Multi-Threads in Java
Multi-Threads in Java
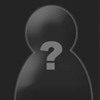
Threads: A difficult task (sometimes to implement but (for sure) to debug) that cannot be implemented by all programming languages.
A thread is an autonomous process that runs in memory in order to perform a specific task. The threads usually created by a program.
While a tread is created, it performs its task in "parallel" with its creator (the program). Well, the word "parallel" basically depends on two main things:
- The Operating System (multi tasking capabilities).
- The processor (Multi threading technology, Dual Core, multi processor system etc). But, anyway, I will not go too far with the OS & hardware issues.
A program can create more than one thread: In such case we have a multithread situation.
Java is one of very few languages (for my opinion) that allow a relatively easy way of creating and processing threads. There are two basic methods to do this:
1.The easy but not always a productive way: Inherited from build-in class Tread.
2.The usual way: By executing a runnable interface. An interface is the Java method to handle multiple inheritance.
A very simple example that shows how to use threads is the following: Suppose that we have to count from 0 to… 11..!! yes… yes… don’t "lol" you lamer !!…. the example is for educational purposes. But, of course, you can extend it to handle more… complex situations.
Well,… instead of making a for loop to count from 0 to 11 we can apply the "divide and conquer" method: Creating 3 threads: Thread One will count from 0 - 3. Thread Two will count from 4 - 7. Thread Three will count from 8 - 11. Well again…. i am just thinking… if a brute force apps could be "broken down" in many such steps…. ;) ….
I provide the source code of the above example… by using both methods. I suggest you to use my code for practice. In addition, please extend my code to handle more…. "complex" situations… ;)
Note: See how the threads are asynchronously executed once they are started.
/*--------------------------------------------------------------------------------------------------*/
/*
* (c) Thiseas 01/10/06
* Multi threads in Java
* 1st way: extending the Thread class.
*/
public class myThreads
{
public static void main(String [] args)
{
System.out.println(\"Begin main Function\");
mThread Thread_One = new mThread (\"One\",0,3);
mThread Thread_Two = new mThread (\"Two\",4,7);
mThread Thread_Three = new mThread(\"Three\",8,11);
Thread_One.start();
Thread_Two.start();
Thread_Three.start();
System.out.println(\"End main Function\");
}// main
}//myThreads
class mThread extends Thread
{
private String ThreadName;
private int StartFrom;
private int FinishTo;
public mThread(String name, int n1, int n2)
{
ThreadName = name;
StartFrom = n1;
FinishTo = n2;
}
public void run(){
for (int i=StartFrom; i<=FinishTo; i++)
System.out.println(ThreadName+ \"Counter:\" +i);
}
}//mThread
/*--------------------------------------------------------------------------------------------------*/
</code>
2nd way
[code]
/*--------------------------------------------------------------------------------------------------*/
/*
* (c) Thiseas 01/10/06
* Multi threads in Java
* 2nd way: Implements a Runnable Interface
*/
public class myThreads2
{
public static void main(String [] args)
{
System.out.println(\"Begin main Function\");
mThread Thread_One = new mThread (\"One\",0,3);
mThread Thread_Two = new mThread (\"Two\",4,7);
mThread Thread_Three = new mThread(\"Three\",8,11);
System.out.println(\"End main Function\");
}// main
}//myThreads2
class mThread implements Runnable
{
private String ThreadName;
private int StartFrom;
private int FinishTo;
public mThread(String name, int n1, int n2)
{
ThreadName = name;
StartFrom = n1;
FinishTo = n2;
Thread t = new Thread(this);
t.start();
}
public void run(){
for (int i=StartFrom; i<=FinishTo; i++)
System.out.println(ThreadName+ \"Counter:\" +i);
}
}//mThread
/*--------------------------------------------------------------------------------------------------*/
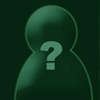
ghost 18 years ago
Not a bad article, but that's a hell of a lot of code to print the numbers 1-11… I'd just stick with the old-fashioned 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11 method.
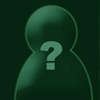
ghost 18 years ago
CrazyCaity123….. when someone pointing to the moon…. do you look his finger? :( :(
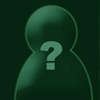
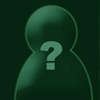
ghost 18 years ago
No, I scream and run into my house because the moon, like many other things in the world, is out to get me… Did you know that there's ghosts on the moon that eat your soul if you stare at it for too long?