Writing Games in Flash -- Part II
Writing Games in Flash -- Part II
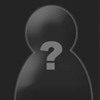
[Edit]Note: at the time of publishing, AS 3 had not yet been released. This tutorial assumes AS 2 [/Edit]
Introduction Welcome to my article on writing games in Flash part II!
If you haven’t done so already check out tutorial number 1
As promised I will cover topics mentioned in the first article in addition to several new concepts: *Health Bars *The Distance Formula *Projectiles *Rotation
Jumping right in…
Health Bars Health bars can be extremely helpful in writing games. Although called health bars you can use this concept to represent any value in a game, time remaining, hp, mp, reload times, ammo, whatever you need.
Alright, so when working with healthbars, we will use two variables: health and maxhealth.
health = 50;
maxhealth = 100;```
The code:
Lines 1 & 2 declare two variables, health and maxhealth. The variable health will change as a character gets wounded, but maxhealth will always remain the same. So lets say the character has 50 health and 100 maxhealth, we know they are at 50% (50/100 * 100) health.
But what if the two numbers are not so easy to calculate? Maybe 26/31? Turning this into a percent is fairly easy. You probably do this every time you get a test back from a teacher. Divide the two numbers, then multiply by 100.
So 26/31 * 100 = 83.8709677%
A number with decimals like the number above will not work with the healthbars we are about to create. So we can use the Math.round(number) to round this number off nicely.
```markup
health = 26;
maxhealth = 31;
currenthealth = Math.round(health/maxhealth);
//returns 84```
Now that we have turned our health and maxhealth values into a clean number between 1 and 100, we can continue building our healthbars.
Create a new movieclip. Name it 'healthbar'.
Give the movieclip 100 frames. Im not going to cover animation in this tutorial, but make your healthbar however you like it, being full at frame 100 and being empty at frame 1.
Add this script to the first frame of the movieclip:
```markup
stop();```
This stops the movieclip so the healthbar doesn't keep filling and emptying itself.
Now, whenever the value of the healthbar needs to be changed, we will:
* Calculate currenthealth (health/maxhealth * 100)
* Set frame of healthbar to currenthealth
For example our hero has 10/10 health. Then he gets shot in the balls. Now he has 2/10 health, and 2/10 * 100 = 20. So we go to frame 20 of our healthbar where it is one fifth full.
Application of this method: In the example, we have a movieclip 'healthbar' and two variables with input bars ('_root.health' and '_root.maxhealth').
This script goes on the healthbar itself
```markup
onClipEvent (enterFrame){
currenthealth = Math.round((_root.health/_root.maxhealth)*100+1);
this.gotoAndStop(currenthealth);
}```
Explanation:
Line 1: Tells flash to run this script every frame (enterFrame)
Line 2: Uses the variables to calculate current health (And adds one. Base zero issue, don't worry about this)
Line 3: Sets the frame of the movieclip to the currenthealth number
Line 4: Closes the enterFrame event
Congratulations, you've got your healthbar :)
So pretty much, turn variables into a percent, round the number to an integer, and tell healthbar to go to that frame.
**The Distance Formula**
Being able to find the distance between two points is very useful. Which enemy is closest? Where is the closest repair station?
[Example](http://i153.photobucket.com/albums/s216/digital_fire_tutorial/distance_formula.swf)
You probably learned the distance formula in school, but i'll go over it again in case you're a bit rusty ;)
The distance formula is derived from Pythagoren's theorem. Pythagorean's theorem states that a^2 + b^2 = c^2, where a and b are legs of a right triangle and c is the hypotenuse of that right triangle.
So lets add points red and blue to the triangle, and give them x and y coordinants x1, y1 and x2 y2. Don't be scared by the numbers, were simply stating that 2 seperate x and y coordinants exist.
Now we can find the length of the bottom side of the triangle by subtracting x1 from x2.
In the same respect, we can find a by subtracting y1 from y2.
Now that we have a and b we can find c using pythogorean's theorem!
c^2 = (x2-x1)^2+(y2-y1)^2
c = squareroot[(x2-x1)^2+(y2-y1)^2]
Therefore:
although this looks complicated, it really is not.
And don't worry about subtracting y1 from y2 or y2 from y1. It will come out the same either way. So
squareroot[(x2-x1)^2+(y2-y1)^2] = squareroot[(x1-x2)^2+(y1-y2)^2]
there you have it! The math at least. Now applying this to flash:
In the example this code is on point b (which is a movieclip):
```markup
onClipEvent (load) {
function CalcDistance(x1, y1, x2, y2) {
//Distance =SquareRoot of [ (x1-x2)^2 + (y1-y2)^2 ]
DeltaXSquared = (x1-x2)*(x1-x2);
DeltaYSquared = (y1-y2)*(y1-y2);
Distance = Math.sqrt(DeltaXSquared+DeltaYSquared);
return Distance;
}
}
onClipEvent (enterFrame) {
mydistance = CalcDistance(this._x, this._y, _root.a._x, _root.a._y);
_root.distance=Math.round(mydistance)
}```
Line 1: Tells flash to run this code when the movieclip loads (load)
Line 2: Defines a function. Functions are groups of code that can be run together to perform tasks.
Line 3: Comment.
Line 4: Sets the variable DeltaXSquared to the difference of the x values of the points squared.
Line 5: Sets the variable DeltaYSquared to the difference of the y values of the points squared.
Line 6: Sets the variable Distance to the square root of the sum of DeltaXSquared and DeltaYSquared
Line 7: Returns the value Distance. This is how information is retrieved from functions.
