Classes in PHP 5
Classes in PHP 5
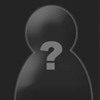
In this article, I’ll explain how to use classes in PHP 5. So, let’s start!
Every class definition begins with the keyword “class”, followed by a class name, followed by a pair of curly braces which enclose the definitions of the class’ properties and methods.
The class name can be any valid label which is a not a PHP reserved word (like public, private, addslashes, etc.). A valid class name starts with a letter or underscore, followed by any number of letters, numbers, or underscores.
A class may contain its own constants, variables (properties), and functions (methods).
<?php
class myClass {
public $myVar = "myVar";
public function myFunction() {
echo $this->myVar;
}
}
?>
The keyword $this is available in all classes. When you use the “get_class($this)”, you will get the name of the class name:
<?php
class myClass {
function getClassName() {
echo get_class($this);
}
}
?>
The output of this will be: myClass
Well, let’s say we include the file class.myclass.php (see below) in index.php (below too), where we want to use the class’ methods and properties:
File: class.myclass.php
<?php
class myClass {
public $myVar = "myVar";
public function myFunction() {
echo $this->myVar;
}
public function getmyVar() {
return $this->myVar;
}
}
?>
File: index.php
<?php
include('class.myclass.php');
$instance = new myClass();
echo $instance->myVar . "<br>";
$instance->myFunction() . "<br>";
echo $instance->getmyVar();
?>
The output of this will be: myVar myVar myVar
Let’s take a closer look at the code: First, we include the class file. If we wouldn’t do that, PHP gives an error that “myClass” is not defined. Then, we create a new instance of the class “myClass”. If you don’t set a new instance and just use myClass::getmyVar(), the script won’t work correctly because “$this” is not defined. With the just created instance of “myClass”, we can use the property “myVar” and the methods “myFunction()” and “getmyVar()”. The difference between the methods is that “myFunction()” echoes the variable “myVar” immediately and “getmyVar()” just returns “myVar”.
What about inheriting a class?
That goes with the keyword “extends”. Let’s take a look at the following example:
<?php
include('class.myclass.php');
class childClass extends myClass {
public function echoMyVar() {
echo parent::myVar;
parent::myFunction();
echo parent::getmyVar();
}
}
$instance = new childClass();
$instance->echoMyVar();
?>
This does exactly the same as the example with “index.php”, but now the class “childClass” extends “myClass”, what means it inherits it’s methods, properties, etc. However, if the function “getmyVar” in class “myClass” was declared with the keyword “private” instead of “public”, the script won’t work. Private functions are not visible for an inherited class.
You can also define “constants” in PHP classes. Constants always remain unchangeable Here’s an example:
<?php
class constExample {
const constVar = "constant value";
public function echoConst() {
echo $this->constVar;
}
}
$inst = new constExample();
$inst->echoConst();
?>
Well, let’s see how constructors/destructors work in the classes:
<?php
class baseClass {
function __construct() {
echo "Class constructed";
}
function __destruct() {
echo "Class destructed";
}
}
$inst = new baseClass();
?>
This will echo “Class constructed”, because you defined a new class. When the class gets destructed (when exit() or something is called), the script will output “Class destructed”. Of course, you can pass parameters with __construct and __destruct, as they work as normal methods.
Well, this are the very basics of classes in PHP. I hope you have learned something about this!
Black Wolf
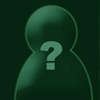
stealth- 15 years ago
Why no code tags? The code was pretty hard to follow due to the lack of code tags and how pretty much everything had the same name. Add that to the fact that there are already thousands of PHP tutorials out there that probably include this, and I can't rate this any better than average. It wasn't horrible though, just needed some more effort put into it.
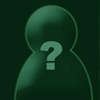
ghost 15 years ago
Sorry about the code tags. This is my first article, but didn't knew code tags were supported because they didn't work when I previewed it -.-
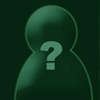
ynori7 15 years ago
Seems pretty basic. Not horribly written, but nothing special. Average rating from me.
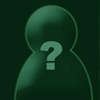
ranma 15 years ago
It's alright. But pretty basic stuff and would have liked to see more applications of the classes in PHP. As in, what can you do with these classes? give examples.
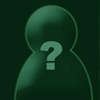
ghost 15 years ago
This is just enough of an article on PHP classes to make me want to go read an article somewhere else on PHP classes. It started off well, then just reiterated a few basics with an inheritance twist in there. Just learn from the criticisms here and write a more complete article next time. :) Average here as well.
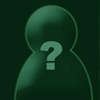
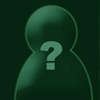
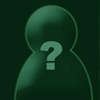
ghost 14 years ago
This is pretty basic yes. Although it says "Classes in PHP", not "Advanced usage of OOP in PHP5".
Although, I'd like to see more of a detailed comment on why and when to use classes. How it can be extended, abstracted and finalized and what it means should be included as well.
Average from me.