Win32 ASM Tutorial
Win32 ASM Tutorial
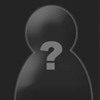
Assembly Language is the most low-level programming language. Every instruction in Assembly (asm) has an equvilent instruction in Machine Code. ASM programs are typically very small, and are very fast. The price for this efficiency is increased complexity in programs.
Anyone who has programmed in C++ will usually be familiar with the console window in which non-GUI programs execute. In ASM, there is no console window (unless you code it in). Instead, the Windows API functions will be used to perform similar tasks to what can be accomplished with the use of the console.
In this article, I will show you how to display a simple message box, containing the text "Hello World". You will need MASM (I suggest you download a copy from the URL at the end of the article. I will show you the code first, and explain it afterwards. NOTE: HBH strips out slashes, so I will place a * where a slash should be
.386 .model flat, stdcall option casemap:none
include masm32includewindows.inc include masm32includekernel32.inc includelib masm32libkernel32.lib include masm32includeuser32.inc includelib masm32lib*user32.lib
ExitProcess PROTO uExitCode:DWORD MessageBoxA PROTO ,:DWORD, :DWORD, :DWORD, :DWORD
.data MsgBoxCaption db "My first ASM program",0 MsgBoxText db "Hello World!",0
.code
Main:
INVOKE MessageBoxA, NULL, ADDR MsgBoxText, ADDR MsgBoxCaption, MB_OK
INVOKE ExitProcess, NULL
end Main
The first 3 lines are instructions telling MASM that we want to use the 386 instruction set, the flat memory model (the one that is avaliable in win32), the stdcall method of calling functions. We also specify case-sensitivity. The next 5 lines are fairly straightfoward too. Think of them as C/C++ #include statements. The .inc files are simple text files included with the version of MASM that I use (see the link at the bottom of the article). They define some constants and function prototypes for us, so we don't have to worry about defining them in all our programs. The includelib lines tell MASM what import libraries to link our program to. You can specify them on the command line for the linker program, but it is easier to put them here (then we can build our program with Qeditor).
Next, we define function prototypes for ExitProces (a kernel32 function) and MessageBoxA (a user32 function). A function prototype is an instruction that gives MASM information about the number and type of arguments that the function takes. This is only required if you are going to invoke the function (which is a much better way that simply PUSH'ing the parameters and CALL'ing the function, but I won't go into too much detail on that here). We will be using invoke in this article.
After that, we define the start of the .data section, which contains initialized data. There is a section for uninitialized data too, but we don't need that here. You need only define the sections that you will use, and the start of one section marks the end of the previous one. We then define 2 0-terminated strings, which are the text and caption for our messagebox.
Next comes the .code section. All your code must reside between a label (I will use Main here, but the name is unimportant) and end label. We then make our call to MessageBoxA which will display a messagebox with the specified text, caption and style information. The first parameter is NULL, because we don't have a window for the messagebox to become a child of. By using the ADDR keyword, MASM will fill in the appropriate hex-values at assembly time, so we don't have to. We are telling it to put in the addresses of our text and caption. The last parameter in the call is MB_OK, a constant defined in windows.inc (look it up if you wish). This will give us a messagebox with a simple OK button.
Then we invoke ExitProcess with a single parameter of NULL, which tells our program to exit with an exit code of 0. The end Main label follows it, which marks the end of our program.
Run the qeditor.exe program in the MASM directory and put in all the code and save it as whatever.asm. Then go to Project -> Assemble and Link. MASM will then execute the 2 programs required to build our program. It will produce 2 files, a .obj and a .exe in the same directory that you saved your .asm file in. The .obj file is produced as a product of the assembling process, and is unneeded once the exe has been created from it. Go ahead and run the .exe file, and a messagebox will appear on your screen. Congratulations, you have just built your first program in ASM! I might do some more of these tutorials to cover other aspects of win32 ASM programming (like how to create a GUI, which is easier than it might seem!).
–– Links –– http://www.uploading.com/files/0EP7CWEL/masm32.zip.html
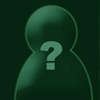
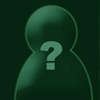
ghost 18 years ago
Nice article mate. Post some more about asm!
here's a small asm program which users MessageBox with a 'yes' and a 'no' button. if yes is clicked it will execute notepad.exe with WinExec.
Overcommented source code (compile with FASM): http://www1.webng.com/dw0rek/tmpdir/asdf_02.asm Executeable: http://www1.webng.com/dw0rek/tmpdir/asdf_02.7z
cheers /dw0rek
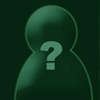
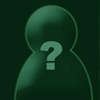
ghost 18 years ago
Well yea, MASM is closer to C than asm, but when you can have a 2KB GUI application, who really cares? :happy:
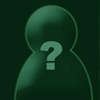
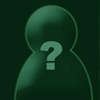
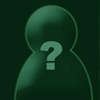