Python lesson 4: File Handling
Python lesson 4: File Handling
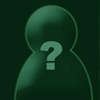
Python lesson 4: File Handling
Ok guys! Back into it. This article is a follow on from Lesson 3. If you're only here to learn how to play with files it'll all make sense, but the project at the bottom of the article is based heavily upon the last one.
Files: When you open a file it attributes a "File-Like Object" to your variable. There are other things that have this property, some kinds of sockets, assorted other objects. It's also a common standard for writing your own objects.
So on with it. To start you need to create your object. myFile = open(path, mode)
path is rather self-explanatory. It follows all rules for the platform you're on. If you want to write multi-platform code that uses files across multiple directories, you'll need to tune in to my article on the os module, or do some research on http://www.python.org/doc/. Otherwise just use files in the same directory.
mode determines the way in which it opens the file. if left blank it defaults to read, which puts the iterator at the start of the file. The other modes are r, w, and a. These are not variables they are values, and so must be used in quotes.
so: 'r' : Puts the iterator at the start of the file, that is if you try to read teh next line you will get the first. Will throw an error if the file you have tried to open doesn't exist. 'w' : Opens the file for writing. If the file already exists, it doesn't now. Overwrites existing contents. Throws errors if you try to read from it. 'a' : Opens the file into a writable state. But leaves all existing contents. Puts the iterator at the end, so writing to it is possible. If the file doesn't exist it creates it.
Now the methods that apply TO file like objects.
Again, this is taken from http://docs.python.org/lib/bltin-file-objects.html
close() : I can't state this clearly enough. You NEED to have this in your programs, and implemented in such a way that it WILL run. In most cases if the program crashes, the OS can work itself out, but it's stupedously bad form to leave it up to the OS.
read(x) : Read x bytes from the file, including newline chars. You can leave x out, and it will return the entire contents of the file as a string.
readline() : Return the current line that is pointed to by the iterator.
write(data) : When the object is open for writing, appends the data to the end of the file. You'll need to put in your own endlines. \r, \n, or \n\r depending on OS. Python supports 'em all, so it's just a matter of how they'll show up in text editors.
newlines : This is a value, not a method, but appears when –with-universal-newlines is used to build Python, (don't worry! that's the default). the newlines value has either a single value, or a list of values representing all the newline characters it has seen in that file. It can be an excellent time and effort saver, rather than importing os for endlines.
Ok! Today's project. I'll be building on the phonebook from last time, adding two new options, Write to file, and Read from file. If you were very quick you may have noticed that the code from lesson 3 had a typo, it had "Type a number (1-7):" as the prompt, I was planning to put that in. I won't add comments for the code that is identical to last time.
#!/usr/lib/python
def print_menu():
[tab]print '1. Print Phone Numbers'
[tab]print '2. Add a Phone Number'
[tab]print '3. Remove a Phone Number'
[tab]print '4. Lookup a Phone Number'
[tab]print '5. Write Phone Book to File' ##two new entries!
[tab]print '6. Read Phone Book From File'
[tab]print '7. Quit'
numbers = {}
menu_choice = 0
print_menu()
while menu_choice != 7:
[tab]menu_choice = input("Type a number (1-7):")
[tab]if menu_choice == 1:
[tab][tab]print "Telephone Numbers:"
[tab][tab]for x in numbers.keys():
[tab][tab][tab]print "Name: ",x," \tNumber: ",numbers[x],
[tab][tab]print
[tab]elif menu_choice == 2:
[tab][tab]print "Add name and number"
[tab][tab]name = raw_input("Name:")
[tab][tab]phone = raw_input("Number:")
[tab][tab]numbers[name] = phone
[tab]elif menu_choice == 3:
[tab][tab]print "Remove Name and Number"
[tab][tab]name = raw_input("Name:")
[tab][tab]if numbers.has_key(name):
[tab][tab][tab]del numbers[name]
[tab][tab]else:
[tab][tab][tab]print name, "was not found"
[tab]elif menu_choice == 4:
[tab][tab]print "Lookup Number"
[tab][tab]name = raw_input("Name:")
[tab][tab]if numbers.has_key(name):
[tab][tab][tab]print "The Number is",numbers[name]
[tab][tab]else:
[tab][tab][tab]print name,"was not found"
[tab]elif menu_choice == 5:
[tab][tab]phone_book = open('phone_book.txt', 'w') ## open the phone book to write. it may be an existing one, and we don't want to add a nearly identical entry to the end of an old one
[tab][tab]print "Write Phone Book to File"
[tab][tab]phone_book.write("Name: \t Number: \n") ## add an entry to it so if someone opens it it's readable
[tab][tab]for x in numbers.keys(): ## for each name in the book
[tab][tab][tab]#string = "Name: ",x," \tNumber: ",numbers[x] ## this is a comment by me. it's so i can remember what i'm writing to the files
[tab][tab][tab]phone_book.write(x) ## you can write over as many statements as you like.
[tab][tab][tab]phone_book.write(" ") ## so this is a much more orderly way of doing it
[tab][tab][tab]phone_book.write(numbers[x].replace(" ", "_") ## change spaces to 's for storage
[tab][tab][tab]phone_book.write("\n")
[tab][tab]phone_book.close() ## always close your damn files!
[tab]elif menu_choice == 6:
[tab][tab]try: ## try except clause. Will be covered soon in a error handling article
[tab][tab][tab]print "Read Phone Book From File:"
[tab][tab][tab]phone_book = open('phone_book.txt', 'r') ## opening it with mode read
[tab][tab][tab]if phone_book != "": ## if the file is not empty. This is the statement that will throw the error if it does
[tab][tab][tab][tab]list = phone_book.readline() ## this is actually just to remove the index line we added earlier
[tab][tab][tab][tab]for line in phone_book:
[tab][tab][tab][tab][tab]myList = line.split(" ") ## seperate it into a list with 2 entries
[tab][tab][tab][tab][tab]numbers[myList[0]] = myList[1].replace("", " ") ## rewrite it into the list with spaces instead of underscores
[tab][tab][tab][tab]phone_book.close()
[tab][tab]except:
[tab][tab] print "Phone book file does not exist!" ## Yell at the user for a bits
[tab]elif menu_choice != 7:
[tab][tab]print_menu()
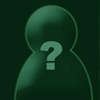
regret 16 years ago
Another nice article Rico. I've been using Perl alot here lately, but because of your articles I am beginning to assimilate some of your Python knowledge. Much appreciated…
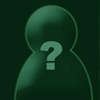
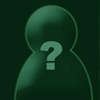
ghost 16 years ago
great stuff, keep pumpin' em out. what's next on the list menu? "Performance Analysis Between Python and C code"? lol, I'll be glad to read something about integrating Python with lower level languages to produce some really advanced scripts and programs.
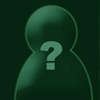
richohealey 16 years ago
ha ha netfish, yes there is an article about integrating lower level languages, though that's a bit later on. i'm avtually trying to think of some interemediate topics to cover. i have an article on sockets, and one on GUI's nearly ready for release. I'm just polishing, and building up with some other stuff. i might release one on OO next…. hmm we'll see.
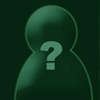
bahpomet1105 8 years ago
nice article richohealey. Maybe would you like to write some python programs together.
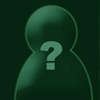
bahpomet1105 8 years ago
Python was my second language but I has taken over my life I use it for everything. From raspberry pi to and fuzzying protocols it amazing.