Advanced C++ Pointer(1)
Advanced C++ Pointer(1)
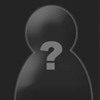
/*************************************** Advance C++ concept, Pointer by : Alka G Vulcan yake_2002 at hotmail dot com ***************************************/ <!–Pre requisite –!> Basic understanding of C++ along with Full understanding of array. Common sense
<!–Intro–!> I wasn’t able to find article about pointer in forum, and session over MSN seems not organized method to explain such concept to few friends of mine, so I figure I would submit article about it.
Pointer is arguably toughest concept in C++ to understand and utilize at its full capacity, along with polymorphisms depends on one’s opinion. If you are planning on going to software related field, it’s inevitable to learn pointer. Pointer variable, often called pointer, is a variable that holds addresses, and by through that address you can indirectly manipulate data stored in other variables.
Pointer facts
- working directly with memory locations that regular variables don’t give access to +working with strings and array +creating new variables in memory while program is running +creating arbitrarily-sized lists of values in memory +pointer holds only one thing, address
<!–Main–!> DECLARATION of int and double pointer is as follow
int *iptr;
double* dptr;
/* As you can see the spacing between asterisks and variable name doesn’t really matter. Also it’s critical to understand variable iptr does not mean that iptr is and integer variable, it means that iptr CAN hold address of an integer variable. */
ASSIGNING VALUES to pointer. int *iptr; //declaring pointer int x = 10; //declaring a variable with a value iptr = &x; //assigning variable’s ADDRESS to a pointer
In order to assign value to pointer, we would have to approach indirect method. First we declare pointer and a variable with value and assign variable’s address to pointer, since pointer can only have address.
As you can see we can dereference variable by using ‘&’ sign.
/* you can see the address of variable by “cout << &x; “. Depends on your compiler or operating system it will show hex decimal or else. */
So after following code if we run this code…. cout << iptr; // will output something like 0x12345 or whatever cout << &x; // will output same as above both will output same address.
In order to see what value is in pointer’s address by reference….
cout << *iiptr; // will show 10 since 10 is in x’s address
cout << x; //will output same as above
both will output same value.
/* As you can see asterisks have many job in C++, multiplication, pointer and possibly many more depends on operator over loading. So it’s critical to organize your code to not to confuse yourself.*/
RELATION with array It turns out array and pointer is very similar, in fact array is nothing but a variable that holds address like pointer as well.
For example….. int array[] = {10, 20, 30, 40, 50}; int *ptr; ptr = array; //notice we don’t have to dereference array by &, because array is address.
cout << array; // will show address of begging of array, may vary depends on compiler.
cout << ptr; //output same as above.
cout << *array; //will show first value in array, notice I’m using reference, “*”, just like a pointer.
cout << *ptr //will output first value in array just like above.
cout << ptr[0] //this also will output first value in array, notice I’m indirectly accessing array through pointer.
// Also in side note it’s good to know pointer’s way to access object’s member
cout << *(ptr +1) // will output first element of array
cout << *(ptr + 2) //will output second element of array
/* Pointer work with array’s method of “[]”, and array work with pointer’s method “ *(ptr +1)“ way. */
I don’t know how familiar you guys are with arrays and its arithmetic. But pointer’s arithmetic is quite similar to array’s arithmetic
For example int array[] = {1, 2, 3, 4, 5}; int *ptr; ptr = array; //1. following is array arithmetic and will output 5 elements in array. For(int x = 0; x < 5; x++) { cout << array[x] << endl; } //end of array arithmetic
// 2. pointer arithmetic and will output 5 elements in array. This also will output all 5 elements
for(int x = 0; x< 5 ; x++)
{
cout << *(ptr + x) << endl;
}
// 3. following is also result same output as above
for(int x = 0; x < 5; x++)
{
cout << *ptr << endl;
ptr++; //notice incrementing pointer’s address.
