Sockets in perl
Sockets in perl
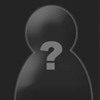
Sockets in perl.
You know you love it.
So, let's get down to business. Firstly, you might be saying to yourself 'Well hey, what are sockets and why do I need them?". The simpilfied definition is "The thing that applications use to communicate". As for the second part of the question, a great man once said "Everyone loves a socket".
Today, we're going to have a look at network sockets. These let you communicate over a network, and so they are pretty gosh darn bloody useful.
To demonstrate, I'm going to show you a port scanner written in perl and explain how it works.
use IO::Socket; print "Enter ip address to scan: "; my $ip = <STDIN>; chomp($ip); print "Enter starting port: "; my $startsock = <STDIN>; chomp($startsock); print "Enter finishing port: "; my $endsock = <STDIN>; chomp($endsock); die "Finishing socket must be greater than or equal to starting socket!" if$startsock > $endsock; my $i = $startsock; print "Scanning…\n"; for($i == $startsock; $i <= $endsock; $i++) { my $socket = new IO::Socket::INET(PeerAddr => $ip, PeerPort => $i, Proto => 'tcp',); if($socket) { print "Port $i is open\n"; close($socket); } }
-
use IO::Socket; #Imports the socket module we will need to use
-
print "Enter ip address to scan: "; ################################# my $ip = <STDIN>; # This section of code # chomp($ip); # gets the target IP address, # print "Enter starting port: "; # starting port and finishing # my $startsock = <STDIN>; # port, storing them in the # chomp($startsock); # variables $ip, $startsock # print "Enter finishing port: "; # and $endsock respectively. # my $endsock = <STDIN>; # # chomp($endsock); ################################# die "Finishing socket must be greater than or equal to starting socket!" if$startsock > $endsock; #Makes sure the starting port is smaller than the finishing one. Because otherwise it would be silly.
WHATS THAT? YOU DON'T KNOW WHAT A PORT IS?
OK, rather than explain it myself, I'll let google's "define:" search do it for me.
Because most network interfaces have only one or two physical ports (the means by which data comes into the computer from outside), you need to designate port numbers for different kinds of IP traffic. For example, port 80 is commonly used for HTTP traffic and port 21 is used for FTP.
Got that?
OK. More code.
-
for($i == $startsock; $i <= $endsock; $i++) #Run through the port range, assigning the value of each port as we pass through it to $i
-
my $socket = new IO::Socket::INET(PeerAddr => $ip, PeerPort => $i, Proto => 'tcp',); #Now, this creates the socket, and it takes three arguments - PeerAddr(The IP address to connect to) PeerPort(The port to connect to) And Proto(The protocol. Screw UDP, I'll write an article on that sometime).
So, now we have our socket, lets see if it connected.
- if($socket) #That was easy { print "Port $i is open\n"; #If it connected, then that port is open! So lets tell the user. close($socket); #Close the connection }
The execution looks like this
$perl pscan.pl Enter ip address to scan: 192.168.1.6 Enter starting port: 1 Enter finishing port: 600 Port 427 is open Port 548 is open
Oh, by the way - this only scans TCP and aint too stealthy. I'm just using it as a demonstration.
OK. It's 2:30 in the morning and thats all I can manage. Sorry if you can't understand my tired words. Next time - client and server applications in C.
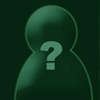
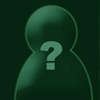
n3w7yp3 19 years ago
BobbyB: good code. BTW, you know that you can imbed the socket building routines in an if() loop? While iot may not seem like much, it cuts down on line size and does speed it up. GJ.
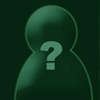
ghost 19 years ago
but n3w7yp3 code is sexy why would you want to reduce the amount of lines?! Nice Article BobbyB
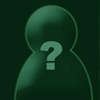
ghost 19 years ago
Very nice code, I love the IO::Socket module, such a wide variety of uses. Anyway, good article, one of the few i've seen around here lately.
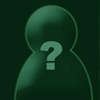