Logical and Bitwise Operations
Logical and Bitwise Operations
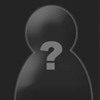
Logical and Bitwise operations
If you write in javascript, php, or a similar language, you're probably familar with conditional statements. Sometimes they deal with conditions, and other times numbers. This will help you understand the different kinds of operators, what's being tested for when you use them, bitwise operations/shifts, and when they might be useful.
Logical Operators __
AND - used to combine two parts of a conditional statement and check to see if both parts are true OR - checks to see if at least one part of a statement is true before moving on XOR - checks to see if only one part of the two returns true NOT - only returns true if the condition is false
Ok, those are the names, but each one has an actual operator that corresponds.
AND - && OR(inclusive) - || XOR(exclusive) - ^ NOT - !
Here are a few examples to see how they work
(3==3 && 2 != 1) //true, 3 equals 3 and 2 doesn't equal 1 (7+1==5 || 3 == 4) //false,7+1 is 8, not 5, and 3 doesn't equal 4 (3*3==9 ^ 2!=2) //true, one condition(the first)is true (6.8 != 6.9) //true, 6.8 doesn't equal 6.9
Bitwise Operations __
Not only can those operators be used to check conditions, but can also figure calculations with numbers. This is where the bitwise operations come in. What's a bit? Well you should be familliar with binary to understand what's going on here. In a byte, there are 8 bits.
| bit | bit | bit | bit | bit | bit | bit | bit |
__________ byte ____________
Example: 3^5=6
So how's it calculated? Well the two numbers the operation is done on are converted to binary. You can get them to binary by going here http://www.yellowpipe.com/yis/tools/encrypter/index.php if you want. So you see that 3 converts to 00110011 and 5 converts to 00110101. Now you're xor'ing these, so you would take the first number from both to start. Remember how only one condition could be true for the whole statement to be true? 1 represents true and 0 represents false you take first number from 00110011 (0) and xor that with the first from 00110101(0) which would be 0. If you were and'ing, they would be the same to get 1. Or would return false. You continue over the whole byte until you have another binary number, which is the answer.
Bitwise shifts __
Shit left - <<
Shift right - >>
Assembly instructions:
Shift left: SHL Shift right: SHR
There are shift left's and shift right's of numbers. Again, it has to do with binary. [number] [way to shift] [ number to shift]
Example: 4<<1;
Get 4 to binary
00110100 then move each number 1 to the left, then convert the result to ascii and you have the answerthe first zero on the left would move to the second from the right and so on to get your answer of 8. Same with the right only you move right.
Why should you need to know these? These shifts and operations are done a lot in assembly and can help you in reverse engineering and analyzing code. They are apart of encryption and making one-way algorithms like MD5 and others. The avalanche effect in encryption is a result of bitwise shifts because the slightest different in bits that make up the byte can make totally different results. Hopefully someone can benefit from this, good luck.
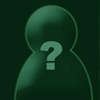
ghost 19 years ago
Very nice article. Didn't learn much but will be well written for those who don't know this already. Thumbs up!!
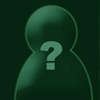
ghost 19 years ago
Decent article- The only thing I'd suggest is that articles on concepts like these generally need far more explanation of any large concepts you build on to be helpful to people who don't already know most of the information you present. Definitely a good article for now, but that's just a suggestion for next time.
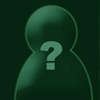