Port Scanner
Port Scanner
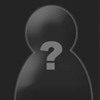
Ok, here is our java code….with some comments. more on it at the bottom
import java.io.; import java.net.; public class socketTest { public static void main(String args[]) { String save = ""; String in = ""; try{ BufferedReader input = new BufferedReader(new InputStreamReader(System.in)); //initialize the input stream so that we can tell what needs to be searched
Socket sock;
System.out.print("IP> ");
in = input.readLine();
System.out.print("Starting port> ");
int sport = Integer.parseInt(input.readLine());
System.out.print("End port> ");
int eport = Integer.parseInt(input.readLine());
String ip = in;
save = ip + " scanned on ports " + sport + " to " + eport + "\n";
try{
HttpURLConnection http = (HttpURLConnection)new URL("http://www." + in).openConnection(); //sets up a http scanner. will run on any http capable host
http.setDoOutput(true);
http.connect();
save = save + "HTTP detection \n";
save = save + "Proxy: " + http.usingProxy() + "\n";
String temp = http.getPermission().toString();
int stop = 0;
for(int k = 0; k < temp.length(); k++)
{
if(temp.charAt(k) == ')')
stop = k ;
}
String perm = temp.substring(27,stop);
save = save + "Permission: " + perm + "\n";
save = save + "Request Method: " + http.getRequestMethod() + "\n";
}catch(Exception x2){System.out.println("Http scan failed.");}
//begin port scanning here
for(int port = sport; port < eport+1; port++)//runs through all the ports you selected
{
try{
System.out.print("Testing port " + port + ":");
sock = new Socket(ip, port);
System.out.println(sock.toString());
save = save + (port + " " + sock.toString() + "\n");//build output stream for saving to a file.
}catch(Exception x)
{
if(port > 999)//im OCD and need it formated correctly.
System.out.println("Failed");
else if(port > 99)
System.out.println(" Failed");
else if(port > 9)
System.out.println(" Failed");
else if(port <10)
System.out.println(" Failed");
}
}
}catch(Exception x1){x1.printStackTrace();}
try{
BufferedWriter out = new BufferedWriter(new FileWriter( new File(in + ".log")));//writes found info to a file
out.write(save);
out.close();
}catch(Exception x)
{
System.out.println("Error:");
x.printStackTrace();
}
}
}
Alrighty. now that you've looked at that and gone ZOMG WTF or if you know java, your thinking, man this guy doesnt know anything. Well, for the former, ill explain, and the later….go write a better one…then say something. otherwise, STFU.
So we begin with just basic keyboard input. This allows the program to by dynamic and not required for hardcoded scans. This is JDK 1.4, newer programmers may see BufferedReader input = new BufferedReader(new InputStreamReader(System.in)); and be confused…this is the old school(and in my opinion better) version of your Scanner s = new Scanner(System.in); //i think thats right
We then run through getting the inputs we need…im hoping you can figure that one out on your own.
Next, we scan this for http capabilities. HttpURLConnection http = (HttpURLConnection)new URL("http://www." + in).openConnection(); http.setDoOutput(true); http.connect(); That will initialize a http object for the IP or webaddres you gave. If it exisits it will check for things like proxies and such. This is really more of just an addon…not the real purpose of the program, in v2.0 this will be further implemented.
OKAY, now to the juicy part. :) Here all we do is set a socket on each port and check if we can connect, if not it throws a connection exception and skips it trying the next one. Again, this version is slow, going one at a time, v2.0 will have a multiple threads to speed things up. This buys time by letting them sit for a bit waiting for that connection while the others do. It cant check them simultaniously, but why wait for one to connect when you could be setting another 20 or 30?
Finally we initialize a file writer (another JDK 1.4 setup) to save this so you dont have to watch. Set it, goto bed, wake up, hack. Enjoy.
–Samurai
[edit 10-9-06] I have currently completed version 2.0. It is much faster and has many more options and is graphical. I will be uploading the source, and a .jar file for usage. Most likely I will post a tutorial ( or a series) on how I built each module
[edit 13-11-06] Version 2.0 is fully packed and ready to go as an .exe. Both .exe and source are available.