Programming in Pascal
Programming in Pascal
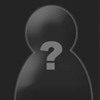
Im taking intro to programming in my school this year and we are learning pascal. we arnt that far into it but i can give some basic commands and explain how to make a basic program. the program i use to write pascal is "kate editor". then to run the pascal program i use "konsole". i havent tried pascal with anything else so i dont know what else it would work with.
Ok to start off ill give you a list of commands.
(the commands will be in caps but you dont need them in caps while writing the program)
PROGRAM this indicates that the code is a program (this should be the first word of your program)
VAR this begins the section where you declare your variables for the program.
(example)
var
number,
number_2,
answer:real;
(if you want a space in your variables you need an underscore. also at the end of a line of code you add a ; so the compiler knows its the end. but if you want to continue lines add a , so the compiler knows they are seperate but still together. also you need to declare what type of variable it is. that is why you do :real; that indicates that variable and the variables above it are real numbers. other ones to use are :integer; for whole numbers :char; for a single letters :string; to store words in a string.)
BEGIN this starts the program ( put this after the VAR section)
to add comments anywhere use { }
WRITELN this prints out what you want onto the screen.
(example)
writeln('Hello World!');
( when you want just words to appear use ' ' and for writeln statements what you want written you write in ( ) )
(another example)
writeln('The answer ', answer);
(this would be used when you want to print out a variable. so if your answer was 35 it would print The answer 35)
READLN this reads the line typed and stores it in a var
(example)
writeln('Enter the number please');
readln(number);
( the word in the parentheses on the readln line is the var declared earlier in the var section. the number that you type after the compiler prints the words 'Enter the number please' will be stored as the variable 'number')
when you want to have calculations in your program do it like this
answer := number * number_2; (that is a basic calculation. you need spaces inbetween each thing. and for equals you need := )
END. end. indicates that your program has ended. (before the end. the line right before the line with end. on it you cant have a ; at the end of that line)
now ill give you a basic program as an example
program compute_volume { this program will compute the volume of a cylinder } var height, {the height of the cylinder } radius, {the radius of the base } pie, {the number pie } volume:real; {the volume of the cylinder}
begin { main program }
{ input }
writeln('Enter the height of the cylinder.');
readln(height);
writeln('Enter the radius of the base.');
readln(radius);
{ calculations }
pie := 3.14; volume := radius * radius * height * pie;
{ output }
writeln('The height ', height);
writeln('The radius ', radius);
writeln('The volume ', volume);
writeln
end. { main program }
that was a basic program that computes the volume of a cylinder.
now ill go through compiling and running the program
first using "kate editor" copy and paste the program i showed. create a folder called test. save the program as test.pas .pas indicates its a pascal program. open konsole and type cd test this opens the folder 'test'. now to compile the program type ppc386 test.pas this command might be different for different konsole versions but im not sure. so if it doesnt work then look it up. now when your compiling it there may be errors. i cant list all of them so if you get an error and dont know how to fix it then google it. after it has succesfully compiled then type ./test to run it.
if you have any questions about this then you can email me at keton06@mail.com i hope this article helps you understand pascal a little. ill have another article on pascal soon. please rate if you liked.
-keton
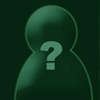
ghost 18 years ago
ok. why would a school teach pascal?? what is wrong with them! u should drop that course and change to OOP course. believe me, i know pascal and i know java and you cant even compare!!
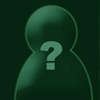
ghost 18 years ago
i would but i switched into this class right before the switching period was over and now i cant switch out.
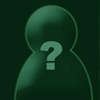
What_A_Legend 18 years ago
lol i submitted a pascal program in to the prog comp lol nice lil programing calling procedures and using loos and what not, nice easy program.
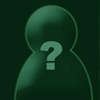
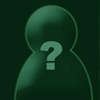
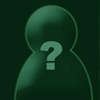
richohealey 18 years ago
but…. pascal is for quiche eaters!
lol, nice article, but i don't approve of the language, slow irratating and inefficient.
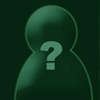
ghost 17 years ago
Pascal is one of my favorite languages, but your article was just a decent introduction. If you had mentioned functions and thrown in a reasonably comprehensive list of data types, then I would've rated this article higher… as it is, it's not a complete intro.