Assembly Basics
Assembly Basics
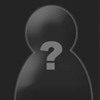
Assembly Basics––––––––––––––––––– by blackmind
Lets take a look at our first sample program:
.MODEL small .DATA printme db "Hello World!",13,10,'$' .CODE mov ax,@data mov ds,ax mov dx,offset printme mov ah,9 int 21h mov ax,4c00h int 21h
I bet your saying it looks like a bunch of garble right now but after I explain the different instructions and what they you will see what a simple program this is.
.CODE - Is a syntax for everything below is the running code
MOV - move a value from one place to another syntax: mov destination, source example: mov ax,10 ; puts the value of 10 in the register ax mov cx,ax ; puts the value contained in the register ax into cx
A register is a series of memory cells inside the cpu itself. Because registers are inside the cpu there is very little overhead in working with them. Storing a value in a register is much faster than storing it in RAM or on your hardisk. To store a value in memory the data has to travel out of the cpu, and wait its turn to get stored in memory. To store a value on the hard disk the data must travel out of the cpu, into the harddisk controller chip, and wait its turn to be serviced by the hard drive. You can see why registers are so much more efficient. This is why we use them in assembly.
The registers known by their specific names:
AX Accumulator BX Base register CX Counting register DX Data register DS Data segment register ES Extra segment register SS Battery segment register CS Code segment register BP Base pointers register SI Source index register DI Destiny index register SP Battery pointer register IP Next instruction pointer register F Flag register
There are four general purpose registers, AX, BX, CX, and DX. These are the registers you will be using often. Each of these general registers are 16- bit. They also have 8-bit counterparts.
AX 16 bits AH 8 bits AL 8 bits
AH being the high bit, and AL being the low bit. Together AH and AL make AX.
Each register has a specific purpose. The purpose of each register will be explained later in the tutorial. Now that we understand what a register is, lets move on with our analysis of the program.
INT - calls a DOS or BIOS interupt. You can think of an interupt as a function that preforms a task you would not normaly write a function. An example could be changing the video mode or opening a file. syntax: int interuptnumber example: int 21h ; calls the DOS interrupt int 10h ; calls the video bios interrupt
So now that we have that information under our belt lets look at see what the program actaully DOES: (the different parts will be color coded later)
- .MODEL small
- .DATA
- printme db "Hello GURU!",13,10,'$'
- .CODE
-
mov ds,axmov ax,@data
-
mov ah,9 int 21hmov dx,offset printme
-
int 21hmov ax,4c00h
Part 1. This is a compiler directive that tells it what memory model to use. For our purposes all we need is the small model. An explanation of each type can be found at ftp://x2ftp.oulu.fi/pub/msdos/programming/docs/asmtutwp.zip. Part 2. This is another compiler directive that tells the compiler the data segment is starting. Part 3. This line declares a peice of data. syntax: dataname db data In this case we declared a string named "printme" that contains a return (13) and linefeed (10) at the end (we will ignore the '$' for now). Part 4. This line informs the compiler the code segnment is starting. Part 5. These two lines of code set the register ds to point to the data segment. Without these two lines of code the program would crash. Isn't it a coincidence that the ds register is called the "data segment register?" You could make it point to any segment you wanted, however if you want your program to work I would suggest using the above example. Note we did not use "mov ds,@data." The reason for this is you can not assign a segment to ds directly. You must assign a register containing the value of the segment you want then assign that register to ds. Part 6. By the rules explained above this obvisly moves the offset (memory address) of printme into dx and puts a value of 9 into ah and then calls int 21h (the DOS interrupt). This block actually prints the message to the screen. The first two mov commands set up the message to be printed when the interrupt is called. The '9' in ah tells DOS that we want the print message function and this function expects the message to be accessible via dx (ergo "mov dx,offset printme"). Part 7. Without this block of code the program would crash. It is the equivalant of exit(); in C. The '00' tells DOS what exit code the program is leaving with.
Thats the basics of assembly of course the registers will change from system to system so it will change names sometimes you need a $ in front but if you read up on other systems they will tell you the specific of each one.
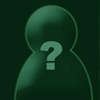
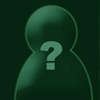
ghost 17 years ago
you should really told every one the pro's and con's of the language and what they need first
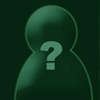
ghost 17 years ago
id like to mention that this article uses INTEL syntax, so it will work with microsoft assemblers like masm32 and debug.exe, but not with as or other AT&T syntax assemblers. good article though
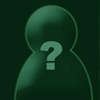
ghost 17 years ago
oh, and "Thats the basics of assembly of course the registers will change from system to system so it will change names sometimes you need a $ in front but if you read up on other systems they will tell you the specific of each one." is kinda wrong. $s are for AT&T syntax and the syntax of a lot of opcodes are reversed in AT&T. rated good :)
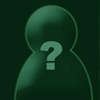
ghost 17 years ago
http://library.thinkquest.org/22447/asmbasics.html
This is just a copy and paste? Or did you write the other one? Bit outdated… an article explaining a basic hello world in MASM would have been more useful to members here… very few would bother with INTs now.
For anyone who is interested, google for Icezilion's asm tutorials. They're a good place to start.
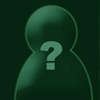
ghost 17 years ago
the one is from my class that i wrote so i just copied it from my computer source of it. and i am writting a more up-to-date one!…PLEASE RATE GUYS
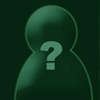
ghost 15 years ago
Consider covering 32bit or more for your next, but what I'd really like to see is a tut for SSE or maybe smc. Good for VERY beginners though.