C++ Basics for the absolute Newb
C++ Basics for the absolute Newb
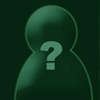
C++ Basics for the absolute Newb By. RockBll4
This tutorial is aimed at people running Microsoft Windows. The system("pause"); function is for MS not for linux or other OS's.
**Comment and rate the article after you read it please**
This is a tutorial for people that are absolute newbs to programming and want to learn C++, I will do as much hand holding as possible and try to explain every part of what we do, and what each part does.
Ok, in this tutorial I will explain most of the basics of the C++ Language. C++ is a superset of the C Language.
C++ is an object-oriented programming language. It is a very low level programming language that produces very fast programs.
C++ is very portable, which means that almost all operating systems support the language.
Ok now, to start programming you will need 2 things, a text editor (such as Notepad), and a compiler. You will use the text editor to write your programs' code, and the compiler to compile it. Some compilers, such as Bloodshed Dev C++, will have a text editor built into it. What a compiler does is converts your C++ code into a language the computer understands (1's and 0's).
In a C++ program you have what is called header files. These are the things that load all the necessary functions you need to run your program. You must include your header files at the beginning of your programs code. Headers begin with the pound symbol (#), followed by the word: include, then the less-than symbol (<), then the name of the header file, and then finally you end it by including a greater-than symbol (>).
Example:
#include<iostream>
The next line of code that we will use is called a namespace. All parts of the C++ library are called within a namespace. The namespace we will be using is called: std. To do this you must first type: using, then type: namespace, and then finally you type the name of the namespace you will be using: std, and don’t forget that you must include a semi-colon which tells the program that you ended the line of code.
Example:
using namespace std;
Next we must begin the main part of our program where we will put all of the code we want to program to execute. Its whats called the main function. It begins as you would begin any other function, with the declaration of what type of function you want it to be (be it an int, float, char etc). To start the main function you need to declare it an int type. So you first type int, then you need to type the name, which is none other then: main, then you include two parenthesis ().
Example:
int main()
After that we start a pair of curly braces. What the braces do is trap code within discreet areas. The processor knows that that’s the code that needs to be executed and therefore will execute the code within the braces.
Example:
{
What comes after the first curly brace is the code that you want your program to execute within the main function. OR it contains mainly all the code of your program. I'm not really going to put anything in here right now, later I'll give you some more examples on what goes in here. But for now we will be inputting the code that ends your program. For starters we will be using the system() function. If you have a basic knowledge of DOS you will know what the pause command does. The pause command will pause your program so you can see the result of what the code did after being executed. OR it was make it so you have to Press Any Key to Continue, after it executes. So to do this we need to start the system function: system(, then type the pause command: pause, then end the parenthesis, and finally include the semi-colon which tells the program you ended the line of code.
Example:
system("pause");
Next you need to end the main function, by returning a value. In this example we will return the integer 0. This makes the program think that it executed successfully and will thus end the program. To do this we use the return function: return, then we type what we want it to return: 0, and finally include the semi-colon which tells the program you ended the line of code.
Example:
return 0;
Next you must end the code block by ending the pair of curly braces. By typing the last curly brace in the program you truly end the program.
Example:
}
The full program example will look like:
#include<iostream>
using namespace std;
int main()
{
system("PAUSE");
return 0;
}
Ok, now that we have the basic layout of a program done, we are going to learn base input/output using C++. Which means that we are going to make text appear on the screen. For this we are going to be using the: cout statement, which is in the iostream header file library. So go ahead and type cout, space, and then you need two less-than signs (<<), space, then you need to include one set of double quotes. Then proceed to type the following words "Hello World", then end your set of quotes. Now we want it to enter down one line before we end the program, so we need two more less-than signs (<<) and type what I call the "end line" command. It looks like this: endl. So type that and do you remember how to tell your program your finished with this line of code? That’s right your type a semi-colon.
Example:
cout << "Hello World" << endl;
Ok what we just did was use the cout statement to display the words: "Hello World" on your computer screen. The endl statement does what it says: it ends the line. So it enters down so the next line of code will run one line down. The whole program should look like:
#include<iostream>
using namespace std;
int main()
{
cout << "Hello World" << endl;
system("PAUSE");
return 0;
}
Now compile and run it. It should've compiled successfully and opened up a console window with the words Hello World. If you got that, then congratulations! You have just written and compiled your first C++ program! ... Very exciting. Now you can just repeat everything that you just learned here so you can get it stuck in your memory and it will be just like tying your shoes.
All right, now that we have the basics out of the way, I'm going to introduce you to the wonderful world of variables. What variables do is store certain data types that you assign in your program into the computers memory, so you can retrieve them for later use. Take this for example:
Example:
x = 1
Here we store the value of 1 in the variable x. So if we need to get the value again later in the program we can simply refer to the variable the variable that it is stored in. Which is x.
