Advanced C++ Pointer(2)
Advanced C++ Pointer(2)
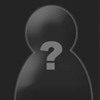
/*************************************** Advance C++ concept Pointer(2) by : Alka G Vulcan yake_2002 at hotmail dot com ***************************************/
<!–Pre requisite –!>
Understanding of pointer and basic C++ concepts, for more information about basic pointer concept read my “Advance C++ concept Pointer(1)”
<!–Intro–!>
In this article we are going to discuss about selecting members of objects through pointer, so we can prepare ourselves for Linked List data type and polymorphism, both my favorite. Also allocating multi dimensional pointers just like multi dimensional arrays.
<!–Main–!>
SELECTING MEMBERS OF OBJECTS THROUGH POINTER
Let’s make as simple class and experiment with it.
class student
{
public :
string name;
int age;
};
Now in our main()
int main()
{
student a;
a.name = Jimmy; //notice we used “dot” operator
a.age = 12;
Cout << a.name << “ “ << a.age; //notice we used “dot” operator
}
In this case we didn’t used pointer so using dot operator is perfectly fine. But when we declare an object with pointer we have to change it little bit.
This is our new main()
int main()
{
student *a;
a = new student; //memory allocation
a->name = Jimmy; //notice use of “->”
(*a).age = 12; //notice same as “->” will explain
Cout << a->name << “ “ << a->age; //notice we used “->” operator
}
Indirectly accessing the members of class through a pointer is clumsy, however if the indirection operator is used. So we know we have to dereference the pointer in order to use operator since it’s a pointer…
So one might think the following statement will access the name member of the class but it doesn’t.
*a.name = Jimmy; //no good code, won’t work
This won’t work because dot operator have higher precedence than the indirection operator, so the indirection operator tries to dereference “a.name”, not “a”. To dereference the “a” pointer, a set of parentheses must be used
(*a).name = Jimmy; //good code, will work
Because of the awkwardness of this notation, C++ has a special operator for dereferencing structure pointers. It’s called the structure pointer operator, and it consist of a hyphen and followed by greater than symbol, just like following example.
a->name = Jimmy; //good code, will work
The “->” operator takes the place of dot operator in statements using pointers to objects. The operator automatically dereferences the object pointer on its left and there is no need to enclose the pointer name in parentheses.
/* Following is a chart of some pointer pointing to object syntax. Ignore it if you don’t really understand pointer because it will confuse you even more….
Dereferencing pointers to objects.
(“s” implies pointer pointing to object “m” implies contents inside “S”)
s->m //accesses the m of the object pointed by s.
*s.m //access the value pointed to by s.p
(*s).m //acess the m member of object pointed to by s, same as “s->m”
*s->m //access the value pointed to by s->m
*(*s).p //access the value pointed to by (*s).p, same as “*s->m” */
MULTI DEMENSIONAL POINTER ALLOCATION
Now we are going to talk about how to have multidimensional pointer just like multi dimensional arrays. It’s quite simple once you remember the syntax. But for some reason remembering this syntax was one of the hardest thing I ever had to do in my career… Don’t know why… Maybe I’m retarded… :P
Declaring a 2 dimensional pointer is quite simple… just like following
int **ptr;//2 pointers because it’s double pointer.
But allocating memory for it is little tricky…
int **ptr;
ptr = new int *[5]; //notice “*” before [5]
for(int x = 0; x < 5; x++) //do it 5 times…
{
ptr[x] = new int [5]; //for each element, allocate
}
Upper code will allocate two dimensional pointer with 5 rows and columns, just like this
int array[5][5];
/* doing 3, 4, and x amount of dimensional pointer allocation should be obvious now… */
<!–end –!>
You may or may not able to feel the importance of pointer throughout my 2 articles. I don’t blame you because I didn’t see it either when I was in your position. But more and more I program and more and more I study, I feel imperative to understand and dig deeper. This is arguably hardest concept of C++, but it is absolutely necessary to understand, because without pointer you can’t fully perform C++‘s capacities. There are so many concepts that are just impossible to understand without pointers. I think my next article will be Linked List because it’s my second favorite data type as of now. I would do the polymorphism but then it needs understanding of class and inheritance. Any question, help, or simply want to discuss programming, just contact me through email or MSN.