PHP File upload application & Importance of File validation.
PHP File upload application & Importance of File validation.
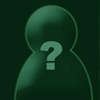
The target audience for this article is to the beginner web developer and the main objective is to shed light on the importance of validating "file types" that you would allow users to upload to your server; it is NOT on how to build the perfect file upload application.
A couple years ago I got into Web Development. One of my first assignments was to develop an image upload application for a client. I was very “new†to this field so I had no idea what I was doing. So I think I did what most noobs do - look around online for scripts in code banks and “take a snippet of code here and there†until you make what you need.
My tragedy was I had no clue to the functionality of certain lines of code, so to ease my confusion I omitted certain lines of code in my final application to offer the BARE BASIC MINIMUM of what was required, and that was to Upload the damn file…Not taking into consideration of what “file types†would be allowed and/or not allowed! Big Mistake! Internet Security was never a concern of mine back then, because after all, who would want to hack my site or my client’s site, right?! ~smirk
Several months later when working on a similar project I realized my error and corrected the problem. The problem is this; without validating the "file type" (or even file size in some cases) being uploaded to your server anyone can essentially upload data to your server that you never intended to host. In my case, my application was “intended†to upload “images†but in its original development it was an open gateway for anyone to upload anything they wanted (i.e. junk files, malicious files etc). It doesn’t take a lot of imagination for a seasoned script kiddie to figure a crafty way “to pull someone’s pants down, grease’em up, and aim for penetration of exploitation†due to this very preventable flaw.
Here is a sample script for a very basic yet functional image upload application:
- <?php
- if (isset($imagefile)) {
- $path = "/image/upload/directory/";
- $path = $path . basename( $_FILES['imagefile']['name']);
- if ($HTTP_POST_FILES['imagefile']['type']=="image/gif" || $HTTP_POST_FILES['imagefile']['type']=="image/pjpeg" || $HTTP_POST_FILES['imagefile']['type']=="image/jpeg"){
- if (move_uploaded_file($_FILES['imagefile']['tmp_name'], $path)) {
- echo "The file ". basename( $_FILES['imagefile']['name']).
- " has been uploaded";
- }
-
}else{
-
echo "Wrong File Type";
-
}
- }
- ?>
- <form enctype="multipart/form-data" action="<?php $_SERVER['PHP_SELF'] ?> " method="POST">
- Choose a file to upload: <input name="imagefile" type="file" /><br />
- <input type="submit" value="Upload File" />
- </form>
======================================================= I would like to direct your attention to line 5 (not considering the line break). Basically this is the line of code that sets the parameters for the “upload†to occur.
The parameter being this: "IF" the file type is a “gifâ€, “pjpegâ€, or “jpeg†- than the message, “The file ‘file_name’ has been uploaded successfully†will display (line 7) and the image file will be uploaded to the directory path you set (line 3). Yes you will need to edit this line!
ELSE
"IF" the File type is NOT a “gifâ€, “pjpegâ€, or “jpeg†- than the message, “Wrong File Type†will display (line 9) and the image file will NOT be uploaded.
You can of course modify this for any file type you intend a user to upload to your server (i.e. .txt, .pdf, .doc, .png etc).
As I stated before this article is intended for beginning developer to be aware of the importance of file validation when developing upload applications. I agree, the above example is not the most secure “fool-proof†script but I chose to use it for the sake of simplicity due to its minimal lines of code and merely as a demonstration for the topic of this article. There is a lot more to consider and to be scripted when developing an upload application than what’s included in the above example.
One Final note for the hopeless and clueless like myself, if you finish developing your upload application correctly and you are greeted with the following error message:
Warning: move_uploaded_file(/img/upload/directory/â€imagefilenameâ€): failed to open stream: Permission denied in /fileupload/directory/upload.php on line “whateverâ€
Solution: Try changing permission settings to the actual folder you are allowing users to upload to; that will help!
I hope this helps - nimbus01
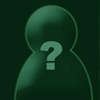
ghost 18 years ago
Good tutorial for people who haven't done anything like this before (like me). Well done :)
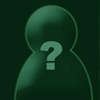
ghost 18 years ago
I am working on PHP for the time being and it was reassuring I could see what's going on here. Brilliant article dude.
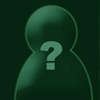
ghost 18 years ago
Thanks for the update! You can edit your article by viewing it VIA your profile ;)
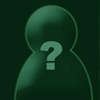
ghost 18 years ago
Actually, THIS IS NOT SAFE AT ALL. This method will allow ANY file ending with .gif to be uploaded. Go ahead and try it yourself. Write some html/javascript, save it as "myfile.gif" and try to upload it, BAM, it gets uploaded. You don't even need to hex edit for it to upload GIF images. I did not do any tests on other files, but it won't allow you to upload a file ending with .jpg or .jpeg. I'm assuming you can bypass this just by hex editing the image and inserting the proper image tags with the hex editor. DO NOT USE THIS IF YOU DON'T WANT XSS ATTACKS ON YOUR SITE
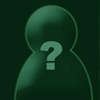
ghost 18 years ago
Furthermore, your article does not address your topic of discussion. You mention briefly that it is important that we validate file types, but no reason why or how. You then post a completely insecure script, which does not get the job done at all. This script would not protect you from even the most NEWBIE of all hackers.
Basically your article has no depth. You should give us a good example of why file validation is important, and then procede to demonstrate A SECURE EXAMPLE. It's meaningless to post an example that doesn't work. That teaches NOTHING. Like I said before, I was able to upload any file with a GIF extension using this script.
I don't want to be rude, I just want to point out to everyone that this script is COMPLETELY insecure. I also want to give you a few pointers, so maybe you can improve your article, and hopefully write better articles in the future. Thanks :)
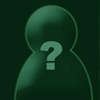
ghost 18 years ago
Actually, I am wrong. It does allow you to upload any file type specified in line 5 of the code, so long as that file ends in the required extension, and regardless of the contents of the file. So your script only checks file extensions.
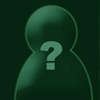
nimbus01 18 years ago
@ paintballa_4life2005, I appreciate your constructive criticism – But in all fairness the “topic of discussion†was the importance of “file validation,†as stated in the title, when developing an upload application. In paragraph one I stated, “This article is NOT on how to build the perfect file upload application,†and in my closing paragraph I clearly stated, “… the above example is not the most secure “fool-proof†script… There is a lot more to consider and to be scripted when developing an upload application than what’s included in the above example.â€
So I thank you for reiterating in the comments section what I already stated in my article…
But again for those that read the article, the scope of the article is simply to make the “beginner aware†of the importance of file validation when developing upload application and give them an idea on how to do so.