Introduction to Multiple Layered Architecture
Introduction to Multiple Layered Architecture
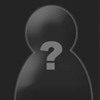
Introduction to Multiple Layered Architecture
Pre-require :
- Good knowledge of POO
============ Introduction
What is a multiple layered architecture ?
A multiple layered architecture is a way of coding that completely separate each part of the code that shouldn't interfer with each other and makes it possible to modify a layer without changing anything else to the other layer. Each layer is becomming independent of the other. This concept can be applied in many way. The most common of it is MVC (Model-View-Controller).
Where does it apply ?
For smaller code it's pretty useless to use this concept, but once your starting to code bigger thing it's the key to make your code better.
====================== How do we apply this ?
For this example, I'm going to use a website.
In the first, you need to ask yourself what are your going to need for that website. Do I need a blog ? Do I need a guestbook ? etc. In this website, we are going to have a blog and an image gallery.
After, you need to ask yourself what layer are you going to need ? Do I need database ? Do I need online payment stuff ? etc. For the exemple we are online going to need database for the blog. There is going to be 1 layer for the database, 1 for the output and 1 for the model. The layer for the model is the layer in which we are going to put all code that isn't related to the other. The layer for output is generally associated to template.
How do we code this now ?
Usually each layer is coded in a class or a series of class. The best example of that is a SQL class. Everyone has seen one before or even has coded one. These kind class are independent of the rest of the code. The model is the only layer that doesn't need class. Note that the class can call each other, but they must not have code that is specific to a layer in the other layer. This means that in the class for the output, I’m not going to see something like "mysql_connect". The general looking after your coding is done is :
include(\'class/Database.class.php\');
include(\'class/Output.class.php\');
$template = new Template();
$template->load(\'main.template.html\');
$db = new DatabaseConnection();
$result = $db->doThisQuery(\'SELECT * FROM exemple\');
for ($i=0; $i<count($result); $i++) {
$template->setValue(\'value\'.$i, $result[$i][\'field\']);
}
echo $template->output();
?>```
After all that what does it gives ?
Let\'s just say your client change his mind in the middle of the project and says \"I don\'t like MySQL, change it to PDO\". If your database wasn\'t a layer imagine the pain ! But since we coded in layer there is no need to panic. All we need to change is the DatabaseConnection class and the rest of the code won\'t change at all. That\'s the main strength of this concept and this is why it\'s often used in company. One of the other strength is that all the class or layer you coded can be reused in all your project, because remember they are independent of each other. So if you want to code many website, are you going to start them all from scratch because your code isn\'t reusable ? If it\'s coded in layer, all you need to recode is your model layer.
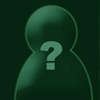
ghost 16 years ago
I like the introduction to the separation of layers and the reference to reusable classes… which yields reusable code. Unfortunately, this introduction failed to be complete by neglecting to demonstrate all three layers. It also lacks the type of organization that an introduction about separating the layers would imply. That is, each layer should be addressed separately, rather than a piece of code that combines both covered layers into one piece. Ultimately, this ends up feeling like an introduction written for someone who already knows the concepts… which is of little use to someone trying to learn them. Just because something is easily researchable online, that doesn't excuse a certain obligation to explain them (at least in short) in an article that seeks to be an "introduction". Rated "Average"… for being just that.
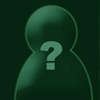
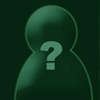
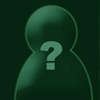
spyware 16 years ago
If you have nothing new to say, and we doubt your skills, and you're an idiot, don't bother replying on this stuff. Oh, real smart, saying Zeph is right and voting what he voted. Real safe. Real fucking stupid. Get the fuck outta here, go read something you might understand.
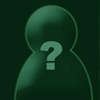
spyware 16 years ago
Ah. Well, good. You know what you're doing. I still stand behind my point though.
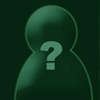
ghost 16 years ago
Zephyr_Pure -> Gonna see what I can do about the explannation of each layer more in precision. From that point of view it's clearly missing some information. I wanted to do it general, but it might was too general which isn't necesseraly good.
spyware -> For the pre-require, I putted that because multi-layer architecture is the "How To Use Better Class", so you got the know what are classes are and how to use them.
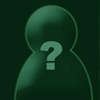
K3174N 420 16 years ago
oh my good god, spyware strikes again, i was saying it seemed interesting, and that i would (and have read up on it) that was my comment, anthing else would of been repeating, which im sure you would of called me an idiot for anyway, seriously spyware, shut the fuck up >.<
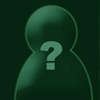
K3174N 420 16 years ago
jesus what is wrong with you people? i read the article, my opinion was started good, ended shit., which is basically what zep put in a more elegant manor,.. now seriously spyware, everytime i post ur there offending me, and just coz you have been here longer you get people defending you, it pisses me right off, your a fucking kid. grow up man.
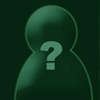
ghost 16 years ago
Dude, shut up and drop it. I'm sure I'm not the only one that thinks your whining about Spy is getting old. The reason you got shit over your comment is because you basically just said "I agree with ____" instead of contributing a unique perspective or at least an independent thought. That's the truth, and this is not the place for you to have your little revenge session. This is an article comment area… if you're not talking about the article, you need to shut up. It's your fault you got the responses you did. Learn how to contribute something at least halfway meaningful to an article's comments.
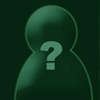
K3174N 420 16 years ago
stop defending the cunt. as i said before, if u read, my view is that it started well, interesting subject, half decant introduction, the it did not finalize attal, now i am sure, if i had of said those words instead of zep said it all, spyware would f still found room for a meaningless insulting post… and my post wasnt completly irelivent, i rated, and said i was gonna read up on it, which i have, so to all the mother fuckers giving me a hard time about it, go fucking grow up, im fed up of ya.
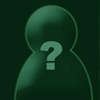
ghost 16 years ago
Shut up and drop it… this area is for article comments. Is it that fucking hard for you to read?
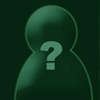
ghost 16 years ago
yo arto, your flames towards zephyr in his erticle about php coding still echo around in my head. I told you: "getta writin'"…So you did…Now let's look at your rating and article quality! I hate to say it…but…i told you so! To not make it more sad i won't even vote for it:happy: