PySerial and serial devices
PySerial and serial devices
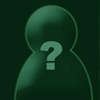
Python serial communication.
This article is for those who want to learn about how python can communicate with serial data. This could be handy for many things; Bluetooth communication via COM ports or even USB to Serial communication.
I use PySerial to communicate with my Arduino and my BasicStamp. PySerial supports two way communications with file handles. You can read and write strait to serial. Since python’s interpreted, fast testing, debugging, and developing can be done to improve proto-to-production time.
You could use Pyserial to: *send servo posistions *physical email notification *robotics and Wii motes
I am using it to read accelerometer data, along with analog piezo data. Reading a value is simple.
s = serial.Serial(\'COM8\') #for Linux use something line serial.Serial(\'/dev/ttyS1\')
s.read()
..
..
>>\'5\'
Assuming the port had data to read. It is a good idea to set a timeout for ports, because you might wait forever. It is also a good idea to set a baud rate too, so you don't get any sync errors or bugs. To do this, simple specify, and you shall receive.
s = serial.Serial(\'COM8\', baudrate=9600, timeout=3)
You can also use readline to gather larger amounts of data. You are required to specify a timeout on readline and readlines, else it will give you tracebacks.
After doing a readline, you can manipulate the data better.
s = serial.Serial(\'COM8\',timeout=1)
data = s.readline()
data = data.split()
print data
[\'x\',\'=\',\'5028\',\'y\',\'=\',\'2507\']
Assuming the serial device is sending data, this was grabbed from an accelerometer.
As you have noticed, the data is in string format. The easiest way to get around this is to use int(). Now you can use values in conditional statements, math calculations, and graphs. Coupled with Pygame, Tkinter, or excel has limitless potential (well almost limitless).
s = serial.Serial(\'COM8\', baudrate = 9600, timeout=1)
while 1:
d = s.readline()
d = d.split()
if int(d[2]) > 2700:
print \"X value beyond threshold.\"
s.write(\"x\")
if int(d[5]) > 2850:
print \"Y value beyond threshold.\"
s.write(\"y\")
Assuming the device reads the value we have written to serial, a LED would light up. I used the Arduino Duemilanove. The Arduino platforms programming language is based on Processing. Processing itself is based on C/C++, so programming isn't difficult.
The biggest problem I ran into while working with PySerial, doesn't have to do with the module, it was on the Arduino. To do a readline the arduino had to send a newline character so I could split the data.
This is the Processing code on the Arduino for the examples I've used:
/*
Quick and dirty program for led output.
Intended for example not actual code.
*/
const int xPin = 2; // X output of the accelerometer
const int yPin = 3; // Y output of the accelerometer
const int xLed = 4; // X LED
const int yLed = 5; // Y LED
void setup() {
// initialize serial communications:
Serial.begin(9600);
// initialize the digital pins
pinMode(xPin, INPUT);
pinMode(yPin, INPUT);
pinMode(xLed, OUTPUT);
pinMode(yLed, OUTPUT);
}
void loop() {
// variables to read the pulse widths:
int pulseX, pulseY;
char sd
// gather the data
pulseX = pulseIn(xPin,HIGH);
pulseY = pulseIn(yPin,HIGH);
// write to serial so python can read it
Serial.print(\"X = \");
Serial.print(pulseX);
Serial.print(\" Y = \"); //notice the space before the Y. This is to keep Y off of X\'s value
//Else it would give a value error for int() function in python.
Serial.print(pulseY);
Serial.println(); //newline character to signal end of data for python
//Read data
if(Serial.available() >=2){
sd = Serial.read();
if(sd = \"x\"){
digitalWrite(xLed, High);
}
if(sd = \"y\"){
digitalWrite(yLed, High);
}
}
}
This would make two LED's light up, if the x,y values are beyond the given threshold respectively.
Now you may have a better understanding of how to communicate via serial. It is super simple, and very powerful.
Thank you for reading, Tech B.
Ref: PySerial Python Arduino Memsic 2125 Processing Processing Code Examples PySerial Code Example
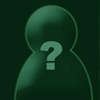
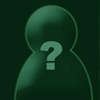
stealth- 15 years ago
Hmmm, pretty sweet stuff, techb. I still find it amazing that you can do this stuff in python, just makes me fall in love with it all over again :D
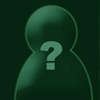
techb 15 years ago
@Moshbat Your right, it isn't verbal. Nor is you reading something online. It would be verbal if I presented this on youtube, with physical recording. I'm not relaying this information verbally. Its all document.
@stelth- and anyone who cares This Is going to be my next project. I have two arduino mini's and this would be GREAT for pen testing.
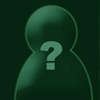
stealth- 15 years ago
Haha, yeah, I remember reading about that on Hack a day's RSS feed. Nasty little bugger, it is.
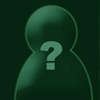
korg 15 years ago
Nice article, very informative. Never really used this, makes me want to investigate further.
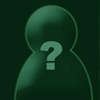
techb 15 years ago
After a trip with google, I guess your right in the sense of the common definition of verbal communication.
And even though the code isn't verbal communication, it teaches in the form of example. And the best way to learn something, like a new library or module, is to look at the code (opinion).