Python lesson 1: Basic Intro
Python lesson 1: Basic Intro
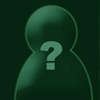
- ^^ That is the only line that won't be commented on it's own line.
- Under *nix the first line of an executable script contains the
- path to the interpreter. Type which python at command line to get
- the path to your Python interpreter.
Basic Intro To Python.
First of all, this is not going to be the definitive beginners guide. There are already plenty of good ones, so I'm not going to recap. Instead I'm going to set the trend for this series of articles. Each article will work towards a project. Many of the projects will lead on from one another. Where this is the case I will tell you at the end of the the former and the begginning of the latter that there will be/was another part.
In this article I am first going to dish out some links. This article by wolfmankurd is fantastic. Bookmark it. Now.
Next up is the Python documentation Here. You need to have this bookmarked. Preferably a printed copy, but obviously you should probably wait until you decide that Python is for you.
Now our project:
Lesson 1: Input/Output.
In Python using input and output is legendarily simple. In fact our first project will be only a few lines. We will create a passthrough script to send commands back to the shell. This will involve an imported module. Please bear with me and trust me that i will go into greater depth about modules when I release the article on classes and object oriented programming.
The most common way to get input is the input functions, raw_input() and input(). The difference being that input() returns an integer or float, and only accepts int and float types. Inputting other values will raise an exception. raw_input() returns a string, and can take any type of input. Both of these functions can take a single argument, prompt, optionally. If set the prompt variable (surpisingly) prompts the user.
Now, the only thing that you will need for this lesson that's not covered in wolfmankurd's article is defining functions. This is characteristically simple.The syntax is: def function(args): [tab]Code [tab]Code
and that's it. your function can be named anything (but make sure you don't go ever stuff that's builtin/predefined!). If you put an argument in brackets ([]) it is optional. You can also put a varialbe with a = clause, which causes it to have a default value.
You could use this attribute for a file reader that defaults to read, in two different ways def file_open(path, [mode]): [tab]if mode: [tab][tab]return open(path, mode) [tab]else: [tab][tab]return open(path, 'r')
You could also save yourself some space by having: def file_open(path, mode='r'): [tab]return open(path, mode)
Next up: Loops. There are two loop types, for and while. their syntax is very simple, there are tips and tricks to them that will be in a later dedicated article. but simply, they are; for i in (iterators): [tab][do stuff]
and while (condition): [tab][do stuff]
If you include a break statement in a loop, it will "break" out when called, it exits that loop and goes back to the indentation level of what called it.
Now our code. Each line will be commented. This program simply passes stuff back to the shell, unfortunately returning that output is beyond the scope of this article, so only commands like "pause" (windows) will have effect. It IS however a good way to spawn Shells (CMD) where you are locked out ;)
#!/usr/bin/python
^^ That is the only line that won't be commented on it's own line.
Under *nix the first line of an executable script contains the
path to the interpreter. Type which python at command line to get
the path to your Python interpreter.
import os ## This imports the os module which contains funtions and ## classes relating to the shell and filesystem.
def exec_cmd(command): ## We're defining a function. as you can see it takes one argument. [tab]os.system(command) ## os.system() just passes it's argument back to the instance that executed your script
while 1: ## While 1: goes forever (or until broken) [tab]user_input = raw_input("Command?") ## take some user input [tab]if user_input == "exit": ## if that input is the word "exit" [tab][tab]break ## break out [tab]exec_cnd(user_input) ## otherwise execute the command
And that's out first script!
This is the first in the series, it will be updated. Please comment me, email me with any issues with this article. Also feel free to PM/email me with general Python stuff.
[Edit]Oh, and feel free to suggest topics for more articles, I have another 5 planned, but I'm always keen to do more![Edit]
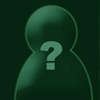
ghost 18 years ago
Nice article. It does a good job of introducing the basics, and demonstrates what good code looks like. It's important to build good code habits right from the start.
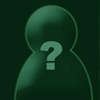
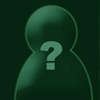
ghost 18 years ago
I like that you're writing python articles :)
I can't wait til you teach me something I don't already know :D
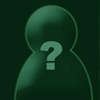
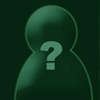
richohealey 18 years ago
some data structures stuff for art 2.
then some file handling.. witth more than just open() and x.write()
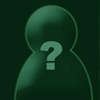
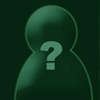
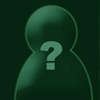
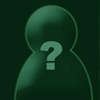
bahpomet1105 9 years ago
Awesome article I am reading all of them but I am currently reading Python by o'rielly.