Introduction to C++
Introduction to C++
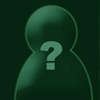
- In C++ we have different data types but since this is an introduction I will only talk about the most important, or most used ones.
- | NAME | SIZE | DESCRIPTION ||—————————————————–|| char | 1byte | Character or small integer. || short | 2bytes | Short Integer. || int | 4bytes | Integer. || long | 4bytes | Long integer. || bool | 1byte | Boolean value(true or false). || float | 4bytes | Floating point number. || double| 8bytes | Precission floating point number. |
- Since we can use logical operators we can also use logical expressions with truth tables–NOT–| a |!a ||—––|| T | F || F | T |
- –AND–| a | b | a && b ||––––––––|| T | T | T || T | F | F || F | T | F || F | F | F |
- –OR–| a | b | a || b ||––––––––|| T | T | T || T | F | T || F | T | T || F | F | F |
Introduction to C++
In this tutorial I will cover the basics of C++ for people that have no experience whatsoever with the language. First thing you need to remember is that C++ is case sensitive so 'int' is not the same as 'Int'. I'll skip the basic output(Hello World) program since it's been covered before. Lets go right ahead and start with declaring variables.
In C++ we have different data types but since this is an introduction I will only talk about the most important, or most used ones.
| NAME | SIZE | DESCRIPTION | |—————————————————–| | char | 1byte | Character or small integer. | | short | 2bytes | Short Integer. | | int | 4bytes | Integer. | | long | 4bytes | Long integer. | | bool | 1byte | Boolean value(true or false). | | float | 4bytes | Floating point number. | | double| 8bytes | Precission floating point number. |
Here we see all the different data types. So the way we declare a variable of any type would be: data_type name; we could also assing a value to the variable as soon as we declare it by doing this: data_type name = value;
So let's try a sample program using some of the data types specified before and see what happens. SOURCE
#include <iostream>
using namespace std;
int main()
{
int age;
double height;
cout << \"How old are you? \";
cin >> age;
cout << \"How tall are you?(enter value in meters) \";
cin >> height;
cout << \"AGE: \" << age << endl
<< \"HEIGHT: \" << height << endl;
system(\"pause\");
return 0;
}
OUTPUT
How old are you? 19
How tall are you?(enter value in meters) 1.78
AGE: 18
HEIGHT: 1.78
Press any key to continue . . .
What our code here is doing is asking for the age and height. The 'age' variable is an int so that means the user has to enter a number which will be stored here. In the case of the 'height' variable the user has to enter a number but now the number can either have decimals or not. Thats it for this part so we'll move on to conditional statements now.
There are several types of conditional statements in C++ and now I'll explain how they work. –IF– if (testexpression) { //if true, do something. } In the above structure, if the testexpression is true then the actions will take place. This is the only one choice. In many cases, we may want to do something else when testexpression is false. –IF, ELSE– if (testexpression) { //if true, do something. } else { //else do other thing here. } Same as the first example only that in this case if its false it does something else. –IF, ELSE IF, ELSE– if (testexpression1) { //if testexpression1 is true, do action #1 here. } else if (testexpression2) { //if testexpression2 is true, do action #2 here } else if (testexpression3) { //if testexpression3 is true, do action #3 here } else { //if none of the expressions before are true, do this last action } So this is conditional statements and remember this could be used with either boolean functions or logical operators. For example:
#include <iostream>
using namespace std;
int main()
{
bool value = false;
int a = 1;
int b = 2;
if(value)
{
cout << \"First condition was true\" << endl;
}
else if(a > b)
{
cout << \"Second condition was true\" << endl;
}
else
{
cout << \"No condition was true\" << endl;
}
system(\"pause\");
return 0;
}
OUTPUT
No condition was true
Press any key to continue...
Since we can use logical operators we can also use logical expressions with truth tables –NOT– | a |!a | |—––| | T | F | | F | T |
–AND– | a | b | a && b | |––––––––| | T | T | T | | T | F | F | | F | T | F | | F | F | F |
–OR– | a | b | a || b | |––––––––| | T | T | T | | T | F | T | | F | T | T | | F | F | F |
we can use these in this way: if( (a>b) && (value) ) { //do this. }
NOW we're done with conditional statements and logical operators. Next part of the tutorial will be loops so lets continue.
Lets say we have a program and we need it to run something several times or until some condition is true. Now I will explain the main loops we use. –FOR– Well the for loop is very easy to use so lets just get right into it and I'll explain whats going on. for(int i = 0; i < 10; i++) { //do something. } This is very simple to understand. The first thing is either declaring a new variable or assigning a new value to an already existing one. Next we set the condition which says that the loop will go on until i < 10. Last part says that everytime the loop passes it adds one to the i variable. Next is the while loop. –WHILE– This isn't that hard either. Lets see how this works. while( a != 1 ) or while ( boolean_variable ) So this says that while the condition is not true the loop will go on. We could use either logical operators or boolean values here for this loop. Now next is something very similar to this and its called the do, while loop. –DO, WHILE– Do while loop works the same way as the while loop but this time it lets you do something before you check the condition and decide if the loop goes on or not. Here is the way to use this. do { //do something. }while( a == 1 || b == 1 ); Like I said before here we do something and then check the condition of the loop. If its true the loops goes on and if not the loop quits.
We're finally done with loops and try not to have too much fun writing infinite loops this way. Now the next part will be the switch statement.
The switch statement is another kind of conditional statement. I'm writing it completely separated to the other conditional statements because this is very different in a way but can be also used to replace the if-else statements. Here's how we use it.
switch ( integralexpression )
{
case exp1:
statement1;
case exp2:
statement2;
default:
statementn+1;
}
There are several things about this that we have to consider. First would be the integralexpression part. From my experience this could be either an int or a char variable. If we use int we would write it like this.
switch ( integer )
{
case 1:
//do this.
break;
case 2:
//do this.
break;
default:
//do something different.
break;
}
and if we use char it would be like this
switch ( character )
{
case 'a':
//do this.
break;
case 'b':
//do this.
break;
default:
//do something different.
break;
}
Probably two of the most important things in switch statements would be break and default. If we dont put break then the whole condition statement would be useless. The default part just says that if none of the cases is true then do that. Switch statements are the best to use if you're writing some sort of menu so keep that in mind. I don't know what more i can say for this so I'll just leave it at that.
So this is my introduction to C++. Hope you guys can learn from it and if have fun trying new things out and mixing all these things together to write some great programs.