A brief Introduction to C++
A brief Introduction to C++
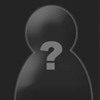
Hello, I am DigitalOutcast, In this article I plan to teach you some basic C++ and provide you with links to some websites that can assist you in learning C++. So lets get down to business. A brief look at C++: C++ is a superset of the original C language. C++ was created by Bjarne Stroustrup to improve upon the C language by adding Object Oriented or OO conecepts to the language. In a way without the OO programming involved in C++ you would just be left with C. So good news for all you old C programmers out there, all functions and libraries in c are available in C++. This makes the language pretty powerful and allows for various ways to write the same program with different functions and libraries.
Vocabulary: Here are some terms that you will most likely encounter in C++ programming.
- Variable- A variable is a small area in the computers memory where values are stored for later use. Think of it as a box that holds stuff that you don't need at the very moment, but want to keep so that you can use it in the near future.
- Statement- A statement is a line of code in C++. A statement always ends with a statement-terminator (;).
- Statement-Terminator- A statement-terminator is just a semi-colon that appears after a C++ statement, this informs the C++ compiler that the statement ends here.
- Compiled Language- A compiled language is a language that is written in plain text and is later converted into machine-language or binary by a tool known as a compiler. C++ is a compiled language.
- Compiler- The tool or program that is used by a programmer to convert the source-code document into a machine-language object file.
- Linker- A linker is the piece of software that links object file to the required libraries and created the executable file that then can be run.
- IDE- IDE stands for Integrated Development Environment, this is a set of tools that has an editor and compiler and linker all built into one, this allows for much faster code production.
Getting into the source: Now that we know all the vocabulary that this article will use its time to start coding. In order to be successful as a C++ programmer, you have to be able to code proficiently and logically. Lets begin by looking at a simple but famous program. The God-like Hello World! program. At some point every programmer even the great Linus Torvalds has written this program.
#include <iostream>
#include <stdio.h>
using namespace std;
int main()
{
cout <<"Hello World!";
system("PAUSE");
return 0;
}
Now to explain this good old source code file. The first two lines of the program are what is known as Pre-Processor Include Directive, These lines of code include the contents of the files that are specified (in this case iostream and stdio.h) into the source code file. These files are called library files. Library Files contain important program code that allows your program to work effectivly.
the next line using namespace std; is a little more complicated than this introduction article wishes to go. For now just know that you need this in most programs. The next line int main() defines the function main(). This function is the most important function you will ever use. This function defines the entry-point for the program to start execution. The main() function is the only function that is required in a c++ progam. The { defines theopening of a block. A block is a small section of code that is grouped together to be used by specific functions. The line cout <<"Hello World!"; is the first of our commands that we will learn through out this series. cout is the standard output stream that is used to print text to the computer monitor. This will be used quite often when you get into serious programming. << tells the compiler to inject the string "Hello World!" into the cout stream and results in the text appearing on the computer monitor. Then finally we have the statement terminator (;), this simply tells the compiler that this statement is finished. Next we have the statement system("PAUSE"); this line of code simply tells the compiler to pause and wait until a key is pressed. then we have the return statement. A return statement of 0 usually means that the program has finished successfully. Then we close the block and compiler and run our code.
NOTE: C++ is a Case-Sensitive language. This means that uppercase and lowercase letters are completely different and cout is not the same as Cout or cOut.*
For more information on the C++ language you can check out one of the following links: Teach Yourself C++ in 21 Days Free Ebook Cprogramming.com
Thank you and I hope you have enjoyed this lesson. Please look for my other articles either on My Homepage or on HBH. Good Luck an feel free to message me if you have any questions.
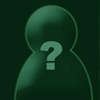
SySTeM 18 years ago
Nice, I edited the formatting a little bit, showed links and put the code tags in
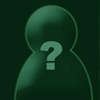
ghost 18 years ago
tnx, i made it for my website and wanted to submit it here, the format on my site was different so it didn't translate, thanks
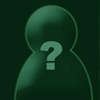
ghost 18 years ago
Ok… nice for the Veeeery beginners… but… one thing… You said "2. Statement- A statement is a line of code in C++. A statement always ends with a statement-terminator (;)." I will get the second as true… since it is possible to write as many statements as you want in…. a line ;)
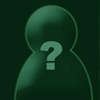
bl4ckc4t 18 years ago
How many articles of "basic intro to C++" are there now?
All they tell is how to do Hello, World. At least make one that codes a simple key logger or something interesting!
-_-
Bl4ckC4t
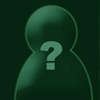
ghost 18 years ago
Never ever use system("PAUSE");, but nonetheless it's alright and it's another one of the many 'intro's on this site. :wow:
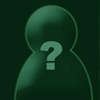
ghost 16 years ago
Adding on to what T-Metal-Risen said, you shouldn't use system calls when you don't need to. cin.get(); would work just fine there, and wouldn't cause the program to be halted, it doesn't really make a difference in such and introductory program, but it's a bad coding habit.