Welcome to HBH! If you have tried to register and didn't get a verification email, please using the following link to resend the verification email.
Administrator Panel Finder - Scans for over 1000 directories - Python Code Bank
Administrator Panel Finder - Scans for over 1000 directories
This is an administrator panel finder I have created. This is the first thing I have made in Python (been doing it for about two weeks) so any feedback is appreciated. The code isn't commented but should be easy enough to understand.
#!/usr/bin/python
import urllib
#Load in directories/files
def load_files(ext_type):
lis = []
extension = ''
directory = ['adm/', 'admin/', 'administrator/', 'account/', 'authentication/', 'area/', 'secure/', 'user/', 'login/', ' test/', 'acc/', 'users/', 'custom/', 'access/', 'sitemap/', 'adminarea/', 'admin_area/', 'adminLogin/', 'siteadmin/', 'sysadmin/', 'sys/', 'system/']
files = ['access', 'account', 'adminaccount', 'admin_account', 'admin', 'admin1', 'authenticate', 'secure', 'adminLogin', 'admin_panel', 'administrator', 'administratorLogin', 'control', 'controlpanel', 'cp', 'cpanel', 'index', 'loginindex', 'panel', 'phpmyadmin', 'user', 'user_panel', 'wp-login']
if ext_type == 'PHP':
extension = '.php'
elif ext_type == 'ASP':
extension = '.asp'
for direct in directory:
lis.append(direct)
for value in files:
value_ext = value + extension
value_html = value + '.html'
temp = direct + value_ext
lis.append(temp)
temp = direct + value_html
lis.append(temp)
if value_ext not in lis:
lis.append(value_ext)
if value_html not in lis:
lis.append(value_html)
return lis
#Access the website
def find_panel(url, page_404, page_text, ext_type):
found = 0
i = 0
lis = load_files(ext_type)
url_temp = ''
test_items= ['login', 'username', 'password', 'admin', 'administrator', 'secure']
try:
urllib.urlopen(url)
except:
print url + " has failed to open!\n\n\n"
print "\nProcessing..."
for directory in lis:
i += 1
if i == len(lis):
print "Done!"
url_temp = url + directory
try:
page = urllib.urlopen(url_temp)
except:
print "Cannot read the webpage!"
text = str(page.read())
code = page.getcode()
if code == 200:
if url != page.geturl() and page_404 != page.geturl() and page_text not in text:
for item in test_items:
if item in text:
print item
print "Found: " + url_temp
found = 1
print "Trying to find more..."
continue
if found == 0:
print "\nSorry, " + str(len(lis)) + " directories tested and administrator panel could not be found!"
#User 'interface'
def get_details():
print """
Administrator Panel Finder v1.0 - Created by w0rms
- w0rms@securityaddicts.org -
Once the script is running, you will be prompted to enter a url trailed
by a backslash (/). Next you will be prompted for a custom-404 page. It
is becoming more common for websites to implement custom 404-pages
(e.g. www.example.com/404.php) - This can lead to false positives when
trying to find the administrator panel. Check for a custom 404-page. If
there is no custom 404-page, press return when prompted for one. Otherwise,
add the whole URL of the custom 404-page when prompted. Next you will asked
for text that is displayed when a 404 page or error page is found. This is
set to 404 by default. The last thing you will be prompted for is the file
extention of the webpages. Currently, v1 only supports php and asp pages.\n"""
print "||Administrator.Panel.Finder - Coded by w0rms||"
url = raw_input("Please enter the URL trailed by a backslash: ")
page_404 = raw_input("Please enter a custom 404-page: ")
page_text = raw_input("Please enter some text that is shown when psge 404(404 by default): ")
ext_type = raw_input("Please select filetype: PHP for .php, ASP = .asp: ")
test = url.endswith('/')
if test == False:
url = url + '/'
if page_text == '':
page_text = '404'
ext_type = ext_type.upper()
if ext_type != 'PHP'and ext_type != 'ASP':
print "You have entered the extension type incorrectly. Restarting..."
main()
find_panel(url, page_404, page_text, ext_type)
#Define main()
def main():
get_details()
main()
Comments
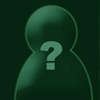