Perl For Noobs
Perl For Noobs
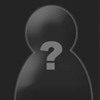
- Contents
- 1. Introduction
- 2. Comments
- 3. Variables/Arrays
- 4. User Input
- 5. If Statements
- 6. Loops
- 7. Functions
- 8. A Program
- 9. Conclusion
-Perl for n00bs written by CrashOverron-
##################################
Contents
1. Introduction
2. Comments
3. Variables/Arrays
4. User Input
5. If Statements
6. Loops
7. Functions
8. A Program
9. Conclusion
#################################
-
Introduction Hello everyone! This is my Perl article, it is intended for people just starting out in Perl, which is a very easy language to learn and quite often used in the hacking world to write exploits. Perl is an interpreted language, so in order to run Perl programs you must download an interpreter. I suggest Active Perl, simply because thats what I use, but any work I suppose. Anyways on with the show.
-
Comments A comment is something that programmers put in their code to explain typically what is going on, it will not get executed by when ran. In Perl a comment is done by placing # before text.
#This would be a comment
- Variables/Arrays Variables are data in your code, such as a name, number, or anything you can think of basically. Variables are represented in Perl by a $, an example of a variable would be
$name = "CrashOverron";
all this line is doing is declaring the variable "name" and setting the value to "CrashOverron". Also you notice the ; at the end. This is to tell the Perl interpreter that this is the end of the line and to proceed to the next command.
Arrays are simply a line of variables saved together. These are represented in Perl by a @ an example would be
@names = ( "CrashOverron", "KillerGuppy101", "BassBoy102" );
Arrays are zero-based index, meaning in order to output "CrashOverron" on screen our line of code would be
print $names[0];
This line prints "CrashOverron" on screen since its first on the list in the array its array placement is 0 and not 1.
- User Input User input is recognized by Perl by <STDIN>. In the code a variable is declared then the input code is placed after, so the input is saved into the variable such as
$name = <STDIN>; chomp($name);
we use chop() to get rid of the automatic newline created from the user input
- If Statements If statements are used to test variables with other variables or values if the comparison is false then the program skips the "if" code and goes to the "else" code. Variables can be compared by using operators such as
== equals != not equal
greater than < less than = greater than or equal to <= less than or equal to && and || or
an example of an if statement would be
if($variable == 1) { #some code } else { #some other code }
the && and || are used to create more complex if statements, like if you want something to happen only if two values are correct instead of just one, such as
if($variable1 == 1 && $variable2 == 4) { #some code } else { #some code }
- Loops Loops are used if the programmer wants to execute a code a specific amount of times. There are for loops, while loops, and foreach loops. For loops are formated like so
for(variable, statement, variable increment) { #code }
and example
for($i = 0; $i < 10, $i++){ print $i; }
this for loop sets the variable $i to zero and will go through as long as $i is less than 10, each time through it prints the value of $i. the $i++ adds 1 to $i after each pass through the loop, you could also use $i– to decrease $i by 1.
While loops are formatted much differently then for loops. instead of declaring a variable in the statement, it simply needs a statement, so a while loops could look similar to
$variable = 0; while($variable < 10) { print $variable; $variable++; }
this loops does the exact same thing as the previous for loop except done using a while loop.
Foreach loops are used to loop through arrays. A foreach example would be
@names = ("CrashOverron, "KillerGuppy101", "BassBoy102"); foreach (@names) { print $_; }
this loop simply loops through the entire array of names and outputs each value; the print $_ outputs the variable that is caused by the foreach from @names
- Functions Functions are very useful in programming. They are used if you have code you wish to use multiple times within your program. In perl, functions are declared by using "sub". Here is a small example program to demonstrate the use of a function and how to call it.
#!/usr/bin/perl #the above line is used to tell the interpreter this is a perl program
#begin function sub function { $name = "CrashOverron"; #create name and set to CrashOverron print $name; #print $name to screen } #end function
function(); #call function
the example function simply creates name and sets its value to "CrashOverron" and then prints it, it is then called later in the program.
- A Program Ok, now using everything, or nearly everything, that you have hopefully learned; lets create a small Perl program.
#!/usr/bin/perl #tells interpreter its a perl script
print "Name: "; #print "name: " onto the screen $name = <STDIN>; #ask for user input print "Password: " #prints "password: " $passwd = <STDIN>; #asks for user input if(passwd == "crashiscool"){ correct(); #call correct function } else { print "$passwd is not the correct password"; }
#start function sub correct { @users = ("CrashOverron", "KillerGuppy101", "BassBoy102"); #declare array users
#begin loop
for($i = 0, $i < 3; $i++) {
print \"User $i: $users[$i]\"; #print each user from users array
}
#end loop
} #end function #end code
- Conclusion Well I hope you learned something while reading this article, and I am sorry if some of it doesn't make sense it's 4am so im a little tired lol but if you have any trouble or need help with any of it just PM me. I will possibly write a more advanced Perl article in the near future depending on how you all like this one. Please vote for the article as you see fit and also come check out some of my programming projects at http://crashoverron.t35.com. well thanks for reading my article peace and g'night
CrashOverron
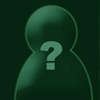
ghost 16 years ago
Thanks i am going to learn perl and this helped me get a few basic things in my head before i start. Cheers:)
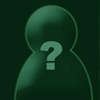
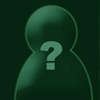
Dystopia 15 years ago
Thanks for this. Gives a clear overview of the basics. Has definitely given me a good start with Perl.
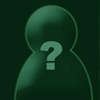
Dystopia 15 years ago
Thanks for this. Gives a clear overview of the basics. Has definitely given me a good start with Perl.
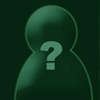