Writing Games in Flash
Writing Games in Flash
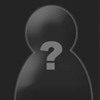
[Edit] Note : at the time of publishing, AS 3 had not yet been released. This tutorial assumes AS 2 [/Edit]
Introduction Welcome to my tutorial on writing games in Flash.
Flash is a great medium for creating games for several reasons: *Flash runs on every OS *Flash runs very well on the Web *Flash is fairly easy to learn
Rather than creating a single game, in this tutorial I will show you a bag of tricks that you will be able to apply to all games you write.
What you need: *Macromedia Flash (MX or 8 work best) *Basic knowledge of the Flash interface, symbols and actionscript syntax. When I say ‘movieclip’, ‘stage’ and ‘symbol’ you should know what I’m referring to. *Knowlege of programming
What you will learn: *Keyboard Movement *Collision Detection *Movieclip Duplication
Alright, lets jump right in.
KEYBOARD MOVEMENT
What we will be making:
Keyboard_Movement.swf (Make sure you click on the movie to select it before pressing the keys)
Using the keyboard to move a character or object across the screen can be very useful in writing games. Luckily, flash makes this extremely easy.
Create a new symbol (movieclip) and paste this code into it:
if (Key.isDown(Key.RIGHT)) {
this._x += 3;
}
if (Key.isDown(Key.LEFT)) {
this._x -= 3;
}
if (Key.isDown(Key.UP)) {
this._y -= 3;
}
if (Key.isDown(Key.DOWN)) {
this._y += 3;
}
}```
And viola, the movieclip moves when we press the arrow keys!
The how:
On each frame this moveclip will look at the state of each arrow key individually, and if any of them are being pressed down it will respond by moving the moving clip.
The code:
Line 1 tells flash to run the block of code between the { and } on every frame (enterFrame).
Line 2 checks to see if the RIGHT key is being pressed.
Line 3: If it is, it changes the ._x (x coordinate) of 'this', which is the movieclip, so the movieclip moves to the right. It moves by 3 pixels (each Frame).
The rest: Repeats the above lines for all arrow keys and moves accordingly.
There are other methods of detecting key presses such as the 'On (keyPress)' method, but the method used above allows for smoother and diagonal movements.
**COLLISION DETECTION**
What we will be making:
[Collision_Detection.swf](http://s153.photobucket.com/albums/s216/digital_fire_tutorial/collision_detection.swf)
Being able to detect if two objects are touching is critical to writing games. Did the bullet hit the player? Did the bullet hit the wall? Is the player up against a wall that he can't move through?
Flash provides a useful function for detecting collisions, called the hitTest. hitTest comes in two flavors: Bounding Box and Shape Flag. We will focus on the bounding box version in this tutorial, which only detects if the bounding boxes around two shapes are touching. If you need more precision than this, shape flag is what you will be looking for, but is left out of this tutorial because it is a little more complicated. Google will help you find tuts on that ;)
Bounding box checks to see if the bounding box around an object touches the bounding box around another object. It takes in the instance names of two objects and returns true if they are touching and false if they are not.
An example of a hitTest:
```markup if (_root.object1.hitTest(_root.object2)==true){
//execute code if they are touching
} else {
//execute code if they are not touching
}```
The code used in the Collision_Detection.swf uses the following code, which is pasted under the blue ball.
```markuponClipEvent (enterFrame) {
if (_root.redball.hitTest(this)) {
_root.condition = "touching";
} else {
_root.condition = "not touching";
}
}```
The how:
On each frame the blue ball will check to see if it is touching the red ball (_root.redball), and if it is, it will set the variable 'condition' to 'touching'. Otherwise, it will set 'condition' to 'not touching.'
The code:
Line 1 tells the movieclip to execute the code between the { and } on every frame.
Line 2 runs the hitTest to see whether the two movieclips are touching
Line 3 is executed if the movieclips are touching
Line 5 is executed if the movieclips are not touching.
**MOVIECLIP DUPLICATION**
Being able to duplicate movie clips gives a Flash developer a lot of power. In order to create 10 bad guys, all the developer needs to do is run a loop 10 times that duplicates a single bad guy. This method has many advantages. Check out [Movieclip_Duplication](http://s153.photobucket.com/albums/s216/digital_fire_tutorial/movieclip_duplication.swf) for an example of what you can create using movieclip duplication.
