Java Programming
Java Programming
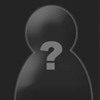
JAVA tutorial
I accidentaly deleted my other article that was the same thing, but this is adding on to pyrodudes article. First off, it is case senseitive. If you were to capitalize or not capitalize something, your program will not work. Second, you need make sure your brackets are in the right spot. Brackets start and end different parts of your program. To start codeing in Java, you will need a compiler. I use JCreator, but you can use google and find any free one. Our first program will open a black screen and type "hello world"
public class hello { public static void main ( String [ ] args ) { System.out.print ( "Hello World!" ); } }
To run this file, go to build>compile file then build>excecute file. Congradulations!!! You have just made your first program! But why does it work? public class is the name of your file, so in order for this to work you must name the file hello. public static void main ( String [ ] args ) { is the bengining of the main part of the program. System.out.print tells the computer to print the line hello world. Remember to put your text in ( ) and "" with a ; at the end. Then, as always, we must close the brackets we started in the begining. Sometimes, if, System.out.print somethimes doesnt work, try System.out.println. Our next program will ask the user for their name and say "Your name is…". For this program we must add some things tot he begining of the program. F.Y.I. if you put // then text in your program, that section will be ignored.
import java.io.*;
public class askname { public static DataInputStream Input = new DataInputStream(System.in); // this tells the computer data will be inputed by the computer.
public static void main (String [] args) throws IOException{
System.out.print ( "What is your name?" ); String name = Input.readLine ( ); // this tells the computer to read what has been typed and make it into a part of the file called name. System.out.print ("How old are you?" ); String age= Input.readLine(); // these lines tell the computer that it is a number. int a= Integer.parseInt(age); System.out.println (name + " is " + a + " years old" ); // this tells the computer to print whatever the user typed.
} }
Excercise: Try makeing a mad libs program. Have the user type in a noun, verb, etc. and print them out at the end like mad libs.
We will now make a program using if and else statements. If and else make the program recognise what the user types. If they type anything else than what the if statement is, the else statements comes in and prints out what it wants.
import java.io.*;
public class test3 { public static DataInputStream in = new DataInputStream(System.in);
public static void main (String [] args) throws IOException{ System.out.println ("What is your name?"); String name = in.readLine ( ); if ( name.equals ("bob")) // this line tells the computer to type "hi bob" if what the user types is bob. System.out.print ("Hi bob!"); else // this tells the computer that if what the user types is not bob, to type "hi person who is not bob". Else statements need brackets after the else and and after the System.out.println. { System.out.println ("Hi person who is not bob!"); } } }
Excercise: Try makeing a program like on a game show. Ask them to type a door and if the door is the same as what you specify, tell them they won.
Now we will start using numbers. Java can perform basic mathmatic operations, +, -, /, x. We have allready learned how to get intigers in past programs, so if we add another line we can make a simple calculator. Try adding this to an existing program after int x = Integer.parseInt(whatever); Get another int, and name it y or something then use the code.
{
int answer = x * x; // change * to any other operation. return answer; try { System.in.read () ; catch (Exception e) {} }
Excercise: Try making a grade calculator. Ask a user for 5 grades, add them up and divide them by fivd. You will need to make two seperate answer strings, one to add and another to divide.
Please comment
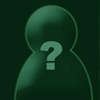
ghost 18 years ago
come on, comment please i want to know what you think or if any of you have actually done anything from here
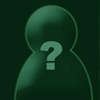
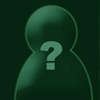
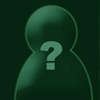
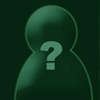