Using PHP's GD library
Using PHP's GD library
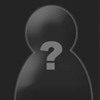
Using PHP's GD library
The GD library is included in php versions >= 4.3.0. It is an image library, that lets you create static or dynamic images, such as the ones you see in some people's profiles, stating their rank. The GD library works in much the same way to other imaging libraries. You start by defining a canvas or a set size, then had a variety of functions available to you to create shapes and text on the canvas.
Creating a canvas
You can create a variety of canvases, starting with either a blank canvas, or a previous image as the background. A common staring canvas can be created with the following code:
$im = imagecreate(400,320); imagecolorallocate($im, 255, 255, 255);
This creates a canvas ($im) which is 400px x 320px, with a white background. All colors in GD are inputed as RGB values, so (255,255,255) is white, and (0,0,0) is black. You could also start with an existing .png file with this code:
$im = imagecreatefrompng("bg.png");
Similar commands exist for other image formats, such as .gif, .jpg/.jpeg, and it even supports loading an image from a variable as a string.
Drawing
Once you have a canvas, you can begin to draw on it. Some common functions to draw are:
imageline — Draw a line imageellipse — Draw an ellipse imagerectangle — Draw a rectangle imagepolygon — Draws a polygon
With these functions, you can draw almost any shape you like. There are also 'filled' variations for each, so for example, there is imagefilledellipse. The only one which doesn't have a 'filled' equivalent is imageline (for hopefully obvious reasons).
Text
For creating text, you can either use GD's inbuild fonts:
imagestring — Draw a string horizontally
Or a postscript font of your choosing, which must be loaded with imagepsloadfont, then used with imagepstext.
Copying images
Occasionally, you might want to include another image in your code. This is entirely possible, using this command:
imagecopy — Copy part of an image
Note You must use this function after you have created your text and drawings, anything you draw afterwards will not be shown! It took me a while to work that one out :D
Finalizing your image and cleaning up
Once you have drawn your image, you must send the correct headers to the browser, and then send the image code for the browser to display it. This is usually done with the following 2 lines (for a png image):
header("Content-type: image/png"); imagepng($im);
You can the free up the resources used in creating the images by disposing of any fonts you might have loaded, and destroying both the image you were creating and any images you may have imported. Assuming you did not load fonts or additional images, you can do this with the following line:
imagedestroy($im);
That pretty much wraps up the basics. You can find more indepth information on GD, as well as syntax at: http://uk3.php.net/manual/en/book.image.php
Below is some sample code I wrote to help you piece together making a simple image with PHP and GD.
/––––––––––––––/
/––––––––––––––/
Running this code generated this image:
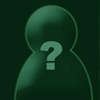
ynori7 15 years ago
Ah, very nice. This'll come in handy for that challenge I was thinking about writing. :D
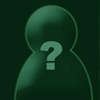
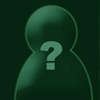
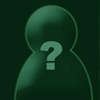
ghost 15 years ago
Good article, with easy explanations. Would be nice to see some more gd functions though eg. imagecreatefrompng, imagecopyresampled, imagecopyresized? Very good from me :D
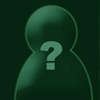
ghost 15 years ago
Edit: dunno why I mentioned imagecreatefrompng - you've got it in the article! Sorry man! :|
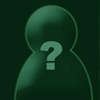
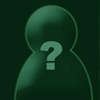
ghost 15 years ago
@ jjbutler88 = ha i see what you mean, will look into it, great article, got it : OWN work, and good structure. right.
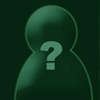
p4plus2 15 years ago
Very good, covers the topic well and doesn't over explain :) perhaps you could have placed the prototype function when you described each one. Though they are not hard to find so its no big deal.
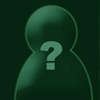