, easily-repeatable. That concept can be applied to select areas, groups of checkboxes… even whole groups of input areas if you have a number of them in succession. Use PHP and MySQL to the fullest when making your site efficient and easy to maintain.
I know everyone promises that there will be a follow-up article to many of the articles… but, I would like to assure you all that I have already started my second article on PHP. So, enjoy this one, leave some quality feedback, and we’ll see how the next one goes!
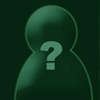
ghost 16 years ago
and at arto…you should really stfu. Write an article, thou 1337ness, then we'll see how laughable that is! I can code your ass under table with php in like 5 minutes with my eyes closed, dumbshit. So stfu, and "getta writing" :vamp: This is a proper, understandable article, which fits beautifully in this community.
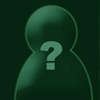
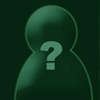
korg 16 years ago
I liked this one, Good content, Well structured, And good grammar. Although I don't agree with some of the functions, It's a good start for people. 8/10