Visual Basic File I/O
Visual Basic File I/O
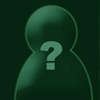
File I/O stands for File Input/Output
In this tutorial we will be accessing .txt files for input and output and using the app.path command. We will also be using a Do While Loop, and an array.
You should have some basic knowledge of VB.
Ok so start off by creating a standard exe. Create a textbox, name it txtWord Create a list, name it lstWords Create a button, name it btnGet, change the caption to "Get" Create another button, name it btnSend, change the caption to "Send"
We will be using the textbox to type the word you want to send, the list to display the words from the text file. We will be using btnGet to run code that will get the words from the .txt file and display them to to list. We will be using btnSend to run code that will send the word from the textbox to the .txt file.
To write to a .txt file you need to open it first. To do this you will need to type this in btnSend:
Open App.Path & "\your_name.txt" for Append as #1
Open opens the file. App.Path, gets the location of where the application is located. Append will add what ever you type in the textbox to the next line in the .txt file. You can also use, Input and Output. Output will Replace any text that is already in the .txt file. Input will get input from the .txt file.
Next we will be using the Print command. I prefer using print because if you use write, it will include quotations around the text in the .txt file. So now type:
Print #1, txtWord.Text
That will send the text in the textbox to your .txt file.
Now you need to close the .txt file. to do that you type:
Close #1
Now type something in the textbox and press the btnSend button. Do it a bunch of times. Then open the .txt file. If you saved your project and form, then go to the directory where you saved it and the .txt file should be there. If not, then you saved the .txt file from the VB main executable. Which is located in the VB folder. Go to: C:\ Program Files\ Microsoft Visual Studio\ VB98, it will be in there named: your_name.txt.
The .txt file should contain all the words that you entered.
Ok so now that you guys have got that down. Were gonna get input from the .txt file. In this part we will be using the: EOF() command which stands for End Of File, and a Do While Loop.
Double click on btnGet to go to the code editor.
At the top of the private sub type:
Dim wordArray(1 to 30) as String, intCount as Integer
Dim will dimension all the variables you type in as the type you put it as. Here we've dim'd wordArray(1 to 30) as a string, its a string type. And intCount as an integer, its a integer type. We will store all the words in the .txt file in the array: wordArray().
Well We need to get the words from the .txt file, so lets open the .txt file. Do you remember how to do that? Of course except instead of using Append we will be using Input. Type the following:
Open App.Path & "\your_name.txt" For Input as #1
Now we need to start a loop that will loop the array and add a word to it each time it loops through. How is it gonna know when to stop? Well we'll use a Do While Loop and the EOF() function.
Ok so now type the following to start your loop:
Do While Not EOF(1)
That initiates out loop. And tells it to loop while its not at the End of File 1. So once it hits the end of file 1 it will stop the loop. Now we need to create an accumulator to keep adding a number to a variable each time it loops through. Well we already created our accumulator variable. Which is: intCount So type:
intCount = intCount + 1
That will accumulate (add 1 to the variable intCount) everytime the loop runs through. Now type:
Input #1, wordArray(intCount)
That will add one word from the .txt file each time with the array number adding one each time. Now type the following to add the words to a list.
lstWords.AddItem(wordArray(intCount))
That will add a word to the list everytime the loop runs through. Now just finish your loop:
Loop
And Close your file.
Close #1
There you go. Theres your tutorial on File I/O. I hope you have a better understanding on the basics of File I/O.
** if you liked this article go ahead and comment and rate it :-D**