Python lesson 5: Modules
Python lesson 5: Modules
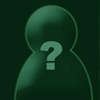
Python Lesson 5: Modules
Python uses a system of inclusion to handle things not builtin to the program. In Python these extras are called modules, in Java, they are beans, in PHP and C they are libraries. Whatever they called they tend to function more or less the same way. In Python to set up references to a module is very easy.
import math
From here you can call any class or function from the math module (Worship THIS) with:
math.function() OR math.class
Obviously replaced by actual functions and classes. If you want to import them all locally and do away with the module. part:
from math import *
This brings them all into the local workspace, and speeds up the process, but consumes more memory, this is a decision you must make on a case by case basis, though for the majority of applications neither really affects performance.
Now to demonstrate the point I should do something with modules.
I'm actually going to write two programs in this article, one using imported functions, the other an imported class.
First up a guessing game using the random module:
#!/usr/bin/python import random
def guessing_game(number = 1): ## defining our procudure, using the number = clause to initaliase our var [tab]diff = input("What number shall i go up to?") [tab]number = random.randrange(1, diff) ## randrange is a part of the random module. it returns a number between it's arguments [tab]print "I have picked a number between 1 & %d." % (diff) [tab]print "Can you guess what it is?" [tab]guessed = "no" ## our counting vars… be careful of using variables with similar name… this was a very small script and i was [tab]guesses = 0 ## careful, plus one of them is only referenced like twice [tab]while guessed == "no": [tab][tab]guesses = guesses + 1 [tab][tab]guess = input("What is your guess?") [tab][tab]if guess > number: [tab][tab][tab]print "Too high." [tab][tab]elif guess < number: [tab][tab][tab]print "Too low." [tab][tab]else: [tab][tab][tab]print "You got it!" [tab][tab][tab]guessed = "yes" [tab]print "You took %d tries to guess my number." % (guesses) [tab]tryagain = raw_input("Do you want to try again?(y or n)") [tab]if 'y' in tryagain.lower(): ## this means that 'yes', 'Y', and all sorts of other replies will return true [tab][tab]guessing_game() ## recursively calling a function is ugly, but if it's the last instruction, it's excusable. [tab]
guessing_game()
So there you have it, well, actually only one call to the module, which is an example of a time where importing * is a waste of memory. If i was determined to import something to local, i could have done:
from random import randrange
But still, really a waste of memory. Now, an imported class. For those of you not aware a class is an object, the strings, lists, tuples etc you've been using are all classes. The next article (or the one after) will go into much more detail about using classes and creating your own.
So, to demonstrate a class I'm going to write a simple md5 hasher.
#!/usr/bin/python
import md5
plainText = raw_input("text to hash?") hasher = md5.new(plainText) ## the new class in the md5 module returns an object that can store data and return the hash cryptText = hasher.digest() ## the digest method (note that it's called on what's called our "instance" of the class, returns the hash of the contents hasher = raw_input("The md5 hash of %s is %s." % (plainText, cryptText)) ## essentially a press return to exit message.
Very simple script. .. but very effective. The next article will be either relating to classes or the urllib module… probably classes.
If there's anything that doesn't make sense to you, then there are others who won't get it either. hit me up and I'll clarify it here.
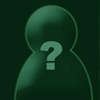
ghost 18 years ago
You can also import one specific thing from a module. This improves performance without the memory hogging. Eg. from random import randrange randrange(1,10)
Wicked article though. And I wanna see somebody touch on the urllib module. Classes are boring. :D
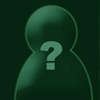
richohealey 18 years ago
So there you have it, well, actually only one call to the module, which is an example of a time where importing * is a waste of memory. If i was determined to import something to local, i could have done:
from random import randrange
uhhh i know.
and i said at the end that i was touching on the urllib module……
Classes rule man…. OOP roxz0rz my s0xz0rz
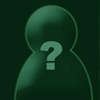
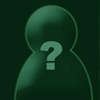
richohealey 17 years ago
Python docs. you should sleep with them under your pillow. and i'm releasing regexes tonight :D
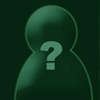