Portscanner in C++
Portscanner in C++
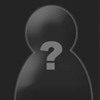
- Table of contents:
- 1° What is a portscanner?
- 2° Why would you need one?
- 3° How do i make one in C++?
- 4° What after that?
- 5° Disclaimer
################################# | | | Basic Portscanner in C++ | | By Anarcho-Hippie | | | #################################
Lo, this is the basic C++ series, my attempt to give something back to the community, so this tut we are going to make a basic portscanner in c++
Since it's a very basic tutorial the portscanner is going to be command line based. So here we go:
Table of contents:
1° What is a porstcanner? 2° Why would i need one? 3° How do i make one in C++? 4° What after that? 5° Disclaimer
1° What is a portscanner?
A portscanner is a tiny program that checks a computer for open ports and services running. There are several ones but we arn't going to go to deep into it now, so for now we are just going to build a tcp one. It operates on the three way handshake, more on that in the disclaimer. You can compare a portscanner to a thief going on his "scout-tour", he goes to a house before he breaks in and checks every door and window to see if anything is open so he can enter later that night without forcing something.
2° Why would you need one?
-A portscanner can server many purposes, you can check to see if you are infected with a certain trojan by checking your computer's open ports for specific trojan ports. Or it can be used to see if a victim has any vurnable ports open that can be used to gain further access to the machine.
3° How do i make one in C++?
K the first thing you have to do is add the right library for the winsock control. You do it like this:
Bloodshed DEV C++:> Make a new empty project, after that go to project -> Project Options -> Load Object file -> C:\Dev-Cpp\lib\libws2_32.a
Visual C++:> Open a new workspace, then go to projects-> settings (alt+F7) -> link and in the object/library modules add this to the end of the line: WS2_32.LIB
K now the linker will include the files we are going to use for the winsock.
Here are the includes and the namespace you need to have:
#include <iostream> //basic input ouput info #include <windows.h> // we need this simply for the setconsoletitle function #pragma comment(lib, "ws2_32.lib") //we need this so the compiler knows where to look for the winsock commands.
using namespace std;
//K now for the main sub, here is the code you will need.
int main()
{
//declaration of needed vars and everything
SOCKET mysocket;
int startport(0),endport(0);
char addres[MAX_PATH];
WSAData wsaData;
//end declaration
mysocket=WSAStartup(MAKEWORD(2,2) ,&wsaData);
if(mysocket==SOCKET_ERROR){
cout<<"Socket could not be started\n";
return 0;
}
cout<<"Socket started\n";
/* K before we go any further i'm going to give some explanation on the code we just put in, SOCKET mysocket => We need to declare a socket that we are going to use for the rest of the program
WSAData wsaData => This is a structure that contains network info but that's for more advanced stuff so for know just leave it as it is.
mysocket=WSAStartup(MAKEWORD(2,2) ,&wsaData) => This is where we startup the socketservice by using the WSAStartup function. The MAKEWORD(2,2) macro is there to input wich version of winsock you are going to use, you can change it if you want to use an earlier version if you need it. The &wsaData is just for the part where the socket get's his info from.
if(mysocket==SOCKET_ERROR){ cout<<"Socket could not be started\n"; return 0; } => The good thing about the winsock is the fact that every error provides a SOCKET_ERROR wich makes it easy to check for errors.
K so now we initialized the winsock, started it up and checked if it was running. Now we need to start up the socket and adjust the settings. */ mysocket=socket(AF_INET, SOCK_STREAM,0); SetConsoleTitle("My First Portscanner"); cout<<"################\n"; cout<<"| PortScanner |\n"; cout<<"|Written in C++|\n"; cout<<"| By |\n"; cout<<"|Anarcho-Hippie|\n"; cout<<"################\n"; cout<<"\n"; cout<<"\n"; cout<<"\n"; cout<<"input ip addres here: "; cin>>addres; cout<<"\n"; cout<<"The Starting Port: "; cin>>startport; cout<<"\n"; cout<<"The Ending Port: "; cin>>endport; cout<<"\n";
/* K again here is the explanation:
mysocket=socket(AF_INET, SOCK_STREAM,0) => Here we start the actual socket and set it to use the inet family(AF_INET) and make it a tcp socket (sock_stream), the null value is there because we don't need it if we use the inet protocol but the function needs 3 parameters.
SetConsoleTitle("My First Portscanner") => This just sets the title of the window. */
endport++; cout<<"###########################################################################\n"; do { loop: SOCKADDR_IN server; server.sin_port=htons(startport);// Socket port server.sin_family=AF_INET; // wich family it's going to use server.sin_addr.s_addr=inet_addr(addres); // wich ip address to use
/* K, here is the settings part, SOCKADDR_IN server => This is the structure that stores all the needed data, the port, the address, and the ipaddressess of the computer. server.sin_port=htons(startport) => the htons() function converts the integer stored in startport to network numbers, htons stands for host to network service. K if you are still following, we are almost done :) */
if(connect(mysocket,(SOCKADDR*)(&server),sizeof(server))==SOCKET_ERROR) { cout<<startport<<" is not open.\n"; startport++; if(startport>=endport) { cout<<"No ports open\n\a"; cin.get(); closesocket(mysocket); //closes socket wsaCleanup(mysocket); // cleans up the socket wsaStartup<-> wsaCleanup break; } goto loop; } else{ cout<<"Port "<<startport<<" is open!\n"; closesocket(mysocket); mysocket=socket(AF_INET,SOCK_STREAM,0); startport++; if(startport>=endport) { cin.get(); break; } goto loop; } }while (startport != endport); cout<<"###########################################################################\n"; cin.get(); closesocket(mysocket); wsaCleanup(mysocket); }
/* K the last part for the socket connect(mysocket,(SOCKADDR*)(&server),sizeof(server)) => This connects thrue the socket we started on the address and socket stored in server (structure SOCKADDR_IN). That's it, you now have a very basic portscanner in c++ */
4° What after that?
After this you should look up something more about sockets in c++. Don't forget you find alot of good info on msdn, one of microsoft's usefull products. Experiment with the code and expand on it, add a switch statement so it recognizes common ports etc, make it do stealth scanning, alot of possibilities.
5° Disclaimer
I am not responsible for any illegal things you might do with this information. Don't forget that this portscanner is a tcp one, thus is works like the three way handshake. You send a request to a port, the port get's the request and sends a reset request back. So don't try anything stupid with it cause you will be noticable the minute you check a port. Other then that, have fun with it :) Don't fuck up cause you will never get any job that has something to do with computers after that, i know that is the dream of many, but dreams come with sacrifices so be carefull what you do or do not do. Any help, comments, suggestions, hatemail, etc you know how to find me.
Cheers Anarcho-Hippie.