Using Templates in C++
Using Templates in C++
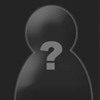
This is a short tutorial on C++ templates.
Readers: People with a little knowledge about C++
DISCLAIMER: Even though it is an article written by me, I have used information from various sites as reference. So I cannot claim it as completely my own article. Also, please forgive any mistakes that I make.
Lets start then.
How many times have you coded a function and thought if it could have worked on different data types. Or you write a class and wished to implement the same one for various data types.
A very simple example would be a function add.
int add(int a,int b)
{
return(a+b);
}
Wouldn't it be nice if we can use the same function to add integers, long and floating point values? Here is where templates come in to play.
TEMPLATES:
Basically, templates make your functions or classes more abstract, so that you can implement a single class//function for different data types.
We can now just classify our templates in to function templates and class templates.
We will deal with function templates first.
FUNCTION TEMPLATES
Function templates allow a function to work on different data types. This can be achieved using template parameters. A template parameter is a special kind of parameter that can be used to pass a type as argument: just like regular function parameters can be used to pass values to a function, template parameters allow to pass also types to a function. These function templates can use these parameters as if they were any other regular type.
The syntax for this is:
template <class identifier> function_declaration;
template <typename identifier> function_declaration;
To call the function with a specific data type, we use:
function_name <datatype> (arguments);
We will see an example to understand more.
template <class T>
T add (T a, T b) {
return (a+b);
}
Above, we have created a template function with template parameter T. Now, this parameter T represents a type that has not been defined. We can later define this parameter to be a data type of our liking.
Here is the function call in the main() part:
int x=2,y=3;
float a=10.3,b=6.2;
int integerSum = add <int> (x,y);
float realSum = add <float> (a,b);
cout<<"Integer Sum = "<<integerSum;
cout<<"nFloat Sum = "<<realSum;
The output will be
Integer Sum = 5
Float Sum = 16.5
We can now analyze how we did it. In the main body, we first declare our variables, x and y which are integers and a,b which are float.
Next, there is an integer variable integerSum which accepts the result from add() function. See how we told the function that we are using integers. We put the data type of parameters as <data type> between the function name and the arguments. So, now the compiler knows that we are sending integer values to the function. So it return an integer after adding the two numbers. Same goes for the floating point values.
Also, it is not necessary to define the data type of the elements. The compiler will automatically check the type. So you can reduce the function call as:
int a=2,b=3,sum=0;
sum=add(a,b);
cout<<sum;
What if we want to send two different types. Then, change the declaration a bit to accept two different types. That is:
template <class T,class U>
T add (T a, U b) {
return (a+b);
}
And the main body:
float a=2.35,result;
int b=5;
result=add(a,b); ////If you want, you can use result=add <float,int>(a,b);
So, that will be all about function templates for now. We can now move on to class templates.
CLASS TEMPLATES:
Class templates are same as function templates except that they are used to work on classes. Class templates are very useful when working with data structures like Stacks, trees, graphs, … etc. Here the members can use template parameter as type.
In the below syntax, identifier refers to the name you give to the type The syntax for class declaration is:
template <class identifier> class classname {....};
Eg:
template <class T> class add_class {.....};
To define a member function of class:
template <class identifier> identifier classname <identifier> :: function_name() {.....}
Eg:
template <class T> T add_class <T> :: sum() {....}
To create an object of class with a specific data type:
classname <datatype> object;
Eg:
add_class <int> obj1;
We can use the same example of addition as used in function templates. Here, we create a class add:
template <class T>
class add {
T a,b;
public:
T add(T first,T second)
{ a=first; b=second; }
T sum()
{ return(a+b); }
};
Above, we use class add. It has two elements a and b which are of the template parameter type. We have to define it later. There is a constructor which assigns values to a and b (since a and b are private, we have to use this constructor). Also there is a member function sum() which returns the sum of a and b.
Now let us look in to the code in the main function that actually adds two integers as well as two floats.
add <int> obj1(2,3);
add <float> obj2(10.3,6.2);
int res1 = obj1.sum();
float res2 = obj2.sum();
cout<<"Integer sum = "<<res1;
cout<<"nFloat sum = "<<res2;
In the above code, we create two objects, obj1 and obj2. obj1 is of integer type and obj2 is float. That is, the elements a and b of obj1 is integer and that of obj2 is float. We initialize a and b with values during object creation itself, using constructors. The rest of the code is simple and you will understand it if you have been reading this article till now.
There is more on templates to talk about, but right now, I am a bit tired. So, we will conclude our article here. There is more to talk about on templates but we will look in to it later. Templates make programming generic and so makes life easier. There is the Standard Template Library in C++ which provides many predefined types like stacks, queues, …etc
[Here is a small paragraph from cprogramming.com on STL ]
One of the later editions to the C++ standard is the Standard Template Library (STL). The STL is a set of abstract datatypes, functions, and algorithms designed to handle user-specified datatypes. Each of the abstract datatypes also contains useful functions, including overloaded operators, to access them. The spirit of the Standard Template Library is the idea of generic programming - the implementation of algorithms or data structures without being dependent on the type of data being handled. For instance, you can use the STL vector container to store a vector (think of it as a resizable array) of any object you desire. In C and non-STL C++, you can use arrays to get a similar feature, but arrays are limited to a single type of data structure. Moreover, the STL provides some nice features such as handling memory for you (no memory leaks), and it is also safer (no buffer overflow issues when using vectors or similar data structures).
Hope you guys liked this tutorial. [DMWM]
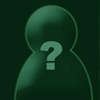
ghost 15 years ago
Way too much text to describe something easy and simple, not the best use of English, awful way to end it, no mention of how it works underneath, nothing about function overloading in association with templates, etc. Rating average though.
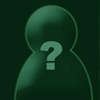
ghost 15 years ago
In the defense of the article's author, I think this is about as short as you can make it for most people to understand it. Agree about the use of English; just make another run through articles after you write them… just takes a quick skim to catch "memeber" and such. Agree about the end, too; way too abrupt and just plain ugly. The article is an average article, but it had a good chance to be a great one; I like that the detail (on what you did explain) is just enough to reiterate the concept without beating it to death. Also, I've heard COM's explanation of this topic before (which was exemplary), so you should certainly pay a great deal of attention to his feedback.
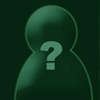
korg 15 years ago
Decent simple article, not that bad. Tightened up the grammar and spelling mistakes for him also, rated good.