Drawing with java...
Drawing with java...
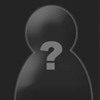
Ok, first off there are two types graphics facilities in Java, Drawing, and Graphical User Interfaces(GUI's). Graphic Options are only allowed within certain context, JFrames and JApplets.
A JFrame is simply a window that our program creates and a JApplet is simply a window that can be displayed from another application like a web browser.
Usually you add JPanels to your JFrame and in JPanels there are two important things that we can do draw figures and such and add other GUI components.
The graphics skeleton would look something like this import java.awt.; import javax.swing.;
public class GraphicsSkeleton
{
private static final int X_SIZE = 100, Y_SIZE = 100;
public static void main(String[ ] args)
{
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(X_SIZE, Y_SIZE);
GraphicsPanel panel = new GraphicsPanel();
frame.getContentPane().add(panel);
frame.setVisible(true);
}
private static class GraphicsPanel extends JPanel
{
public void paintComponent(Graphics g)
{
super.paintComponent(g);
// Add drawing code here.
}
}
}
For your drawing code there are many things you can do. A simple one would be drawing a line so here is how that would look.
g.setColor(Color.BLUE);
g.drawLine(20, 20, 40, 40);
Ok, so the g.setColor(Color.BLUE); is just simply telling the program to make that line blue, and the g.drawLine(20,20,40,40); makes that line. The first two digits the 20, 20 are the first x , y.
It starts at your left top window then x goes right, then the y goes down (backwards from what you normally do because y is positive) and the same thing with the 40, 40.
When you minimize sometimes the program doesnt re-paint itself so you would add the panel.repaint(); command so if it somehow gets minimized it will basically save your paint you have on there and when maximized it will restore it.
If you would like to add words in your drawings you can do it the same as you did the drawings, just follow the example below…
g.setColor(Color.RED);
g.setFont(new Font("Chicago",
Font.ITALIC + Font.BOLD, 40));
g.drawString("Whoo Hoo!", 40, 290);
the g.setColor(Color.RED); sets it to color red, g.setFont(new Font("Chicago", 40<– we have the new Font in there because Java does not have that font and the first 40 tells how big to make the font, so you set your own, the Font.ITALIC + Font.BOLD tells it to be Italic and Bold and the 40 is the x coordinate, the 290 is the y coordinate so just like i said before, it starts at the very top left corner and goes right 40, then goes down 290, and starts the begining letter there then finishes it on the string.
Thanks for reading, sorry for errors comment please :) -pyrodude0303
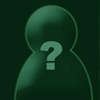
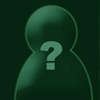
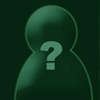
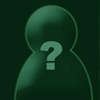
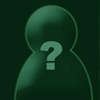
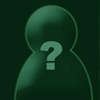
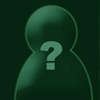
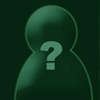
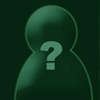