Basic PHP
Basic PHP
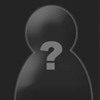
An introduction to PHP by BobbyB.
This is my first ever article. Hope you like it.
I'm going to cover some basics here - I'm new to PHP as well so I'm going to try and teach you some of what I have learnt.
First things first. PHP stands for Hypertext Preprocessor, and is a scripting language for the web. It can be placed anywhere in a page's source. Now on to how to use it.
You can type your PHP scripts in notepad. When you save them, save them as "whatyoulike.php". Make sure you have selected all files, and enclosed the name in double quotes. This prevents it as being saved as a txt file.
Here is a very simple PHP script. Since it is what most tutorials for most things do, this first PHP script will output 'Hello World':
The tag tells the browser that it is no longer PHP. The word 'echo' is a command, and it outputs any text that follows it that is contained with in double quotes. The semicolon tells the browser that the echo command is over.
So the script should output the text 'Hello World'
Here is another script:
In this script, I have introduced variables. Basically, variables store data. The $ sign tells the browser that a variable introduced. The text after the $ sign is the variable name. The = sign assigns anything after it to the variable. The echo command then outputs the variable as text.
So the script should output the text 'Second script'
In this script I have also introduced assignment operators. These are used to assign values to variables. THESE ARE IMPORTANT! Here is a list of the full range:
= e.g. x=1 means x=1 += e.g x+=1 means x=x+1 -= e.g. x-=1 means x=x-1 = e.g. x=1 means x=x*1 /= e.g. x/=1 means x=x/1 %= e.g. x%=1 means x=x%1 (x = the remainder of x/1)
NOTE: unlike most languages, variables in PHP do not have to have a variable type - any vaiable can contain any data.
Do you like these scripts? If so, lucky you. Here's another:
This script creates 3 variables: numberx, numbery and total. numberx and numbery are added together to and this value is assigned to total. Since numberx = 4 and numbery = 8, total = 12. total is then echoed.
In this script I have introduced arithmetical operators. These are used to change mathematical data. Here is a list of the full range:
- (Addition)
- (Subtraction)
- (Multiplication) / (Division) % (Modulous/Remainder of division) ++ (+1) – (-1)
Here are a few examples of how to use these:
$z = 4*2;
$z = 8
$z = 2; $z++;
$z = 3
$z = 4-2
$z = 2
$x = 6 $y = 4 $z = $x%$y
$z = 2
For my final script, I am going to introduce loops, and I am going to demonstrate the IF loop. So, here's the script:
This last script uses the if loop to test if a statement is true. The statement is enclosed after the word if in brackets. If the statement is true, the script will carry out the command the line below. If it is untrue however, the script will carry out the command after the else. Since 4>2 is true, the script carries out the command after the if and echoes "Who loves BobbyB? Everyone loves BobbyB!". Which is also true. The numbers could have been replaced with variables.
The 'is greater than sign' > could have been repalced with any other comparison operator. These are:
== (is equal to) != (is not equal to)
(is greater than) < (is smaller than) = (is greater than or equal to) <= (is smaller than or equal to)
That is the end of my tutorail. I hope I have outlined the basics of PHP for you. If you want to learn more, go to www.w3schools.com It's where I learnt all of this.
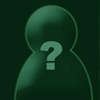
ghost 20 years ago
Yeah, i was going to mention the open tag. But, very good article, especially for your first one!
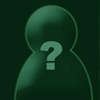
ghost 20 years ago
About that open tag - I think php fusion cuts it off for security reasons. I did on another site I posted it to.
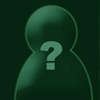
ghost 20 years ago
<html> <body> <?php echo "Hello World"; ?> </body> </html>
<html> <body> <?php $content = "Second script"; echo $content; ?> </body> </html>
:P
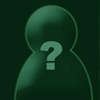
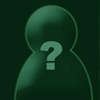
ghost 19 years ago
Very nice article good job i started learning php yesterday and already i feel that i have the hang of things. to me php is kinda a html ,javascript , & vb mix :p NICE JOB!!!
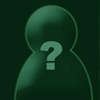
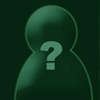
Thucydides 18 years ago
A good intro article. I just want to point out a small error though, PHP stands for PHP: Hypertext Preprocessor and is thus a recursive acronym (actually, a recursive abbreviation in this case), sort of like GNU Not Unix as another example.
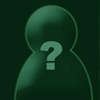
02nwood 18 years ago
For my final script, I am going to introduce loops, and I am going to demonstrate the IF loop. So, here's the script.
IF ISN NOT A LOOP IF ISN NOT A LOOP IF ISN NOT A LOOP
I SHALL GIVE AN EXAMPLE OF A LOOP IT IS NOT IF IT IS WHILE
i dont think you mentioned this in the article but with some functions you need to put { } after the function to tell the function what code to execute. if is not a loop a while loop goes something like this
$loop = "this code loops"; while (condition){ echo "loop: " .$loop "";
i am sorry but the if loop just qute basicly annoyed me
thanks doskey