Date Functions (Javascript)
Date Functions (Javascript)
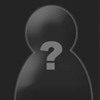
- Okay, I felt like writing a little tutorial on the Date() functions of JavaScript, inculding getYear(), getDay(), getMonth, and finally getFullYear().
- If you want, you can then do a document.write(??) to display the date, time, year, and day of the week (ex. Wed Nov 23 20:52:32 CST 2005).
- which would display the year, either (in most versions) as the four digit number ex. 2005, or (in Jscript v1.0) as the number minus 1900 ex. 105
- again, if you don’t want it to display a number, you’ll need to use an if, else if function
- and yet again, you need an if, else if function to display it as a word.
- and that should be everything you need to know about the getFullYear function.
Okay, I felt like writing a little tutorial on the Date() functions of JavaScript, inculding getYear(), getDay(), getMonth, and finally getFullYear().
First, the main Date() funtion: Okay, what all you really need to do to get the date is create a variable, name it something like date. Now the format you use is:
var Date = new Date()
If you want, you can then do a document.write(??) to display the date, time, year, and day of the week (ex. Wed Nov 23 20:52:32 CST 2005).
Second, the getYear() function: This is a strange one because in Jscript version 1.0, instead of simply displaying the date (ex. 2005), or the last two numbers of the date (ex. 05) it takes the date and subtracts 1900 from it. So the year 2000 is displayed as 100 and 1899 is -1. The variable format for this is below:
var Year = Date.getYear()
which would display the year, either (in most versions) as the four digit number ex. 2005, or (in Jscript v1.0) as the number minus 1900 ex. 105
Next, the getDay() function: This one is still a little strange, but not quite. Instead of displaying days, it assigns each day a number (see below), you can easily get around this by using a simple if, else if function. 0 - Sunday 1 - Monday 2 - Tuesday 3 - Wednesday 4 - Thursday 5 - Friday 6 - Saturday It’s variable format is:
var Day = Date.getDay()
again, if you don’t want it to display a number, you’ll need to use an if, else if function
Now, the getMonth() function This function, like the getDay() function, displays a number (see below). Again like the getDay() function, it can be fixed by a if, else if function. 0 - January 1 - Februry 2 - March 3 - April 4 - May 5 - June 6 - July 7 - August 8 - September 9 - October 10 - November 11 - December And it’s variable format is (like the others…):
var Month = Date.getMonth()
and yet again, you need an if, else if function to display it as a word.
And last, getFullYear(): This is a lot more reliable than a getYear() function, because it displays an absolute number such as 2005 instead of the number minus 1900. And yet again, the variable format:
var FullYear = Date.getFullYear()
and that should be everything you need to know about the getFullYear function.
Hope this helps someone :D