Python lesson 2: Data Structures
Python lesson 2: Data Structures
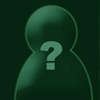
Data Structures.
Ok, I'm guessing that if you're reading this then you've either read my first article or have some Python background. If not go read my first one here
Now as I mentioned these articles are project based. In the next 2 articles (And then in a 3rd one ages from now) we will write a simple phone book handler, in this encarnation it won't have a save function. The next lesson will cover file handling, and then finally I will detail database integration in about article #10. In fact if i don't come up with something better i might build on this for the GUI article as well. That said, let's get coding.
In Python there are datatype that you need to worry about for the time being.
Strings: Can be defined many ways: "This is a string" : Used for strings that you plan to use the % () variable handling method. Think of them like interpreted strings.
'This is a string' : Used to "stupidly" read the contents. The only special character it sees is the \ (backslash). I will sometimes call them stupid quotes.
"""This is a string""": Triple quoted strings can have newlines in them. Good for long strings that will be printed. Also if oyu define a function you can put a triple quoted string as the first line, and when working in IDLE, that string will come up as a tooltip when you type the first ( after the function name. Example: def add(var1, var2): """add(var1, var2); returns var1 + var2 var3 = var1+var2 return var3
For now that's all the string types you'll need.
Now i mentioned the % method.
If you have a statement that goes:
print "hi, my name is " + name + ". I am " + age + " years old. I'm glad to meet you " + yourName + "." This works but is messy and hard to read, especially without highlighting. A much better alternative to use: print "hi my name is %s. I am %d years old. I'm glad to meet you %s." % (name, age, yourName)
Now this is simple as you can see. You must be very careful to use the right variable type though, or you will get exceptions left right and center. The types are: d | i Signed integer decimal o Unsigned octal u Unsigned decimal F | f Floating point decimal format r String (converts any python object using repr()) s String (converts any python object using str()) % No argument is converted, results in a "%" character in the result.
That was grabbed and stripped down from http://docs.python.org/lib/typesseq-strings.html. Go there for the full list.
Next data type: Lists and Tuples.
I'm grouping them together because of the huge similarities.
The key difference is that a list is mutable (changable) whereas a tuple is immutable (unchangable).
A list is defined thus: myList = list('richo', 7, "secretpassword") OR myList = ['richo', 7, "secretpassword"]
sililarly a tuple is defined: myTuple = tuple('domeguy', 26, "his password") OR myTuple = ('domeguy', 26, "his password")
from here you can call an object by it's index myList[1] == 7 myTuple[2] == "his password"
negative values work backwards myList[-1] == "secretpassword"
and the colon gives you a from:to myList[:2] == ['richo', 7] myList[1:] == [7, "secretpassword"]
keep in mind that indexes begin at 0. Now the key difference is that myList[0] = "richohealey" works, whereas myTuple[0] = "that dude" throws an exception.
I'll run through some common list methods, and put a ** at the start if they are non tuple. As they are methods they are called thus list = ['Richo', 'Healey'] list.append('is fucking awesome')
**append([objects]) Tacks objects, (can be any data type) onto the end of lists. If you want to do this with a tuple you need myTuple = myTuple + ('some', 'more' 'stuff')
**pop(index) returns the object at [index] and removes it from the list.
index(value) returns the index of the first occurence of value in list. best called after an if in clause: if value in list: index = list.index(value)
count(value) returns the number of value's in list
**remove(value) removes the first instance of value
del list[x] not technically a method, but deletes the entry at index x
Now the project. It WAS going to be a phone list, but this article is already longer than I expected, so that'll be in the next installment, that I'll probably still write tonight.
Instead we'll be doing a shopping list program that will only add an item if it's not already on the list.
#!/usr/lib/python shoppingList = [] ##initialise our shopping list menu_list = """1) Add an item 2) Check an item 3) Display current list 4) Remove an item 5) Start a fresh 6) Go shopping (quit) """ ## define our menu def menu(): ## create a function for out menu to clean up the main loop a bit [tab]"""Displays a menu and takes a choice""" [tab]print menu_list [tab]return input("Choice?") def print_so_far(): ## since this procedure is called in more than one place, ## we Create a function for it [tab]print ## whack in some white space for neatness [tab]print "The list thus far" [tab]for i in shoppingList: ## iterate through the list, printing each item on it's own line [tab][tab]print i
while 1: ## run until stopped [tab]print ## whitespace [tab]choice = 7 ## create a variable, and give it a value that won't bypass the loop [tab]while choice > 6: ## keep demanding a choice till they make a good one [tab][tab]choice = menu() [tab]if choice == 1: ## each of the if/elif statements is obviously testing their input [tab][tab]item = raw_input("Add what item?") [tab][tab]if item not in shoppingList: ## check to see if it's already on the list [tab][tab][tab]shoppingList.append(item) ## if it's not, add it to the end [tab]elif choice == 2: [tab][tab]item = raw_input("Check what item?") [tab][tab]if item in shoppingList: ## check it exists [tab][tab][tab]print "%s is on the list" % (item) [tab][tab]else: [tab][tab][tab]print "%s is not on the list" % (item) [tab]elif choice == 3: [tab][tab]print_so_far() [tab]elif choice == 4: [tab][tab]item = raw_input("Remove which item?") [tab][tab]if item in shoppingList: # test existence first to avoid exceptions, it's nearly always # better to avoid than catch [tab][tab][tab]shoppingList.remove(item) [tab][tab]else: [tab][tab][tab]print "Hey! %s isn't even on the list!" % (item) [tab]elif choice == 5: [tab][tab]shoppingList = [] ## hose the old list [tab]elif choice == 6: [tab][tab]print_so_far() [tab][tab]raw_input('Press return to quit') ## show them the list, and wait for confirmation before quitting [tab][tab]break
This script's man downfall is that non-numeric values will throw errors at the menu choice, but exception handling is far beyond the scope of this article.
Sources: http://docs.python.org/lib/
Thanks for the read, and stay tuned for more articles soon!!
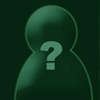
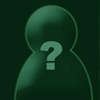
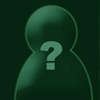
ghost 17 years ago
I love it! I didn't know how to do data structures in Python, only in C++ :D
*remind me to buy you a beer ;) *
–Skunkfoot
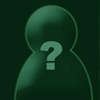
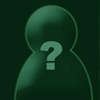
Flaming_figures 17 years ago
You know, I started wanting to get into python but couldn't find any solid tutorials that were easy t understand, good examples, and I kept thinking "Damn ricohealey needs to make a bunch of articles on python.. why hasn't he!" and then this. thanks man… my prayers (litterally) answered.
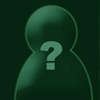