To declare a variable you need to assign it a type. There are a number of different variable types. The variable depends on the type of value you want to store in the variable. I will give a few examples of the variable types:
Type Description
********~~~~~~~~~~~~~~~
int | Integer (number) ints don’t have quotes around them.
char | A Character (such as 'a') characters have single quotes ' '
bool | True or False
long | A long integer
double | Float-point variable
string | A string of characters
There is a description of some of the variable data types. Now to start declaring variables we first need to assign it a type, so in this example we will declare an integer. So type: int, then we need to name the variable, so we'll just call it x, lastly you need to end that line of code: ;.
Example:
int x;
To assign a value to a variable you can do it right after you declare it, by adding an equals sign (=), and then the value u want to assign (lets say 1), and of course end your line of code: ;.
Example:
int x=1;
Or you can declare the variable and then store a value later on. To do this you need to have the variable already declared and then whenever you want to assign a value, you just type the name of the variable and then the equals sign again (=), the value and of course your semi-colon.
Example:
int x;
x=1;
If you want to display the value stored in the variable, you have to use the cout statement, which we just learned, and you type the name of the variable (without quotes), after the two less-than signs. So start out by typing the cout statement, then the two less-than signs, then of course your variable name (without quotes), and we want it to enter down before it ends the program, so type two more less-than signs, and then of course the endl command, and your semi-colon
Full Example:
#include<iostream>
using namespace std;
int main()
{
int x;
x = 1;
cout << "The value of x is: " << x << endl;
system("PAUSE");
return 0;
}
Remember that you aren’t restricted to the int variable type. You can use char and store an 'a', remember the single quotes. Or You can store a string of characters using string.
I'm not going to go into too much detail with the mathematical operators. But you will use pretty much the same symbols you would use in 3rd grade math. Here is a short list of the operators that you can use:
Operator Description
*********~~~~~~~~~~~~~~
+ | Used for Addition
- | Used for Subtraction
/ | used for Division
* | Used for Multiplication
++ | Used for Incrementing
-- | Used for Decrementing
Like I said I’m not going to go into too much detail about these so I'll just show you some examples of how you can use these:
Example 1:
int x=1;
int y=2;
int result = x+y;
Example 2:
int x;
x = 3-1;
Example 3:
int x, y, result;
x = 3;
y = 2;
result = x*y;
Now just keep practicing and using these operators and find out the rest. You will need them later on in your programming career.
We are going to get on the subject of getting user input. For this we will be using the cin statement. The cin statement will allow the user to type some input and if u want to assign it to a variable it will let you do that. To use this you should have a variable ready to assign the input from the user to. So to start this you should declare yourself a variable, lets make it an int. Next you should return a couple times, and type the cin statement, after that you will use to greater-than symbols this time (>>), what they do is assign what the user inputs from the cin statement and assigns them to the variable that your about to type. So next you type the name of the variable that you declared and end your line of code with a semi-colon.
Example:
int x;
cin >> x;
In that example we just assigned x to what integer the user inputted. You can also have it display what the user typed on the screen using the cout statement. You should know how to do this by now.
Full Example:
#include<iostream>
using namespace std;
int main()
{
int x;
cout << "Type an integer: " << endl;
cin >> x;
cout << "You typed: " << x << endl;
system("PAUSE");
return 0;
}
You can combine all the things I just taught you into a small basic C++ program, that will get user input and store them in a variable, then displaying what the user typed back to them.
Example:
#include<iostream>
using namespace std;
int main()
{
int input1;
int input2;
int result;
cout << "Hello, why don’t you input an integer?" << endl;
cin >> input1;
cout << "And another..." << endl;
cin >> input2;
result = input1+input2;
cout << "The result of " << input1 << "+" << input2 << "=" << result << "." << endl;
system("PAUSE");
return 0;
}
Now that you have learned the basics of C++, I'm going to let you go and embark further into the wonderful world of the C++ programming language. If you liked this tutorial, please leave me some feedback
LMAO seriously how hard is it to atleast rate each article you read? i have over 640 reads and 4 rates… plz at least rate my article :-D
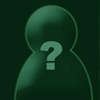
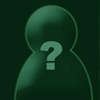
ghost 18 years ago
C++ is not a very low-level language, in fact it is the complete opposite, it is a very high-level language. The article is alright, but just a few side-notes for you. :) The double greater-than(>>) symbol is known as the extraction operator and the double less-than(<<) symbol is known as the insertion operator. Although C++ is very portable, some commands aren't ( e.g. system("PAUSE") ). Good job.
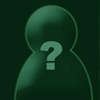
ghost 17 years ago
Great i have been trying to get into C++ but i haven't done it this article inspired me into getting alot more into it thanks for this! :)