The ShellSort procedure
The ShellSort procedure
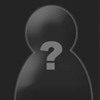
First of all I want to say that my English isn't that good so bare with me, ok? ;)
Now let's begin.
Sorting text… Most people will think this is quite ordinary, you write something and you click a button or call a procedure to sort a textbox/array or whatsoever.
No problem with the build-in procedures except that they sort text in a strange fashion. Let me illustrate with an example: if you have an array {1,10,2,33} then normally it will be sorted like this {1,10,2,33}. As you may notice this is all wrong.
Therefore a certain guy named Donald Shell invented an algorithm in 1959 to sort text in a 'correct' way. An implementation of this algorithm is the ShellSort Sub procedure. I will now give this procedure written in Visual Basic as this will be easy to understand for everyone. It can be compiled using the .NET or 2005 edition.
Sub ShellSort(ByRef sort() As String, ByVal numofelements As Short, ByVal order As Integer)
'The ShellSort procedure sorts the elements of the array sort() 'in descending(=1) or ascending(=2) order 'and returns this array to the calling procedure
Dim temp As String
Dim i, j, span As Short
span = numofelements \\ 2
Do While span > 0
For i = span To numofelements - 1
For j = (i - span + 1) To 1 Step -span
Select Case order
Case 1
\'1 means descending
If sort(j) <= sort(j + span) Then Exit For
Case 2
\'2 means ascending
If sort(j) >= sort(j + span) Then Exit For
End Select
temp = sort(j)
sort(j) = sort(j + span)
sort(j + span) = temp
Next j
Next i
span = span \\ 2
Loop
End Sub
I wasn't able to display this code with tabs and such although I did type it this way. Oh well, Visual Studio can structorize this code ;)
Please note how simple this procedure is, by changing <= into >= you simple reverse the sorting order (see the Select Case structure).
Now for the use. The variables itself tell the required input: ShellSort(<array of type string with the elements you want to sort>, <number of lines>, <ascending or descending>) => returns an array with the sorted elements.
This code can be used in combination of C# or C++ and of course VB. In theory with every .NET language ;)
This code is also avaible at the Code Bank (nicely structured ;), http://www.hellboundhackers.org/readcode.php?id=23). Some elements are imported form the book Microsoft Visual Basic 2005 Step by Step by Michael Halvorson.
I hope you all liked the article, it's my first one. Please post both negative as positive comment, we all learn from our mistakes ;)
- The_Cell
PS if someone could PM me how to structure code in articles ;) I know it's isn't very readable. Just take a look at the Code Bank.