HTTP Authentication with PHP.
HTTP Authentication with PHP.
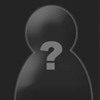
Note: The HTTP Authentication hooks in PHP are only available when it is running as an Apache module and is hence not available in the CGI version.
Alright lets first start of by having a look at the header that needs to be sent: Some of you may have noticed when trying to break into a .htaccess secured folder that it gives you something like: 401 Unauthorized. Well that's atually what makes your browser to create this login box. So this idea is basiclly based on tricking the browser with that sort of header.
To send the right type of header thru PHP you use this:
header(\'WWW-Authenticate: Basic realm=\"Your Realm\"\');
header(\'HTTP/1.0 401 Unauthorized\');
The realm is your name of choice, can be anything.
PHP stores the values entered in the boc in two variables: $PHP_AUTH_USER ($_SERVER['PHP_AUTH_USER']) and $PHP_AUTH_PWD ($_SERVER['PHP_AUTH_PWD']).
So lets have a look at a basic script that checks the username and password in htaccess style.
if (!isset($_SERVER['PHP_AUTH_USER']) || !isset($_SERVER['PHP_AUTH_PWD'])) { // If user isn't logged in then prompt for username and password. // Start_Header output header('WWW-Authenticate: Basic realm="My Realm"'); header('HTTP/1.0 401 Unauthorized'); // End_Header output echo 'You need to be logged in to view this site'; // Text to show if user pressed cancel instead of login exit; } else { if($_SERVER['PHP_AUTH_USER'] == "username" && $_SERVER['PHP_AUTH_PWD'] == "password") { // Code here if user successfully logged in. echo 'You are authorized'; } else { // Username or password was incorrect, prompt to login again. header('WWW-Authenticate: Basic realm="My Realm"'); header('HTTP/1.0 401 Unauthorized'); echo 'You need to be logged in to view this site'; exit; } }
So i guess you read the comments in the code huh? If not, then do it! Anyway, you replace "username" and "password" with your credentials of choice.
As you may understand this way is far more dynamic then basic htaccess and can be used with database aswell.
Althou, some people that's using this has problems with the logout feature of the site (if needed). By googling i found out that making a new prompt for username and password removes the cache for the currently logged in user. So by sending the header again you can logout. Geniou huh? Althou i had some problems with that and "Headers allready sent from line blablabla" in PHP so i found an easier way to do this, althou this may not work on all browsers (Safari for example).
Some of you people may know that you can automaticly login in a htaccess protected area by making this type of URL: http://username:password@site.com/
Well, by sending false credentials our cache gets deleted. So a quick way to logout as i use is: http://logout:@site.com
Hope someone finds this article usefull so my 20minutes of typing wasn't good for nothing xD
~root_op
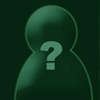
ghost 17 years ago
Well i was kinda tired when i wrote this, so bare with me xD Thx for your response mosh :)
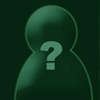
mido 17 years ago
Actually, I knew this, but haven't tried; not experienced with auth's. But found it somewhat informative. But I only used .htaccess to do this, without PHP involved. But anyways, nice article. Very Good
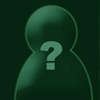
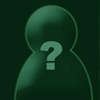
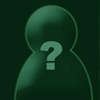
ghost 17 years ago
Nice content ? Your just presenting an Hello World. And your saying that htaccess isn't good. Your making a really big mistake. Read up more about htaccess and you'll see that it's by far better than using this kind of script. The only use of that kind of script is when you don't have htaccess which is really rare.
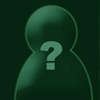
ghost 17 years ago
Well, lets say you want that kind of protection on your site, wich has like 20000 users, you want a htpasswd file with 20000 entries? Better of with database access instead.
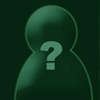
ghost 16 years ago
http://www.hellboundhackers.org/articles/258-htaccess-Password-Protection.html
well, it is something like mine.. :D