Introduction to Assembly (MIPS)
Introduction to Assembly (MIPS)
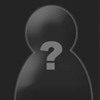
Introduction to Assembly Lanaguage. Part 1.
In this tutorial we will be using MIPS assembly and using a MIPS32 Simulator called SPIM.
Spim is a self-contained simulator that will run MIPS32 assembly language programs. It reads and
executes assembly language programs written for this processor. Spim also provides a simple
debugger and minimal set of operating system services.
You can download SPIM at: http://www.cs.wisc.edu/~larus/spim.html You can use this for windows / linux / mac etc.
Once you have downloaded SPIM, we can start to create our first assembly program.
Also here is a link to further explain how to start you first program. http://www.cs.usna.edu/~lmcdowel/courses/si232/spim/PCSPIM-HowTo.htm
First I will explain what assembly is. The words of a machine's language are called instructions,
and its vocabulary is called an instruction set. We will be using the mips instruction set. Assembly language is symbolic equivalents of the machine-language instructions of a particular
computer.
When we use high level languages such as c and c++, they are compiled into an executable. MIPS
does not compile, what happens is executable code gets interpreted into assembly language and then
into 1's and 0's (Machine Language). Hence assembly is the lowest level of programmerable code.
It is very fast and very efficient as you can control basically anything you want (memory,
registers, devices, etc).
Now to introduce some MIPS operands.
In MIPS, data must be in registers to perform any arithmetic. Registers are stored in the CPU.
There are functions in assembly that load constants into a register and also store them back into
memory.
Mips registers. Mips has 32 registers r0-r31 and use the '$' to distinguish that they are registers. In assembly their name is as follows.
$zero = 0 $at = Reserved for assembler $v0-$v1 = Stores results $a0-$a3 = Stores arguments $t0-$t9 = Tempory, not saved $s0-$s7 = Saved results $k0-$k1 = Reserved by OS $gp = global pointer $sp = stack pointer $fp = frame pointer $ra = return address $Hi = Results of multiply and Divide - This is the remainder $Lo = Results of multiply and Divide - This is the quotient
Here is a link that basically says what I just said and explains further http://www.cs.uiowa.edu/~ghosh/01-25-07.pdf
Now for some explantion on memory. Memory is just a long single array that stores data. MIPS
uses byte addresses, so sequential words differ by 4. 1 byte is 8 bits, so a word is 32 bits.
Memory holds data structures, such as arrays, and spilled registers, such as those saved on
procedure calls.
Here are some basic instructions used by MIPS.
Instruction…….Example……….Meaning add …….. add $s1, $s2, $s3 …….. $s1 = $s2 + $s3 subtract ……. sub $s1, $s2, $s3 ……. $s1 = $s2 - $s3 multiply …….. mult $s2, $s3 ……. Hi, Lo = $s2 * s3 divide …….. div $s2, $s3 …….. Lo = $s2 / $s3, Hi = $s2 mod $s3
Data Transfer load word lw $s1, 100($s2) $s1 = Memory[$s2+100] Word from memory to register store word sw $s1, 100($s2) Memory[$s2+100] = $s1 Word from register to memory
There is alot more to it but this is just an example.
There are also logical operands such as and / or etc.
Conditional branchs such as beq, bne which is branch on equal and branch on not equal.
Jumps such as j, jr, jal which is jump, jump register, jump and link
I will explain these later in another article possibly, or just read up on what these do since
this is an introduction.
A basic example now. f = (g + h) - (i + j);
add $t0, $s1, $s2 # Register $t0 contains g + h add $t1, $s3, $s4 # Register $t1 contains i + j add $s0, $t0, $t1 # f gets $t0 - $t1, which is (g+h)-(i+j)
Now that we understand some basics, we can take a look at a fully functional program. You can
just code it in notepad and then load it into the assembler. Make sure to save it as .asm. Here is a complete program I made that calculates the fibonacci of a value. Fibonacci numbers are the numbers in the Fibonacci sequence 0, 1, 1, 2, 3, 5, 8, 13, 21, … ,
each of which, after the second is the sum of the two previous ones.
#By Chris JOhansson
.data
output: .asciiz "\nEnter a value n for fib: " result: .asciiz "fib of n = "
.text
.globl main
main:
li $v0, 4 # prints to screen
la $a0, output # loads address of output
syscall
li $v0, 5 # read and integer
syscall
move $a2, $v0 # copy $a2 = $v0
li $a1, 0 # $a1 = 0
li $a0, 1 # $a0 = 1
jal fib # call function fib
move $s1, $v0 # store result of $v0 in $s1
li $v0, 4 # print result
la $a0, result # loads address of result in $a0
syscall
li $v0, 1 # prints integer
move $a0, $s1 # stores $s1 in $a0
syscall
li $v0, 10 # exits cleanly
syscall
fib:
bne $a2, $zero, note0 # if $a0 not equal to $zero, goto note0
move $v0, $a1 # sets $v0 to $a1, which $a1 is b.
jr $ra # returns 0
note0: # not equal to 0
addi $a2, $a2, -1 # This if for the count = count -1
add $t0, $a0, $a1 # $t0 = a + b, $a0 + $a1
move $a1, $a0 # $a1 = $a0 b = a
move $a0, $t0 # $a0 = $t0 a = a + b (old)
j fib
So from what we know now, you can understand basically what this does and how it works.. This is a
bit more advanced but you get the idea.
For more introduction to assembly visit:
http://en.wikibooks.org/wiki/MIPS_Assembly#Section_1:_Introduction_to_MIPS Or just google some introductions.
This is my first article so please rate it and comments are welcome.
Thank you.
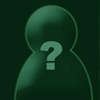
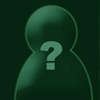
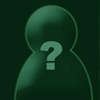
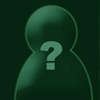
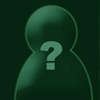
ghost 18 years ago
well you don't really use assembly for developing software. Its more to study and learn how the computer works. Its good for small programs that can control specific memory / CPU / IO routines and alot of virus's are made in assembly.
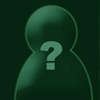
Uber0n 18 years ago
@Frag_Wurm: Let's say you've built your own PCI card or something. If you want to use it, you probably want to learn ASM ;) And you can make "real" applications in ASM as well.
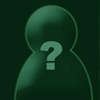