Python lesson 3: (More) Data Structures
Python lesson 3: (More) Data Structures
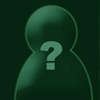
Python Lesson 3: (More) Data Structures.
Last time I discussed list and tuples (known as arrays in other languages), and strings.
Lists and tuples come under the heading of indexed arrays, in that the only reference to an object in it is it's position in the list. An associative array in contrast has a 'key' by which the other objects are known. This is known in Python as a dictionary. They are defined thus: myDict = {} OR myDict = dict()
To add values to a dictionary, just assign it as you would a list: myDict['key'] = "value"
To create a dictionary with some preexisting values: userPass = {'Richo' : 'richo\'s pass', "some key": "some value"}
There are a few dict methods you will need at first (this is an extract of http://docs.python.org/lib/typesmapping.html) len(a) the number of items in a del a[k] remove a[k] from a a.items() a copy of a's list of (key, value) pairs a.keys() a copy of a's list of keys a.values() a copy of a's list of values a.get(k) a safe way to do a[k], won't throw exceptions if k not in a
So, onto the project for today. We're coding a phone book manager. It doesn't have support for saving to file (tune in later tonight for that one!) but it does have some rather spiffy dictionary usage. I'd like to add here that some things I've done in a different way to last time. One of Python's key strengths is the way that there's always 2 ways to achieve something (and generally heaps more!).
#!/usr/lib/python def print_menu(): ## again, putting the menu in a function [tab]print '1. Print Phone Numbers' [tab]print '2. Add a Phone Number' [tab]print '3. Remove a Phone Number' [tab]print '4. Lookup a Phone Number' [tab]print '5. Quit' numbers = {} ## initiallise our phone book menu_choice = 0 print_menu() while menu_choice != 5: [tab]menu_choice = input("Type a number (1-5):") [tab]if menu_choice == 1: [tab][tab]print "Telephone Numbers:" [tab][tab]for x in numbers.keys(): ## in our book, the keys will be representative of names [tab][tab][tab]print "Name: %s \tNumber: %s" % (x, numbers[x]) ## using % again, also \t is a tab, so they'll line up [tab][tab]print ## whitespace == neat presentation [tab]elif menu_choice == 2: [tab][tab]print "Add name and number" [tab][tab]name = raw_input("Name:") [tab][tab]phone = raw_input("Number:") ## we'll use raw_input for the number as it may have a +61 prefix or something [tab][tab]numbers[name] = phone [tab]elif menu_choice == 3: [tab][tab]print "Remove Name and Number" [tab][tab]name = raw_input("Name:") [tab][tab]if numbers.has_key(name): ## simple test to be sure we don't throw any exceptions [tab][tab][tab]del numbers[name] [tab][tab]else: [tab][tab][tab]print name, "was not found" [tab]elif menu_choice == 4: [tab][tab]print "Lookup Number" ## here is where i might add to this project in the regex section [tab][tab]name = raw_input("Name:") [tab][tab]if numbers.has_key(name): [tab][tab][tab]print "The Number is",numbers[name] [tab][tab]else: [tab][tab][tab]print name,"was not found" [tab]elif menu_choice != 5: ## if the choice isn't 1-5 print the menu, else continue and exit [tab][tab]print_menu() test = raw_input("Press return to quit")
So there you have it. The comments are actually seeming rather lacking, hit me up in the comments if you need more I'm happy to go back over them. I think this project is one I'll keep using in these articles, adding database integration, file support, regex searching for users etc.
Peace.
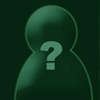
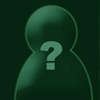