}
//end of pointer arithmetic
/* becareful using option number 3 because as you can see ptr got incremented 5 times and never got back to original place, there for after option number 3’s execution, if you output “cout << ptr; “ you will access 6 th member of array which we didn’t assign. And yes C++ will compile that no problem with no warning. Thus you may accidently alter or change variable that happens to located next of array.*/
RELATION with function Now passing pointer to function is quite simple.
Void doubleValue(int *val) // * before val indicating it’s a pointer
{
*val *= 2; // first * for referencing pointer and second * for multiplication
}
/* notice val’s value will change throughout entire program because what’s in address changed. Unlike normal variable when they passed to following function
Void doubleValue(int val) // val in here is variable not a pointer
{
val *= 2; // first * for referencing pointer and second * for multiplication
}
val won’t be double its original size in “main()”. Because COPY of variable’s value get passed to function and that copy gets manipulated not the original value. We can fix It by following code.
Void double value(int &val) // val in here is variable not a pointer
{
val *= 2; // first * for referencing pointer and second * for multiplication
}
just remember to add & before variable name in argument on function call */
Now returning pointer from function can be tricky….
DYNAMIC MEMORY ALLOCATION
Dynamic memory allocation is nothing but reserving memory for future use, just like array. And it’s most common use is in case of returning pointer from a function.
For example, execution of following code is almost identical to “int array[5];”…. int *start () //notice * before name, it indicates that it’s returning a pointer { int *ptr; ptr = new int [5]; //allocation of memory by using “new” key word for(int x = 0; x <5; x ++) //fill every object with 0 ptr[x] = 0; return ptr; //return pointer }
/* if you return pointer without memory allocation, data will be lost. Remember pointer is an address. And variable declared inside of function will be deleted as function exit. There for without allocation data will be lost while with allocation data will be save in safe location. Although dynamic memory allocation may seem to protect you from buffer overflow or boundary overflow, it won’t prevent from such accident. Memory allocations are only for reserving memory for a pointer.*/
MMEMORY DEALLOCATION Deallocation is freeing the reserved memory by allocation. If you don’t deallocate the memory your computer may never get to use that memory again because it thinks it’s reserved. But now days most operating system automatically detects and deallocate such memory but still, it's imperative to develop good habit.
Int*ptr;
ptr = new int [5];
delete [] ptr; //deallocation, notice I didn’t put number in bracket. No matter the number of object allocation you will be able to delete all by empty bracket.
/* It’s critical that you only deallocate what is allocated before. Otherwise either your program will not function as you expected or won’t compile at all. */
<!–End–!>
I understand I’m not the best teacher and this isn’t the best way to illustrate. My purpose of this article is not to give you 100% understanding of pointer, but give you basic concept and direction to practice pointer. Because I believe practice is the only method to fully understand pointer, just mass with pointers and you will understand.
There are several stuff that I didn’t mentioned, such as -> operator instead of dot operator, and others. I may write another article later if response is good, or you cans ask me on my email at very beginning.
Any questions contact me by email at above, and plead don’t ask me if I can help you hack your friend’s computer or whatever.
p.s. no tab thingy kills me!!!! :P
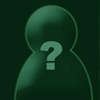
ghost 17 years ago
Awesome! I just started learning about pointers in class, but was having a little trouble understanding them (mainly because I can't understand half of what my teacher says because of his accent :()
Anyway, now I understand it much better, thanks :)
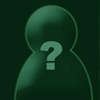
Uber0n 17 years ago
Not very advanced, I mean this is just the basics of working with pointers… Well written though. I'm sure this will help beginners understanding pointers better :happy:
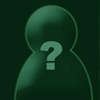
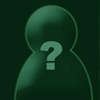
ghost 17 years ago
oh ya… there is sudoku solving C++ program code i submitted and that may help understand pointer as well….
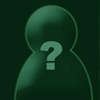
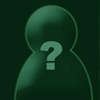
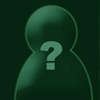
ghost 16 years ago
Ouch. My eyes are realy hurting. To be honest, it is a good text but the many small eroors and stupid use of different code comment syntaxes makes this completely unreadable. Sorry.