Quick start to python
Quick start to python
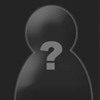
‘Python’® is a dynamic object-oriented programming language that can be used for many kinds of software development. It offers strong support for integration with other languages and tools, comes with extensive standard libraries, and can be learned in a few days. Many Python programmers report substantial productivity gains and feel the language encourages the development of higher quality, more maintainable code.’–python.org
This is all well and good, but I remember when I first started looking for a programming language and came across that description and just thought WTF???
So I’m here to explain a little more about python, and hopefully in terms those new to programming can understand.
I think the best way to start would be to show you a simple programme:
#!/usr/bin/python #hello world a=‘hello world!’ print a b=’’ while (b.find(‘exit’)==-1): [tab]b=raw_input(’press any key: ’)
this may look a little complex but here’s the breakdown:
This line simply states where the python is installed on a system. #!/usr/bin/python
The hash (or pound) symbol in this line means it is a comment and is to be ingored by the interpreter. #hello world
Here I am setting a varible notice how it has no special characteristics? unlike perl or php where a $ is needed. a=‘hello world!’
this line prints a (or hello world!) to the user. print a
before a varible can be used it must be set. b=’’
here is while clause: while (b.find(‘exit’)==-1): it checks varible for the word ‘exit’, a result of -1 means its not present where as if it were the result would be the charater at which the string starts.
[tab]b=raw_input(’enter string: ’) I have had to use [tab] to indicate an indentation as tey are not allowe din articles. this indicates that we are in a clause for example if or for and in this case while. raw_input(’enter string: ’) means take input form the keyboard. and promt with ’enter string: ’.
::notice how no end line temrinator exists.
That was just to show you how a simple script would look, python is a very simple language which is also very powerfull, most people can master it in a week from scratch. It’s usefullness comes in it’s ability to provide a simple solution to a complex problem and in a short space of time. It is also very portible, apart form running in Unix OSX and windows, I recenty discovered it could also run on nokia mobiles.
Below I have included a few structural examples.
if (a==‘true’): checks if a is eqaul to ‘true’ notice the ‘==’ while ‘=’ is used to set a varible.
a=‘password’ raw_input(a+’: ’) will take input fomr the keyboard and promt with ‘password :’ notice how strings are placed togetehr wiht the +.
This brings up another point to remember in python. if you want the string for of some thign you must use str(thing_here) or int(thing_here) for the integer version. for example: a=2 print ‘the number ‘+a+’ follows 1.’ [edit] print “the number %d follows 1.” % (a) [/edit]
would cause an error while: a=2 print ‘the number ‘+str(a)+’ follows 1.’ would not.
this is probly the major error with new python users along with the fact that its case sensitive, ie ‘a’!=‘A’ and functions must be all in lower case. ie raw_input() not Raw_input().
Python like a lot of programming languages utilises modules. so you dont have to or example make scripts from scratch, and would like to open a http page you could import the urllib (url library). here’s and example of code: #!/usr/bin/python import urllib a=urllib.urlopen(‘www.google.com’)# open google.com as html b=open(‘page.html’, ‘w’)#creats a file to ‘w’(write) to. b.write(a)#saves a (the html) to that file b.close()#closes the file.
here are a few operations to rmemebr: a==b #check a and b are equal a=b #set a and b equal a!=b #check a and b are unequal a*b #multiple a by b a**b #do a to the power of b a/b #do a divided by b, unless a decimal is used this will always consider only the integral part (bigest whole number) hint: 4./3 will work. a%b #will give the remainder when a is divided by b a+b #a add b a-b #a minus b
for files: ‘w’ #write to (over write) ‘r’ # read only ‘a’ # append to
in text: ‘[backslash]n’ #new line ‘[backslash]a’ # system beep ‘[backslash]b’ #backspace articles also strip backslashes lol.
Python is downlaod able from http://www.python.org/download/ ofcourse if you run unix or unix-like systems it come pre-instlled.
where you can also find much help with python. Also, the HBH(http://www.hellboundhackers.org/code.php) code bank contains much python code (sadly mostly by me) but can be useful.
Hopefully I havent just given you tonnes of pointless info, I have tried to create a short conise text on the subject.
Copyright: http://www.hbhzine.com by Wolfmankurd
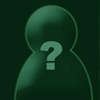
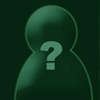
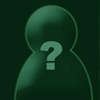
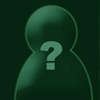
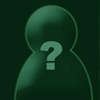
ghost 19 years ago
Nice article. I never got in to python, I hated the way that colons were used in loops, rather than the good old {}.
Anyway, nicely done wolfmankurd.
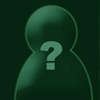
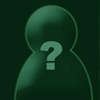
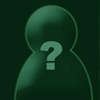
richohealey 18 years ago
print 'the number '+str(a)+' follows 1.'
oh yeah…. much better to show
print "the number %d follows 1." % (a)
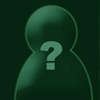