Java file Input
Java file Input
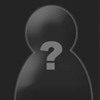
There are very few articles for Java on HBH, so i decided that I would add some of my own.
The first thing I'm going to discuss is File Input using the Scanner utility. You should have at least a basic understanding of Java before viewing this, I promise it will make more sense that way. ^^
When using the Scanner utility it is necessary that you import the proper classes. Rather than going into specifics on which need to be imported I will also explain the use of a wildcard ("*") that can be used when importing classes.
The wildcard is used in the format of anything such as "import java.io.*;", which will add all of the java.io classes to your program, it can be considered a bad coding habit, but when you're just starting out it really isn't all that bad, although it can be a hard habit to break.
Anyways, for this we will be using "java.io.*;" and "java.util.Scanner", notice that the s in Scanner is capitalized, java IS case sensitive, so the capital s is very important. As of right now we have:
import java.io.*; import java.util.Scanner;
and it will be assumed that you also have enough knowledge of java to get a little bit farther with:
public class finput { public static void main(string[] args) { } }
When using the java.io (input output) method you will in most cases be required to add "throws IOException" after you declare "public static void main(string[] args)". This is used to help the program halt "gracefully" if an error occurs. Just look at it as Java's built in error handling system.
Once you have gotten this far, you may call in a Scanner using:
Scanner stdin = new Scanner(new File("FILENAME HERE"));
You may use a file name or file directory here, and the program will look there for the information you are directing it to.
A very simple way to use the Scanner at this point is to use "stdin.next();" which will grab the first piece of data from the file, and when used the second time it will grab the second piece of data, separated by spaces and end lines, and so on and so forth.
So a basic idea of how this will work is to just run a piece of example code:
import java.io.*; import java.util.Scanner;
public class finput { public static void main(string[] args) throws IOException { Scanner stdin = new Scanner(new File("hello.txt"); String information = stdin.next(); System.out.println(information); stdin.close(); } }
This will look in the directory that your program is stored in for the file "hello.txt", and will print the first word, integer that is stored in it.
Well anyways, this is my first guide, so please don't be to harsh on it ^^ Requiem
BTW, sry its ugly, I\'m not sure how to adjust code blocks and the works :(
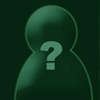
korg 17 years ago
Good article for people learning java. Lacks a little more content but otherwise really good 7/10
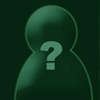
ghost 17 years ago
Good job mate, I'm currently taking a Java class and I wish I would have talked to you about 4 weeks ago. Had a project involving I/O stuff and managed to do it, but in a MUCH uglier/insecure way. This is basically what I was searching for.
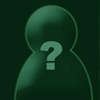
ghost 17 years ago
sorry about the lacking content, I planned on writing several articles to go along with this rather than one large article. I've been kind of sidetracked lately however, trying to prepare for my CCNA.