Visual Basic Random Numbers
Visual Basic Random Numbers
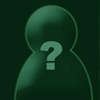
In this tutorial I'm gonna teach you how to generate random numbers.
Well firstly You should open up Visual Basic 6, double click on Standard EXE.
Ok so now what do we want to output the random numbers to?
Hmmm… Well maybe we should just put it on a label. Yes? Great
Put a Button and Label on your form.
Name the button: btnRnd Name the label: lblRnd Clear the caption on the label.
Double click on your button and heres something new to learn:
Dim num1 as integer
That dimensions the variable num1 as an integer. So no matter what THAT variable will be a number. If you assign num1 to be "fish" then its value is 0. Got it? Good.
Ok so once you've typed that enter down a couple times and type:
Randomize
That randomize's the following code.
Now enter down a couple more times and type:
num1 = int(Rnd * 101) + 1
That assigns a number between 1 and 101 to the variable num1. Rnd Generates a random number and the 101 part is the highest number you want the random number to be. The + 1 part is where you want it to start. If you dont care if it starts at 0 then dont put anything there.
Ok once you've got that down type:
lblRnd = num1
That should generate your random number for you.
Now what can you do with random numbers? Well there are alot of options most of which you should figure out for yourself. You COULD create a game with it, like Magic Dice or a lotto game.
And remember that You can do as maney numbers as you want, you dont have to limit yourself to just num1.
Example:
Dim num1 as integer, num2 as integer, num3 as integer
Randomize
num1 = int(Rnd * 101) + 1 num2 = int(Rnd * 10) + 1 num3 = int(Rnd * 32) + 1
lblRnd1 = num1 lblRnd2 = num2 lblRnd3 = num3
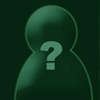
ghost 18 years ago
Thought I'd be the first to mention that there is no such thing as randomly generated numbers, except atomic decay. So the only true random number generator will be the quantum computers
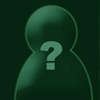
ghost 18 years ago
Ya. Nice article tho. And I'm kinda thinking humans can't even make a random #. At least I can't :P
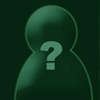
ghost 18 years ago
Well you could at least add a function to generate random with bound … Function Random_Number (int min, int max) Randomize Random_Number = (Rnd*(max-min)+min) End Function The synthax is probably wrong because i haven't done VB since a long time but the algo should be good.