Perl for the noobish
Perl for the noobish
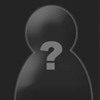
Introduction to PERL (Practical Extraction and Report Language) is a mid-level programming language which is used in command line and as cgi web server scripts. Its basically kind of like like C, Basic or Pascal.
Anyway like any other tutorial I'm going to show you how to make a simple hello world script, oh and by the way I should point out to run Perl scripts on your computer you need a Perl Interpreter. You can get one called ActivePerl from http://www.dzsoft.com/getperl.htm. There's also a program called Perl Editor which just helps write, edit and debug your scripts. To run your script on a web server, it too needs to have an interpreter. So just check to see if the web hoster your using/your web server has Perl installed. Usually if it has cgi your good to go. Ok now that we got that out of the way, on to your first script.
#!/usr/bin/perl
print "Content-type: text/html\n\n"; print "<Hello World!>\n";
You can see how in the first line we have #!/usr/bin/perl. This simply points to where Perl is installed and is needed in all perl scripts, this varies from system to system. NOTE the hash(#) in Perl is how you make comments in your code, except for the first line. Next print "Context-type: text/html\n is only needed if the output is going to a HTML page, otherwise its just a niusance in shell based scripts. Now the final line, print "Hello World!\n";. As before there is the print function, this is similar to C's printf which you actually can use in Perl, but for those who don't know how it works, whatever inside the quotes gets print onto the screen, but you have to note that if you use single quotes everything you write will be printed as is, meaning that any special characters, like new line (\n) wont be processed so will be displayed as normal. unlike when you use double quotes which process all special characters. Also worth noting is the semi-colon (;) which has to be placed after every statement in your code otherwise it wont run.
Next are scalar variables or just variables if that's how you want to call them. Scalar variables can contain numbers, text, or a string of text and declared the first time you use them unlike in C in which you have to declare the variable before using it and had to declare the right one to for single integer, a word, text string etc. and in Perl the way you use them is $scalarname. So onto an example:
$a = "Fatty-booma-lot"; print $a;
As you can see $a has been assigned the value Fatty-booma-lot and then it was printed, so the output would of simply been Fatty-booma-lot. As you can see printing vaiables can be done outside and inside of quotes, but this is not the case when assigning variables and text into the one, so what can you do? The answer is interpolation, which in simpler terms means putting a dot(.) between the two/three/whatever you want to join them together. An example of this is:
$a = 6; $b = 4; $c = "There are " . $a . " knuts in a total of " . $b . " sacks."; print $c
And now onto the last thing im going to discuss today, Array variables aka. Arrays. Arrays are almost the same as scalars except for the fact that they store a list of scalars and that start with a @ (eg. @color)
@food ("korn", "banana", "co2"); print @food; print $food[2];
As you can see we assigned three elements, korn, banana, co2 to the array @food and then we printed the array out and then we printed out the third element in the array. At first you might look at print $food[2] and think with and look at how I said three and think to yourself "guh?". The fact is that if you want to print a certain element in a array you have to print it as if it were a scalar with a $ and have to specify which one you want to print, which is why the square brackets are there and you might have noticed how I called it. In Perl indices start from 0 and go 1, 2 and so and so forth.
Well that is end of my introduction to Perl. ##Dragonista##
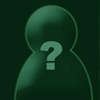
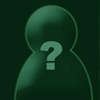
nanoymaster 18 years ago
I've never used perl, but after reading this I now know verry little about it.. thanks. The last paragraph is a little unclear, but none the less understanderbal….awsome article.
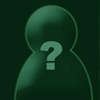
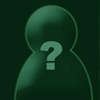
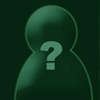
ghost 18 years ago
dont u have too include ""(or ' if u want it to print exactly what u type instead of incorperating things like vars and newline chars) when using the print function.Like.. print "$a";
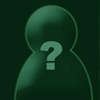
ghost 17 years ago
http://cyberforge.awardspace.com/index.php?page=basicperltutorial This site has a better version of my tut since i noticed that some of the information ive writen here is indeed incorrect
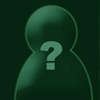
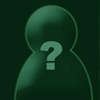
ghost 16 years ago
i know Perl stands for Practical Extraction and Repot Language, but why dont they make the "and" a part of it and call it pearl..?lol. Nice article for beginners, a little shallow you should stick to either shell or web scripting, but nice anyways.