Welcome to HBH! If you had an account on hellboundhacker.org you will need to reset your password using the Lost Password system before you will be able to login.
HBH Module - Python Code Bank
HBH Module
Module for interacting with HBH.
Use it to aid in timed challenges, or pulling forum updates from HBH.
Notice in the GET/POST methods that it returns a string instead of an handle. I prefer a string, so I put it in the method, that way I wouldn't need to call it in main(); this may speed it up a smidgen.
And if your going to use this with the timed challenges, lambda is your friend.
import urllib, urllib2, cookielib
class HBH:
"""HBH Class used to interact with HBH"""
def __init__(self,user,pas):
self.user_name = user
self.user_pass = pas
self.login_data = { 'user_name':self.user_name,
'user_pass':self.user_pass,
'login':'Login' }
def Opener(self,ref):
"""Creats an opener to store cookies,
and keep a referer to the site
Added user-agent to spoof browser"""
self.refrence = ref
cj = cookielib.CookieJar()
self.opener = urllib2.build_opener(urllib2.HTTPCookieProcessor(cj))
self.opener.addheaders.append(('User-agent', 'Mozilla/4.0'))
self.opener.addheaders.append(('Referer',ref))
return self.opener
def GET(self,opnr,url):
"""HBH GET method, notice to data option"""
get_req = opnr.open(url)
return get_req.read()
def POST(self,opnr,url,data):
"""data is a dictinary type like login_data"""
enData = urllib.urlencode(data)
get_req = opnr.open(url,enData)
return get_req.read()
def main():
#Example
me = HBH('techb','This_is_not_my_pass')
url_open = me.Opener('http://www.hellboundhackers.org/index.php')
request = me.POST(url_open,'http://www.hellboundhackers.org/index.php',me.login_data)
f = open('LogInTest.html','w')
f.write(request)
if __name__ == "__main__":
main()
Comments
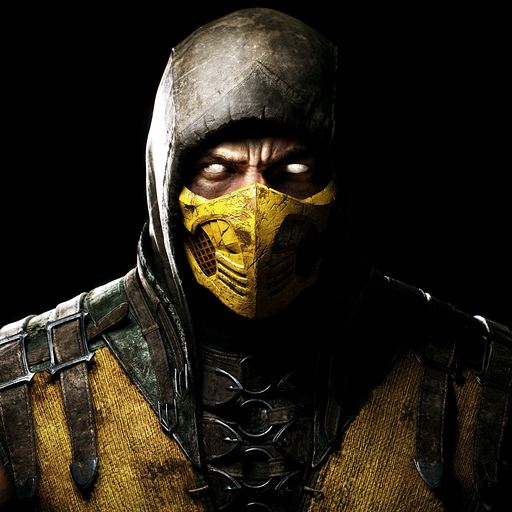