(C++)(Dev-C++) DLLs
(C++)(Dev-C++) DLLs
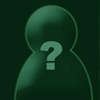
Hello, this is my first article. Well, it is my second, but I decided not to publish the other one. In this one I will try and describe how to make a DLL and use the functions you put into it, in your application, this is successfully compiled with Dev-C++, I don’t know if it will work with any others.
The first thing you need to do is make the DLL. To do this, make a new project and change it so that it compiles as a DLL (Project -> Project Options -> Win32 DLL -> ok). We will call it “My_DLL”, we will try and get a function of “void HelloWorld()” from it.
Add this to a blank file in the project, and save it as *.cpp
. Code .
/* Namespaces */ using namespace std;
/* define ‘DLL’ so we can use it to set functions to be exported */ #define DLL extern “C” __declspec(dllexport)
/* Make the function / DLL void HelloWorld() { / you will see the text ‘Hello, World!’ if the function successfully executes */
cout < < "\nHello, World!\n";
}
. End of Code .
what it does is creates a function in the DLL file (when you compile it) that you can access with a application.
The next thing you would want to do is create another C++ project, this time choose a console application, instead of DLL. Add the following code to a blank file in it, and save it as *.cpp.
. Code .
/* Namespaces */ using namespace std;
/* change the part in the last set of parenthesis to match whatever parameters the function your importing had: for instance if it was: HelloWorld(chartest, int a), you would use: typedef void (WINAPIFunc)(char*,int); the ‘Func’ part can be changed to whatever you like, and you also would change the beginning ‘void’ to whatever the function is, if it were a ‘int’ type, then you just change that to ‘int’ / typedef void (WINAPIFunc)();
/* this is what is going to be holding our function, I like to make it the same name as the function we are importing, but you can make it whatever you like. */ Func HelloWorld;
int main() { /* this is what we will use to load the DLL, and DLL will be our handle to that DLL. Remember earlier, I said that we would call the DLL ‘My_DLL’, I just added a .dll to the end, because when we compiled it, it made a .dll file. */ HINSTANCE DLL = LoadLibrary(“My_DLL.dll”);
/* check for error on loading the DLL */ if(DLL==NULL) { cout < < “We were unable to load: “ < < DLL < < “\n”; getch(); exit(0); }
/* this is where we set the function of 'HelloWorld()' from 'My_DLL.dll' into 'HelloWorld' */
HelloWorld=(Func)GetProcAddress((HMODULE)DLL, "HelloWorld");
/* check for error on getting the function */
if(HelloWorld==NULL)
{
cout < < "Unable to load function(s).\n";
FreeLibrary((HMODULE)DLL);
getch();
return 0;
}
/* Now that we have the DLL loaded, and the function set to HelloWorld, we can just use that as a function while the DLL is loaded. If it were a HelloWorld(int), then we would just add a int into it (we would also have to change the typedef to add a int into it) */
HelloWorld();
/* now that we are done with it, we will release. */
FreeLibrary((HMODULE)DLL);
}
. End of Code .
I hope this article helps out anyone who is curious about writing and getting functions from their own DLLs (you can also do the same thing to get functions from other DLLs.) I don't know how to withdraw classes and stuff from DLLs, only functions.
Well, I guess that's it for my article: happy coding.
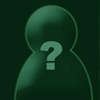
Adeil 19 years ago
I used conio.h because I don’t know any of the standard C++ headers (no .h) that has getch(), and I used windows.h because I don’t know of any header files that define the windows functions without the C header style. I don’t know if they have any updated headers in Visual, Borland, etc. I just have Dev-C++ hehe.
Also, I would have put the code into [code][/code] tags, but it didn’t seem to accept BBCode for the article. I would have changed the font color of all the stuff, so it looked like it does in a compiler’s rich edit text, but it seemed like too much extra work hehe.
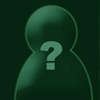
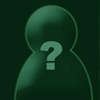