Flash provides the duplicateMovieClip() function for this purpose.
duplicateMovieClip() takes in three arguments:
The first is the instance name of the target movie clip to be duplicated.
The second is the new instance name of the clone
The third is the layer on which to place the clone. Unless this number is unique, Flash will overwrite what is on the layer with the new movieclip.
So here is the code used in the example file:
This code goes on the button:
```markupon (release) {
_root.i += 1;
duplicateMovieClip(_root.triangle, "triangle"+_root.i, _root.i);
}```
The how:
A button will have the 'on(release)' event handler which will detect when it has been pressed. When this happens, Flash will add 1 to the variable 'i', which will be a unique id for the new movieclip. Flash will then duplicate the movieclip named 'triangle', give it the name 'triangle'+i, which would be, for example 'triangle5', and then place the triangle on the layer i so that it does not overwrite any other existing triangles.
The code:
Line 1 detects the release function of the button to execute the code between the { and } when a button is pressed.
Line 2 adds 1 to the variable _root.i
Line 3 duplicated the movie clip
This goes on the first frame of the movie:
```markup_root.i=0```
The how:
We dont need an event handler in this case because the code is on the frame so will be executed upon arrival to the frame. The code will intialize the variable _root.i and set it to 0. And this code goes on the triangle:
```markuponClipEvent (load) {
stagewidth = 520;
stageheight = 270;
this._x = random(stagewidth);
this._y = random(stageheight);
this._alpha = random(50)+50;
this._rotation = random(360);
}```
The how:
If all we do is duplicate a movieclip, flash will place the new one exactly on top of the old one. This chunk of code will randomly place the movieclip when it loads, give it a random rotation, and give it a random opacity (alpha) to set it apart from the other triangles (opacity and rotation optional but cool).
The code:
Line 1 tells Flash to execute the code between the { and } when the movieclip loads.
Line 2 defines a variable 'stagewidth' for later use in random positioning
Line 3 defines a variable 'stageheight' for later use in random positiong
Line 4 sets the '._x' (x coordinate) of the movieclip to a random number between 0 and stagewidth
Line 5 sets the '._y' (y coordinate) of the movieclip to a random number between 0 and stageheight
Line 6 sets the alpha (opacity) value of the movieclip to a random number from 50-100;
Line 7 sets the '._rotation' (rotation) of the movieclip to a random number between 0 and 360.
**Download**
If you want to download the examples visit the url below:
[download](http://dfmission.freehostia.com/flash/)
**Conclusion**
I hope you liked this tutorial. If it gets good reviews I plan on writing another one which will cover
*Mousetracking rotation [preview](http://s153.photobucket.com/albums/s216/digital_fire_tutorial/auto_rotation.swf)
*The distance Formula [preview](http://s153.photobucket.com/albums/s216/digital_fire_tutorial/distance_formula.swf)
*Projectiles [preview](http://s153.photobucket.com/albums/s216/digital_fire_tutorial/firing_projectiles.swf)
and possibly more. I didn't combine the two articles in fear of creating one so long nobody would dare to read it... anyways feel free to PM me about anything in the tutorial and I will be glad to help. This is my first article so go easy :)
And yeah I go under the sn of both DigitalFire and BlackDragonHax, so don't be worried if you see this article posted elsewhere by one of the two.
Please leave a comment :D
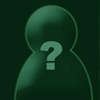
spyware 17 years ago
Not only the best flash article on hbh, but one of the nicest in general. Awesome! Looking forward for more of this stuff man :)
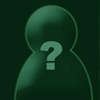
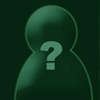
ghost 17 years ago
nice article :) although i prefer c++ for making games myself, this is very useful :)
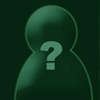
ghost 17 years ago
Very nice, it is a good article for those who are new to flash or to those who doesnt get the basic concept, good article
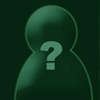
ghost 17 years ago
Excellent Article, I have been learning flash and have been shock on the lack of quality articles on the web. This is top notch. I hope you make more.
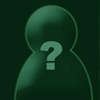
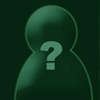
lukem_95 17 years ago
Great article, although i also prefer C++ or C# (XNA!), although it doesnt run on EVERY OS lol, but yeh every popular one. :D
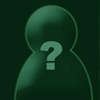
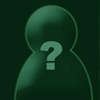