Portscanner in VB
Portscanner in VB
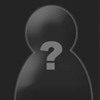
- Table of contents:
- 1' Portscanner, what is it?
- 2' Why would i want it?
- 3' How do i make one in vb?
- 4' How do i make it even better?
- 5' Disclaimer
######################################### | |
Basic Portscanner in Vb |
---|
by Anarcho-Hippie |
######################################### |
Hello again, this is the second tutorial in the basic Visual Basic programming series. The first one was a basic trojan, well now it is time for a basic portscanner :) If you understood the trojan you will understand this one too cause they both use the winsock control.
K let's begin:
Table of contents:
1' Portscanner, what is it? 2' Why would i want it? 3' How do i make one in vb? 4' How do i make it even better? 5' Disclaimer
1' Portscanner, what is it?
- A portscanner is a simple program that scans a computer for open ports. The one we are going to make is a tcp one. It uses the three way handshake to look for open ports. More on that in the disclaimer. You can compare it to a thief going on his "scout-tour", he goes to a house before he breaks in and checks every door and window to see if anything is open so he can enter later that night without forcing something.
2' Why would i want it?
-A portscanner can serve many purposes, you can check to see if you are infected with a certain trojan by checking your computer's open ports for specific trojan ports. Or it can be used to see if a victim has any vurnable ports open that can be used to gain further access to the machine.
3' How do i make one in vb?
-If you decided you want to make one for god knows wich reasons read on. First we need to input the winsock control to our project so create a new project and go to the components screen (ctrl+t) then check the box next to winsock control, press ok. After that it should be added to your toolbar on the left, just drag one to you project and voila.
So now after this create 2 textboxes, 2 buttons, a timer and one listbox. The first textbox is for the ip's, the second is for the starting port, the first button is for the starting, the second one is to stop the scanning. Then go to the timer and in the properties on the right change enabled to false and interval to 1000. If you like to fancy up your form with some warm colours and a fluffy border be my guest. K after that you go to the code menu where the actual stuff happens,
Private Sub Command1_Click() Winsock1.RemoteHost = Text1.Text ' The ip address or url to connect to Winsock1.RemotePort = Text2.Text ' The first port Winsock1.Connect Timer1.Enabled = True ' the rest of the ports. End Sub
Private Sub Timer1_Timer() Winsock1.Close ' closes the last connection Text2.Text = Int(Text2.Text) + 1 'increases the port by one Winsock1.RemoteHost = Text1.Text Winsock1.RemotePort = Text2.Text Winsock1.Connect End Sub
Now maybe you are wondering why i connected once an then enabled the timer to connect for the rest, well because of the line " Text2.Text = Int(Text2.Text) + 1 'increases the port by one " it doesn't scan the first port so you have to do it yourself . Now we have the scanning core of the portscanner, now we need something that says when it's open and when not.
Private Sub Winsock1_Connect() List1.AddItem "Port " & Winsock1.RemotePort & " is having a yard sale." End Sub
This is why winsock is easy to use, everything you need is build in a nice sub. So we just code the connect sub so that when it is called by the winsock scanning for ports it puts the port number in the list.
So that's basically it, but to make it complete we will code the stop button now,
Private Sub Command2_Click() Timer1.Enabled = False ' stops the scanning timer Winsock1.Close ' closes any open winsock connection that was still open. End Sub
That's it, you now have a simple working portscanner. Congratz.
4' How do i make it even better?
Well since it's a basic tutorial you will have a basic portscanner now. Things you could do to make it better:
- make a case statement in the winsock1.connect sub to recognize special ports.
- make it multithreading so several sockets work with eachother to scan faster.
- make it output the scan result to a textfile
- etc etc etc
5' Disclaimer
The usual stuff, i am not responsible for any stupid thing you might do with this, i only wrote this for educational purposes …. One thing you do have to know is that tcp works on the principe of the threeway handshake like i said before. That means that it works like this :> you send a request to a port, the port gets it and sends a "thank you" back. Now i know you have learned that a thank you is always a good thing but not in this case, it means you are discovered the minute you connect to a port cause they have to send a request back in order for it to work. So don't try anything stupid with this porstscanner. Use it on your own, or with permission. If you have questions or something, just get in touch and i'll help you out.