PHP Functions
PHP Functions
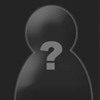
Welcome
I'm learning PHP and decided i would do a little tutorial on Functions in PHP. A lot of you probably already know what functions are, but some of you might not.
here is some example code on finding the length of a string.
<?php echo strlen('hello'); ?>
the result when you loaded the php script into your web browser would be
– 5
there is an example of a built in function in PHP. It takes one ARGUMENT, “helloâ€, and carries out the rest of the little program. Which in this case yields the length of the string “hello†which is five.
There are so many built in functions that are useful. But at some point or another your going to want to be able to make your own little functions to carry out specific processes. Here is an example of the hello World program.
<?php echo “Hello World!â€; ?>
which would yield
– Hello World!
If i was coding something where i would be printing out “Hello World!†a bunch of times, I would take it upon myself to code a function to do it for me. Like So.
<?php function hello_world() { echo “Hello World!<br>â€; } //now that i have the function written i could print this as many times as i wanted just by typing: hello_world(); hello_world(); hello_world(); ?>
which would yield:
– Hello World! Hello World! Hello World!
there was about as simple as functions get. I didn't even have to pass any arguments through the function. Here is an example of a simple function that you might create with arguments passing though.
<?php function print_this($arg1) { echo $arg1; } //now i would have to pass an argument through to get it to print anything. print_this("Hello World!"); ?>
which would yield…you guessed it:
– Hello World!
you can also call functions from inside other functions
That concludes the basics on making your own funcitons in PHP. Functions are a vital part of programming. They make certain tasks easier to perform multiple times and clean up code.