Line 8: Ends the function
Line 9: Ends the load handler
Line 10: Tells flash to execute the following code on every frame (enterFrame)
Line 11: Calls the function from line 2, feeding it values of the x and y locations of the two points
Line 12: Sets the text on the stage to the distance
Line 13: Ends the enterFrame handler.
Thats it! Although directly this may not seem useful, we can apply this other concepts. Such as...
**Firing Projectiles**
[Example](http://i153.photobucket.com/albums/s216/digital_fire_tutorial/firing_projectiles.swf)
Putting it all together!
The example shown above is quite complicated, and will use just about everything youv'e learned so far, including movieclip duplication and the distance formula. Also a little bit of algebra helps a lot :)
The plan:
1) We are going to have a movieclip named "bullet" which will be black dot. It will be duplicated over and over again to be fired at the mouse.
2) We are going to have a movieclip named "tower" which will fire the bullets every so often.
3) The variable _root.i will give each bullet a unique name
Create a movieclip named bullet, drag it onto the stage and name it bullet
Create a movieclip named tower, drag it onto the stage and name it tower
Alright, first lets program the tower.
```markuponClipEvent (load) {
count = 0;
maxcount = 10;
}
onClipEvent (enterFrame) {
count += 1;
if (count>maxcount) {
duplicateMovieClip(_root.bullet, "bullet"+_root.i, _root.i);
_root.i += 1;
count = 0;
}
}
The How: Each frame we add one to count, and once this happens 10 times we duplicate the movie clip with a new name
The Code: Line 1: The load event handler Line 2: Sets the variable count to 0 Line 3 Sets the variable maxcount to 10 Line 4: Ends load handler Line 5: Starts enterFrame handler Line 6: Adds 1 to the variable count Line 7: Checks to see if count is greater and maxcount Line 8: If it is, it duplicates "bullet", gives it a new name "bullet"+i and puts it on its own layer Line 9: Adds 1 to _root.i for a new unique name Line 9: Sets count back to 0 Line 10: Ends if statement Line 11: Ends enterFrame handler
So there is our tower, which will duplicate our bullet every 10 frames.
Now lets program the bullet.
onClipEvent (load) {
this._x = _root.tower._x;
this._y = _root.tower._y-40;
speed = 6;
x1 = this._x;
y1 = this._y;
x2 = _root._xmouse;
y2 = _root._ymouse;
mydistance = distance(x1, y1, x2, y2);
changex = x1-x2;
changey = y1-y2;
clicks = mydistance/speed;
xmove = -changex/clicks;
ymove = -changey/clicks;
function distance(x1, y1, x2, y2) {
xbatch = (x1-x2)*(x1-x2);
ybatch = (y1-y2)*(y1-y2);
mydistance = Math.sqrt(xbatch+ybatch);
return mydistance;
}
}
onClipEvent (enterFrame) {
this._y += ymove;
this._x += xmove;
}
The How: Each frame, we find the distance between the bullet and the mouse, divide that by the speed at which the bullet moves each frame to figure out how many frames it is going to take to complete the journey. Then we only think about 1 such "click" or how far it moves on one frame. We take the total distance of the two points, divide that by ‘clicks’ to find how far the bullet should move on each axis each frame. Then the bullet moves this amount each frame.
That is a big chunk of code, but its actually pretty simple. Lets break it apart and look at it. (you might want to download the file to look at while reading this)
Line1: Declares the on load handler. Lines 2-3: Moves the new bullet to the tower Line 4: Sets the variable ‘speed’ to 6. Change this to change how fast the bullet will move. Lines 5-9: So in the distance formula you remember x1, y1 ,x2 and y2? Now we define these as the x and y locations of the mouse and the tower so we can launch a projectile between the two points. Line 10: Calls the function distance to find the distance of the target. Line 11: Sets ‘changex’ to the difference of the x locations between the bullet and tower Line 12: Sets ‘changey’ to the difference of the y locations between the bullet and tower Line 13: Sets ‘clicks’ to the distance divided by speed. So pretty much we find the rate (Distance = rate * time) or the number of times the bullet is going to move to arrive at its destination. Line 14: Now we set ‘movex’ (How much x is going to change each frame) to changex/clicks. This is how much x is going to change during 1 "click" or move forward. Multiply by -1, don’t worry about this. Line 15: Now we set ‘movey’ (How much y is going to change each frame) to changey/clicks. This is how much y is going to change during 1 "click" or move forward. Multiply by -1, don’t worry about this. Lines 16-20: The distance formula in function form. Lines 22-25: Each frame the bullet moves ‘movex’ on the x axis and moves ‘movey’ on the y axis.
Thats it for the advanced code!
Place
markup_root.i=0;
on the first frame to initialize the creation of the bullets, and you’re done!
Actionscript Rotation
I just don’t have it in me to put you through another math lecture. I thought for sure that the distance formula would go by easy, but it kinda burned me out. So heres some free code :)
rotateTowardsMouse();
_global.radiansToDegrees = function(radians) {
return (radians/Math.PI)*180;
};
MovieClip.prototype.rotateTowardsMouse = function() {
var point = {x:this._x, y:this._y};
this._parent.localToGlobal(point);
var deltaX = _root._xmouse-point.x;
var deltaY = _root._ymouse-point.y;
var rotationRadian = Math.atan2(deltaY, deltaX);
var rotationAngle = radiansToDegrees(rotationRadian);
this._rotation = rotationAngle+90;
};
}
Put that on any movieclip and it will rotate to the mouse. Enjoy :)
Download If you want to download the examples visit the url below: download
Conclusion
Hope you learned something by reading this. Please leave a comment :D
~DigitalFire